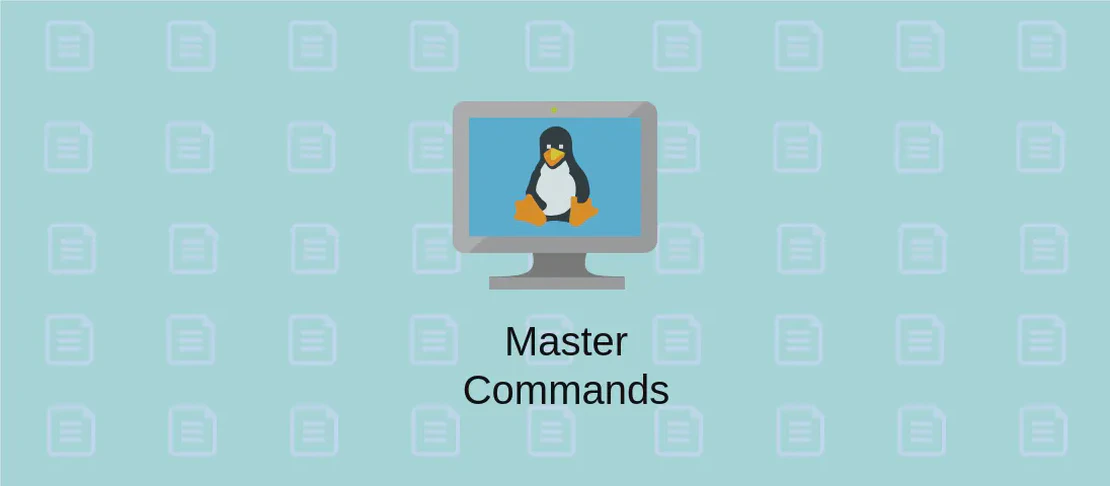
How to use the command 'sd' (with examples)
The sd
command is a fast and user-friendly tool for performing substitution operations, making it a suitable alternative to traditional utilities like sed
. It operates with an intuitive syntax, leveraging Rust’s robust regular expression library to ensure efficiency and ease of use. The command is particularly helpful for developers and system administrators who frequently require batch editing of text within files or standard input streams.
Use case 1: Trim some whitespace using a regular expression
Code:
echo 'lorem ipsum 23 ' | sd '\s+$' ''
Motivation:
In many situations, especially when dealing with user input or processing data, trailing whitespace can be problematic. This example demonstrates how to efficiently remove unwanted trailing spaces from a string, ensuring cleaner data output and preventing potential storage or formatting issues.
Explanation:
echo 'lorem ipsum 23 '
generates a string with excess trailing whitespace.|
pipes this string tosd
.sd '\s+$' ''
:\s+$
: This regular expression pattern matches any whitespace character(s) (\s
) occurring at the end of a line ($
).''
: Represents what the matched whitespace should be replaced with. In this case, it is replaced with nothing, effectively trimming the whitespace.
Example Output:
lorem ipsum 23
Use case 2: Replace words using capture groups
Code:
echo 'cargo +nightly watch' | sd '(\w+)\s+\+(\w+)\s+(\w+)' 'cmd: $1, channel: $2, subcmd: $3'
Motivation:
Capture groups become instrumental when processing complex patterns and needing structured data out of unstructured text. This example illustrates replacing parts of a command string with more descriptive labels, making it easier to parse and understand programmatically.
Explanation:
echo 'cargo +nightly watch'
produces a string illustrating a Rust toolchain command.|
passes this string tosd
for substitution.sd '(\w+)\s+\+(\w+)\s+(\w+)' 'cmd: $1, channel: $2, subcmd: $3'
:(\w+)
: Captures a sequence of word characters. Used here to capture command segments.\s+\+(\w+)
: Matches one or more spaces followed by a+
and another word segment. Represents the toolchain flag.(\w+)
: Continues capturing trailing command segments.'cmd: $1, channel: $2, subcmd: $3'
: Replaces the entire matched string with a structured format using back-references$1
,$2
, and$3
, representing corresponding capture groups.
Example Output:
cmd: cargo, channel: nightly, subcmd: watch
Use case 3: Find and replace in a specific file
Code:
sd -p 'window.fetch' 'fetch' path/to/file.js
Motivation:
When upgrading libraries or moving to simplified code structures, developers often need to find and replace specific function calls or method references across their codebase. This example demonstrates how to execute such a replacement within a specific file, streamlining the refactoring process.
Explanation:
sd -p 'window.fetch' 'fetch' path/to/file.js
:-p
: Stands for “preview.” It shows the results of the find and replace operations without making changes to the file, allowing for verification.'window.fetch'
: The string or pattern to find within the file.'fetch'
: The string with which to replace the found pattern.path/to/file.js
: The specific file to search and replace in.
Example Output (preview format):
Shows lines with substitutions without altering the actual file content.
...
15 | - window.fetch(url)
15 | + fetch(url)
...
Use case 4: Find and replace in all files in the current project
Code:
sd 'from "react"' 'from "preact"' "$(find . -type f)"
Motivation:
Performing mass replacements across an entire project is a frequent requirement, especially during a tech stack migration or when optimizing library usage. This example demonstrates efficiently replacing imports across multiple files in a project directory.
Explanation:
sd 'from "react"' 'from "preact"' "$(find . -type f)"
:'from "react"'
: The portion of code, generally the import statement, to find across the files.'from "preact"'
: The string to use as a replacement, reflecting a library change."$(find . -type f)"
: Utilizes Unix’sfind
command to list all files (-type f
) within the current directory (.
), passing these files tosd
for processing.
Example Output:
Output is typically the replaced lines in respective files, showcasing changes made across the project.
...
src/index.js:
4 | - import { useState } from "react";
4 | + import { useState } from "preact";
...
components/Navbar.js:
2 | - import React from "react";
2 | + import React from "preact";
...
Conclusion:
The sd
command simplifies text transformation tasks with an accessible syntax and powerful pattern-matching capabilities. These use cases illustrate how sd
addresses common issues related to trailing whitespace, structured command parsing, targeted file refactoring, and large-scale project updates, making it an invaluable tool for efficient text manipulations.