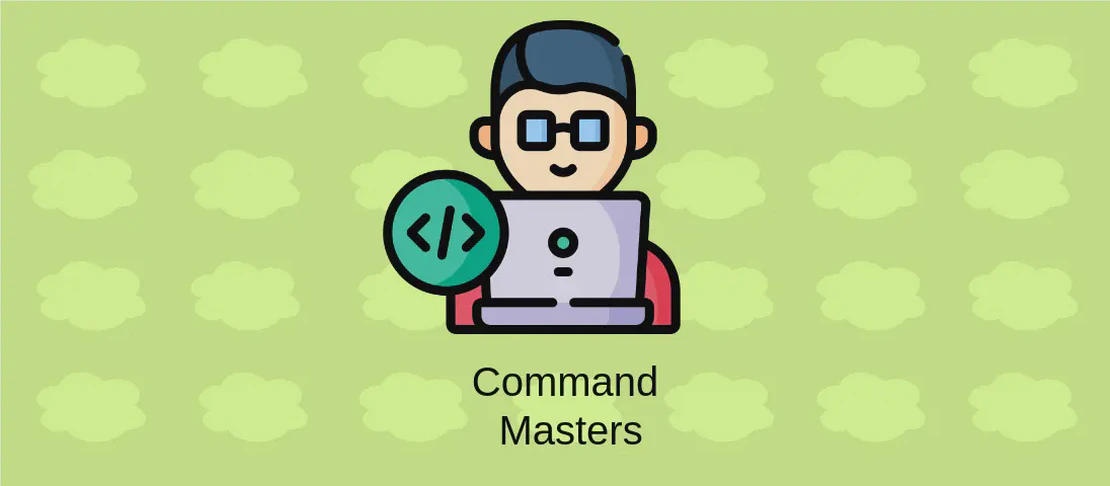
How to Use the Command 'select' in Bash (with examples)
The select
command in Bash is a built-in construct that assists users in creating simple menus from which options can be easily chosen in scripts. It is particularly useful when presenting users with a selection from a list of values, whether these values are static or dynamically derived from previous commands. This command enhances script interactivity by allowing users to make choices effortlessly.
Create a Menu from Individual Words
Code:
select word in apple orange pear banana; do echo $word; done
Motivation:
Creating a menu from a predefined list of individual words is particularly useful when you have a static list of options or choices that the user needs to select from. In many interactive shell scripts, users may need to input a value from a limited set of choices, and select
provides a straightforward way to handle this.
Explanation:
select
: Initiates the selection menu.word
: A placeholder variable that will hold the user’s choice from the menu.in apple orange pear banana
: Defines the options available in the menu; for each iteration,select
displays these words as choices.do echo $word; done
: Loops to echo the chosen option. When the user selects an option, it assigns the value toword
and displays it withecho
.
Example Output:
1) apple
2) orange
3) pear
4) banana
#?
After entering a number corresponding to a fruit, say “1”:
apple
Create a Menu from the Output of Another Command
Code:
select line in $(command); do echo $line; done
Motivation:
Generating menus from the output of another command is a powerful way to handle dynamic data within your shell scripts. If the set of options is not known beforehand and must be generated or fetched at runtime, leveraging the output of commands like ls
, grep
, or any other command is fundamental.
Explanation:
select
: Starts the menu creation.line
: Variable to capture and hold each option during the selection.in $(command)
: Executes whatever command is specified, generating a list that theselect
menu will present.do echo $line; done
: Once an option is selected, it is echoed back to the user, showcasing the selected item.
Example Output:
Suppose command
results in a list of files: file1.txt file2.txt
1) file1.txt
2) file2.txt
#?
Selecting “2” yields:
file2.txt
Specify the Prompt String for select
and Create a Menu for Picking a File or Folder from the Current Directory
Code:
PS3="Select a file: "; select file in *; do echo $file; done
Motivation:
Customizing the prompt string helps in making the menu more intuitive and user-friendly. By changing the prompt, you guide the user to input the expected action. This is particularly helpful when you want users to select files or directories from the existing working directory, ensuring a smoother user interaction.
Explanation:
PS3="Select a file: "
: Sets a custom prompt message displayed each time input is awaited.select file in *
: Lists all files and directories in the current working directory as choices using wildcard expansion.do echo $file; done
: Displays the selected file or directory when the user makes a choice.
Example Output:
Given a directory with doc1.txt
, doc2.txt
, and folder
:
1) doc1.txt
2) doc2.txt
3) folder
Select a file:
Choosing “3” results in:
folder
Create a Menu from a Bash Array
Code:
fruits=(apple orange pear banana); select word in ${fruits[@]}; do echo $word; done
Motivation:
Using arrays with select
is ideal when you want to maintain a collection of items in your script without having to hard-code or separately define them. Arrays allow you to manage and store data efficiently, especially when the dataset is large or might change.
Explanation:
fruits=(apple orange pear banana)
: Defines an array namedfruits
containing the options to be listed.select word in ${fruits[@]}
: Uses array expansion to present all elements of the array as menu choices.do echo $word; done
: Outputs the user’s selection based on their choice from the menu.
Example Output:
1) apple
2) orange
3) pear
4) banana
#?
Upon selecting “4”:
banana
Conclusion:
The select
command is a versatile tool in Bash scripting, with use cases ranging from simple hard-coded menus to dynamic option lists generated during runtime. Understanding its implementation in various contexts helps in creating robust and user-friendly shell scripts. With these examples, scripts can be created to interactively guide users through diverse selections and commands, enhancing the overall scripting experience.