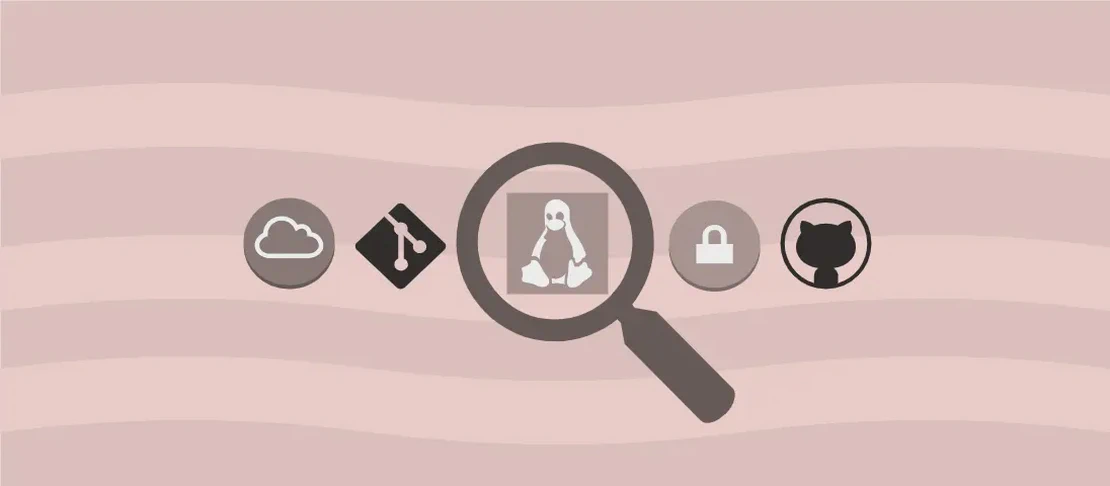
Understanding the 'sed' Command (with examples)
- Linux
- December 17, 2024
The sed
command, short for Stream Editor, is a powerful Unix utility used to parse and transform text in a scriptable manner. It’s built into most Unix-like systems and provides a plethora of functions to search, find, replace, insert, and delete text in a way that’s both flexible and efficient. It’s particularly useful for processing and analyzing large datasets directly from the command line, especially when working within shell scripts. Compared to other text processing tools, it stands out for its simplicity and script-driven transformation capabilities.
Use case 1: Replace All Occurrences of ‘apple’ with ‘mango’ and Print the Result
Code:
command | sed 's/apple/mango/g'
Motivation:
You may often need to substitute specific words in text data, perhaps due to a change in terminology or the need to standardize the text. This command serves as a quick solution for replacing all instances of the word ‘apple’ with ‘mango’ across all input lines, efficiently simplifying any text alterations needed.
Explanation:
command
: Any command that outputs text to the terminal can be piped intosed
.sed
: Invokes the stream editor.'s/apple/mango/g'
: Thes
is the substitute command,apple
is the pattern to search for,mango
is the replacement text. Theg
at the end stands for “global,” which means replace all occurrences in each line.
Example Output:
If the input text is:
apple tree and apple pie
The output will be:
mango tree and mango pie
Use case 2: Replace All Occurrences of ‘apple’ with ‘APPLE’ Using Extended Regex and Print the Result
Code:
command | sed -E 's/(apple)/\U\1/g'
Motivation:
When dealing with data that requires case standardization, this example illustrates how you can convert specific terms to uppercase while using regular expressions (regex). It’s particularly useful in data cleaning tasks in text-based data processing.
Explanation:
command
: Any preceding command piping text intosed
.sed -E
: The-E
flag enables extended regex support, allowing for more complex regex operations.'s/(apple)/\U\1/g'
: Again,s
is for substitution. The pattern(apple)
capturesapple
in a group (necessary for back-referencing), and\U\1
converts the first captured group to uppercase.g
replaces all occurrences in each line.
Example Output:
If the input text is:
apple pie and apple tart
The output will be:
APPLE pie and APPLE tart
Use case 3: Replace All Occurrences of ‘apple’ with ‘mango’ in a Specific File
Code:
sed -i 's/apple/mango/g' path/to/file
Motivation:
Editing files in place without creating temporary copies is crucial for automation scripts and system administration tasks. This command updates the file directly by replacing all instances of ‘apple’ with ‘mango’, streamlining updates to text files.
Explanation:
sed -i
:-i
option allows in-place editing, modifying the file directly.'s/apple/mango/g'
: Thes
operation for substitution, changing every ‘apple’ to ‘mango’ in the file.path/to/file
: Path to the file you want to edit.
Example Output:
Assuming a file with:
apple cider and apple wine
After execution, the file content changes to:
mango cider and mango wine
Use case 4: Execute a Specific Script File and Print the Result
Code:
command | sed -f path/to/script.sed
Motivation:
Complex text processing often benefits from scripting; this allows predefined commands to be executed to automate processes. This method enables the reusability of sed
commands by storing them in a script file.
Explanation:
command
: A command whose output is manipulated bysed
.sed -f path/to/script.sed
: The-f
flag specifies the script file containingsed
commands to be executed.
Example Output:
If script.sed
contains:
s/apple/mango/g
And the input is:
apple juice and pear
The output will be:
mango juice and pear
Use case 5: Print Just the First Line of Input
Code:
command | sed -n '1p'
Motivation:
In scenarios where only the initial entry or header of a dataset is required, this command functions to extract exactly that from a larger body of text seamlessly and efficiently.
Explanation:
command
: Produces text input forsed
.sed -n
: The-n
flag suppresses automatic printing, makingsed
only output data explicitly specified for printing.'1p'
: Tellssed
to print (p
) only the first line.
Example Output:
If the input text is:
First line
Second line
The output will be:
First line
Use case 6: Delete the First Line of a File
Code:
sed -i 1d path/to/file
Motivation:
Removing header lines for data processing tasks is common, especially when assembling large datasets where headers are unnecessary. This command automates the deletion process directly within a file.
Explanation:
sed -i
: In-place editing flag.1d
: Command tod
elete the first (1
) line.path/to/file
: Specifies file to edit.
Example Output:
File before executing:
First line
Second line
After execution, the file becomes:
Second line
Use case 7: Insert a New Line at the First Line of a File
Code:
sed -i '1i\your new line text\' path/to/file
Motivation:
Prepending important information or metadata to documents is essential for structuring data files consistently, especially in reporting or documenting environments. This command inserts new starting lines directly.
Explanation:
sed -i
: Enables in-place file modification.'1i\your new line text\'
:1i
for insert at line 1, followed by the text to be inserted.path/to/file
: File to be modified.
Example Output:
Original file content:
Second line
Third line
After execution, it becomes:
your new line text
Second line
Third line
Conclusion:
The sed
command proves to be an invaluable tool within the Unix/Linux ecosystem, offering a wide range of possibilities for text manipulation directly from the command line. From replacing words globally to modifying lines within files, its versatility and efficiency make it a staple in both automation scripts and one-off command-line tasks. The ability to script its operations further elevates its utility, providing a handy solution for complex text processing needs.