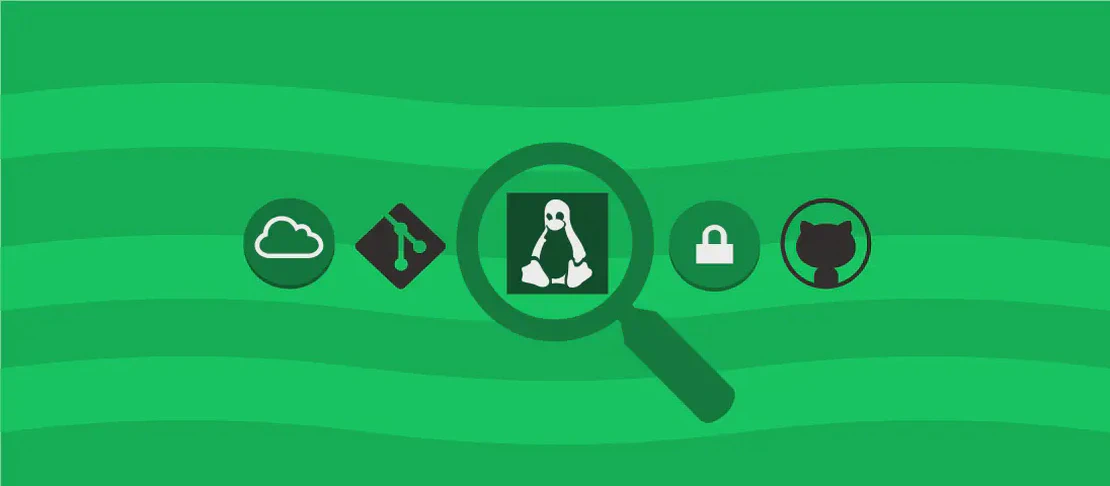
How to Use the Command 'sed' (with Examples)
- Osx
- December 17, 2024
sed
, short for “stream editor”, is a powerful utility in Unix and Linux used for parsing and transforming text. It is widely employed in shell scripts for non-interactive editing tasks like substitution, searching, and text manipulation. Unlike text editors such as vi
or nano
, sed
works through stream processing, allowing the modification of data in a scriptable manner, which is exceedingly beneficial for automated tasks and processing large datasets. The ability to implement complex transformations with concise expressions makes sed
a versatile tool in the Unix command suite.
Use Case 1: Replacing All Occurrences of ‘apple’ with ‘mango’ Using Basic Regex
Code:
command | sed 's/apple/mango/g'
Motivation:
In text processing and data manipulation, replacing words is a common requirement. Suppose you’re processing a dataset containing fruit names and need to standardize the name ‘apple’ to ‘mango’ across all entries. This command provides a streamlined, efficient solution to perform global substitutions across large input data.
Explanation:
command
: Represents any command whose output you wish to process.sed
: Invokes the sed utility.'s/apple/mango/g'
: The substitution operation. The pattern's/old/new/g'
specifies replacing occurrences of ‘old’ with ’new’. Theg
at the end signifies a global replacement, meaning all occurrences in each line will be substituted.
Example Output:
Suppose the output of command
is:
apple pie
apple cider
apple tart
After running the above sed
command, the resulting output would be:
mango pie
mango cider
mango tart
Use Case 2: Executing a Script File and Printing the Result
Code:
command | sed -f path/to/script_file.sed
Motivation:
Leveraging pre-written sed
scripts enables reusability and consistency in text processing tasks. Suppose you have a complex sequence of edits saved in a script file that you wish to apply to multiple data streams. By utilizing this method, you reduce errors, streamline operations, and maximize efficiency.
Explanation:
command
: Represents the input stream to be processed.sed
: Invokes the sed utility.-f path/to/script_file.sed
: The-f
option specifies the use of a script file, which contains multiplesed
commands to be executed in sequence.
Example Output:
If script_file.sed
contains:
s/apple/mango/g
s/pie/tart/g
With input:
apple pie
apple tart
The output would be:
mango tart
mango tart
Use Case 3: Replacing All Occurrences of ‘apple’ with ‘APPLE’ Using Extended Regex
Code:
command | sed -E 's/(apple)/\U\1/g'
Motivation:
Sometimes, text transformation requires using extended regular expressions for more sophisticated pattern matching and case transformation, such as converting text to uppercase. This example caters to those needs by allowing case conversion of text matches, which is particularly useful when normalizing data for comparison or display.
Explanation:
command
: Represents the input data stream.sed
: Invokes the sed utility.-E
: Enables extended regular expressions.'s/(apple)/\U\1/g'
: The substitution pattern;(apple)
captures the matched text, and\U\1
converts it to uppercase. Theg
ensures that every match within each line is converted.
Example Output:
For input:
apple orchard
apple cider
The resulting transformed output would be:
APPLE orchard
APPLE cider
Use Case 4: Printing Only the First Line of Input
Code:
command | sed -n '1p'
Motivation:
In many scenarios, such as log analysis, you might only need the first line of output for quick verification or summary purposes. This example demonstrates how to extract only the first line from a set of input data efficiently without processing the rest.
Explanation:
command
: Represents your input command or file.sed
: Calls the sed utility.-n
: Suppresses automatic printing of lines.'1p'
: Specifically instructs sed to print only the first line.
Example Output:
For input:
First line
Second line
Third line
The output after applying the command:
First line
Use Case 5: Replacing ‘apple’ with ‘mango’ in a File with Backup
Code:
sed -i bak 's/apple/mango/g' path/to/file
Motivation:
Maintaining data integrity is crucial when editing files in place. This use case is ideal when you need to perform substitutions within a file but also want to keep a backup of the original content before changes, ensuring that data can be restored if needed.
Explanation:
sed
: Calls the sed utility.-i bak
: Edits the file in place and creates a backup with the.bak
extension.'s/apple/mango/g'
: The substitution command replaces all occurrences of ‘apple’ with ‘mango’.path/to/file
: Specifies the file to be edited.
Example Output:
Given file
content:
apple pie
apple juice
Before running the command, the file contents would be saved as file.bak
. After executing the command, file
would contain:
mango pie
mango juice
Conclusion:
sed
is an adaptable and robust tool pertinent to text transformation and processing tasks. Each use case demonstrates its flexibility in performing operations like substitution, executing script files, selective printing, and in-place editing with backup features, all of which are essential for efficient text management in Unix-like environments.