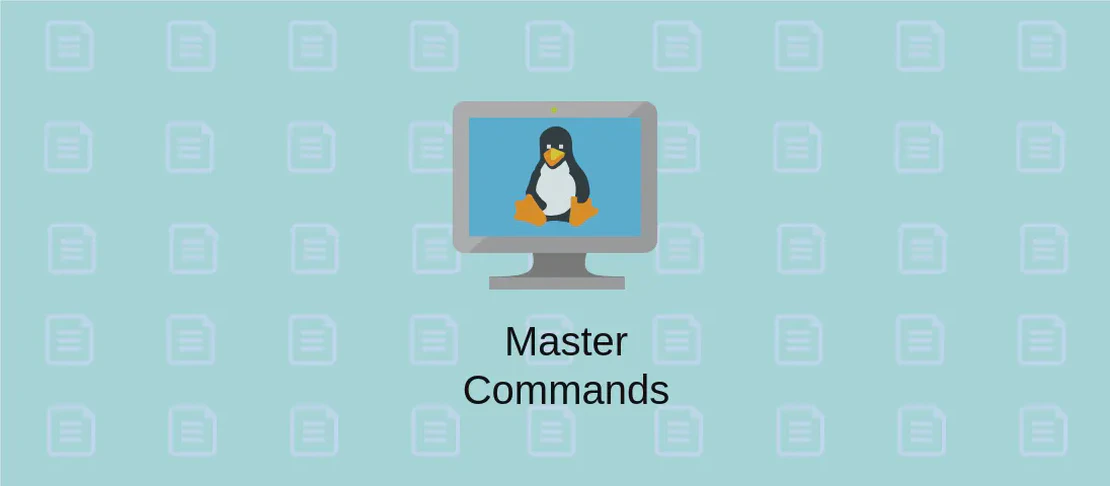
Mastering Web Crawling with Scrapy (with examples)
Scrapy is a powerful and versatile open-source web crawling framework designed to extract data from websites. It provides multiple tools and features that make web scraping efficient and manageable. Scrapy’s architecture is built to handle large-scale scraping, allowing users to create projects and spiders to automate the data extraction process from various web sources.
Use case 1: Create a Project
Code:
scrapy startproject project_name
Motivation: Creating a project in Scrapy is the foundational step when initiating a new scraping endeavor. It establishes an organized framework to manage all the components of a web scraper, such as spiders, pipelines, items, and settings. This step ensures that the project is set up with a folder structure that Scrapy understands and can operate within.
Explanation:
scrapy
: The command-line tool used to execute Scrapy commands.startproject
: A sub-command that initializes a new Scrapy project.project_name
: The desired name for your new project. This will be the name of the directory created to hold all project-related files.
Example Output:
Upon executing, you will see a message indicating that a new project structure has been created, including directories for spiders, pipelines, and configuration files.
Use case 2: Create a Spider
Code:
scrapy genspider spider_name website_domain
Motivation: Spiders are the core of the Scrapy framework, responsible for defining the logic for extracting, processing, and storing data from specific web pages. Creating a spider allows you to set specific rules for web scraping on a given domain or set of URLs, making data collection precise and structured.
Explanation:
scrapy
: Executes the Scrapy tool.genspider
: Generates a new spider template in your project for scraping.spider_name
: The name you wish to assign to your new spider, which will help in identifying different spiders when multiple are used.website_domain
: The domain of the website from which you plan to scrape data. This helps in scoping the crawl, so it only targets specified domains.
Example Output:
On successful creation, Scrapy will generate a spider file within the ‘spiders’ directory, pre-populated with example code for starting your crawl process.
Use case 3: Edit Spider
Code:
scrapy edit spider_name
Motivation: Editing the spider allows you to customize and improve the scraping logic initially set during its creation. By modifying the spider code, you can refine what data to extract, implement parsing logic, configure settings, or add error handling, ensuring that the spider effectively captures the intended data.
Explanation:
scrapy
: The command-line interface for Scrapy.edit
: This sub-command opens the specified spider in the default editor for editing.spider_name
: The identifier of the spider you wish to edit, targeting the specific file in your project.
Example Output:
If configured correctly, this command would open the specified spider file in a text editor, allowing you to make necessary modifications.
Use case 4: Run Spider
Code:
scrapy crawl spider_name
Motivation: Running your spider is the execution step where all your logic and configuration are put into practice to collect the desired data. This step triggers the actual web crawling process, sending HTTP requests, and processing responses according to your defined rules, thus extracting and storing the data as required.
Explanation:
scrapy
: Executes the Scrapy tool.crawl
: A sub-command to initiate the crawling process using the specified spider.spider_name
: The specific spider to run, which executes the predefined crawl logic.
Example Output:
The terminal will display log messages indicating the process of sending requests, receiving responses, and capturing the data. Successfully extracted data is stored as per the configuration.
Use case 5: Fetch a Webpage
Code:
scrapy fetch url
Motivation: Fetching a webpage as Scrapy sees it allows developers to understand how Scrapy interacts with web pages, particularly useful for debugging and ensuring correct page structure retrieval. This is critical for confirming that the crawler can access and interpret web pages as expected.
Explanation:
scrapy
: Invokes the Scrapy command-line tool.fetch
: A command to retrieve the specified webpage.url
: The web address of the page you wish to fetch, providing a sample of the response from that URL as Scrapy would see it.
Example Output:
Running this command prints the HTML source of the specified page to the console, showcasing the exact content fetched by Scrapy.
Use case 6: Open a Webpage in Default Browser
Code:
scrapy view url
Motivation: Opening a webpage in your default browser as seen by Scrapy gives a real-world view of how the scraper perceives the page. This is particularly useful for visually confirming that the page loads correctly without JavaScript, which Scrapy does not support by default.
Explanation:
scrapy
: Executes the Scrapy command-line utility.view
: Opens the specified URL in a browser session.url
: The URL of the web page to be viewed in the browser.
Example Output:
The command will fetch the URL and open it in your default browser, disabling JavaScript to ensure consistency with what Scrapy captures during its crawl.
Use case 7: Open Scrapy Shell
Code:
scrapy shell url
Motivation: Opening a Scrapy shell provides an interactive Python programming environment to test data extraction scripts. This is invaluable for iterating and debugging your HTML parsing code before deploying it in the spider, ensuring each component works correctly.
Explanation:
scrapy
: Calls the Scrapy command-line tool.shell
: Opens the interactive shell for web scraping tasks.url
: The web page to open in the shell, allowing for live testing of extraction logic on that page.
Example Output:
Initiating this command brings up a Python shell prompt where you can execute scripts, test data selectors, and evaluate the output live from the given URL.
Conclusion:
Scrapy is an indispensable tool for web developers and data scientists working with web data. Its rich ecosystem of tools and commands for initiating projects, coding spiders, extracting data, and troubleshooting are vital for seamless web data extraction processes. By mastering these commands and understanding their uses, users can effectively harness the power of Scrapy to fulfill various web scraping tasks.