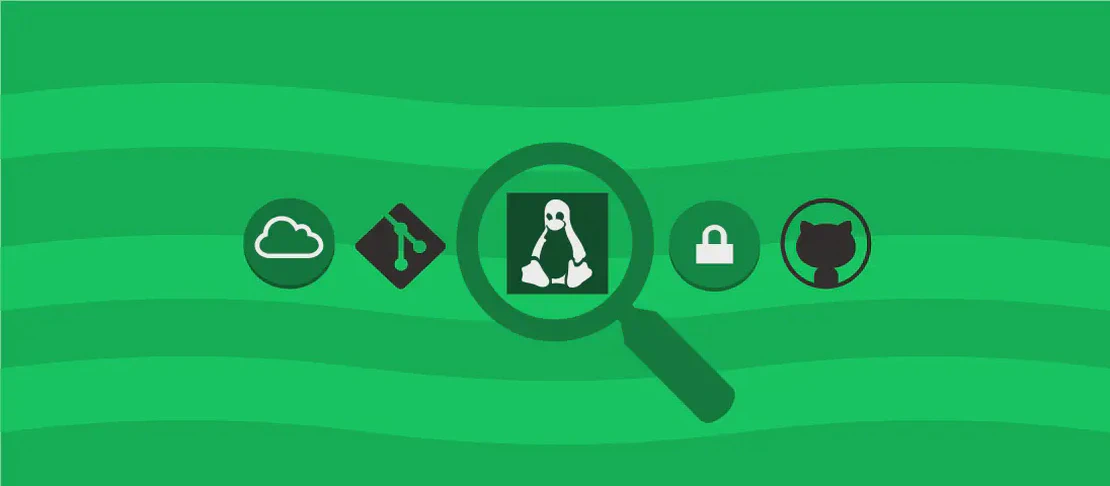
How to Use the Command 'sed' (with Examples)
- Openbsd
- December 17, 2024
The sed
command, short for Stream Editor, is a powerful Unix utility for parsing and transforming text. It allows users to perform text editing in a scriptable manner, making it essential for automating text processing tasks. With its ability to interpret regular expressions, sed
can easily perform text substitution, deletion, and more across multiple files or input streams, all with a few succinct commands. This article explores several practical use cases of sed
, providing insight into its versatility and effectiveness.
Replace All ‘apple’ Occurrences with ‘mango’ and Print the Result to stdout
Code:
command | sed 's/apple/mango/g'
Motivation:
In many text-processing scenarios, you might need to replace occurrences of a specific word across a text stream. For instance, imagine you’re working with a data set or log file where the term “apple” needs to be replaced with “mango” across large batches of data. Using sed
for this task is efficient and eliminates the need for manual edits.
Explanation:
command
: Represents the input stream from which text will be read. It could be a pipeline output or any valid command generating text.sed
: Invokes the stream editor.'s/apple/mango/g'
: Thes
denotes the substitution command.apple
is the pattern to search for,mango
is the replacement text, andg
is a flag indicating that the replacement should occur globally across each line.
Example Output:
If the input stream contains:
I have an apple and an apple tree.
The output will be:
I have a mango and a mango tree.
Execute a Specific Script [f]ile and Print the Result to stdout
Code:
command | sed -f path/to/script.sed
Motivation:
This use case is ideal for repeated, complex text manipulations. By writing transformation rules in a script, you can reuse and maintain them better than in inline commands, especially when dealing with complex sequences of edits or maintaining readability in batch operations.
Explanation:
command
: Provides the input stream for processing.sed
: Calls the stream editor.-f path/to/script.sed
: The-f
option specifies the path to a script file containingsed
commands.sed
will execute each command in the file sequentially.
Example Output:
Assuming path/to/script.sed
has the following contents:
s/apple/mango/g
s/tree/bush/g
And the input is:
I have an apple and an apple tree.
The output will be:
I have a mango and a mango bush.
Delay Opening Each File Until a Command Containing the Related w
Function or Flag is Applied to a Line of Input
Code:
command | sed -fa path/to/script.sed
Motivation:
In scenarios where you need to apply edits conditionally based on the occurrence of a command involving the w
function, this use case becomes significant. It optimizes resource usage by only opening files when necessary, particularly useful when handling operations across large files.
Explanation:
command
: Input data stream.sed
: Activates the stream editor.-fa path/to/script.sed
: Combines-f
to specify a file of commands witha
, a mode that delays file opening until necessary.
Example Output:
Given path/to/script.sed
includes:
s/apple/mango/w changes.txt
With input:
I have an apple.
This command sequence will open changes.txt
for writing only when the pattern matches and produce the output:
I have a mango.
The changes.txt
will contain:
I have an apple.
Replace All ‘apple’ Occurrences with ‘APPLE’ Using Extended Regex and Print the Result to stdout
Code:
command | sed -E 's/(apple)/\U\1/g'
Motivation:
There are times when you want to format your text output with more advanced regex (using groups) and convert text into uppercase. This example showcases sed
’s capacity to work with extended regular expressions, which allow for more complex pattern matching.
Explanation:
command
: Input stream from which text will be edited.sed
: Invokes the sed utility.-E
: Enables extended regular expressions, providing more powerful regex operations.'s/(apple)/\U\1/g'
: The regex captures “apple” and replaces it with its uppercase equivalent.\U\1
converts the captured group (the entire text) to uppercase.
Example Output:
If the input provided is:
apple trees are apple-laden.
The output will be:
APPLE trees are APPLE-laden.
Print Just the First Line to stdout
Code:
command | sed -n '1p'
Motivation:
When handling large files, sometimes you just need to extract the first line, say, for headers or quick previewing of a file’s content. sed
can easily perform this task efficiently.
Explanation:
command
: Supplies the input stream.sed
: Calls the stream editor.-n
: Suppresses automatic printing, allowing manual control over what gets printed.'1p'
: Tellssed
to print only the first line of input.
Example Output:
Assuming this is the input:
First line of the file.
Second line of the file.
The output will be:
First line of the file.
Replace All ‘apple’ Occurrences with ‘mango’ in a Specific File and Overwrite the Original File In-Place
Code:
sed -i 's/apple/mango/g' path/to/file
Motivation:
This use case is perfect for scenarios where you need to perform in-place editing, avoiding the hassle of creating a temporary file to hold updates. It’s commonly used in scripts for batch processing files across directories.
Explanation:
sed
: Activates the stream editor.-i
: The in-place editing flag tellssed
to overwrite the file directly with the changes.'s/apple/mango/g'
: Performs a global substitution of “apple” with “mango”.path/to/file
: Specifies the file to be edited.
Example Output:
If path/to/file
contains:
An apple a day keeps the doctor away.
After running the command, the file will be updated to:
A mango a day keeps the doctor away.
Conclusion
These examples highlight the versatility of sed
for a wide range of text processing tasks. Whether you’re making simple substitutions, applying sophisticated regex transformations, or automating routine edits, sed
proves to be an indispensable tool for programmers, sysadmins, and anyone dealing with text data.