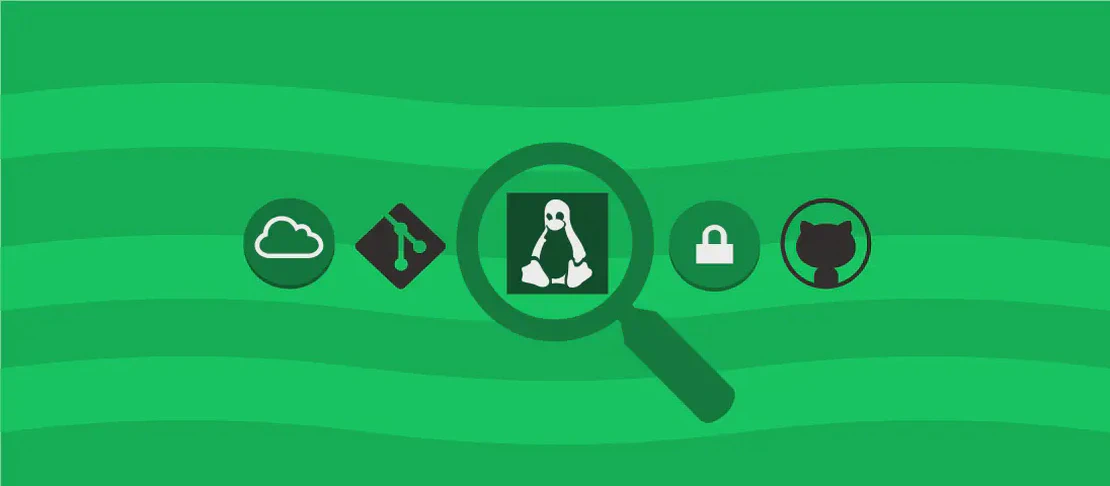
How to use the command `select` (with examples)
- Linux
- December 25, 2023
The select
command is a bash built-in construct that can be used to create menus in a shell script. It allows the user to choose an option from a list of choices.
Use case 1: Create a menu out of individual words
Code:
select word in apple orange pear banana; do
echo $word;
done
Motivation: This use case is useful when you want to present the user with a simple menu where they can choose a word from a predefined list. For example, if you are writing a script for a recipe book, you can use this menu to allow the user to select a fruit ingredient.
Explanation: The select
command creates a menu based on the words provided after the in
keyword. In this case, the menu will contain the words “apple”, “orange”, “pear”, and “banana”. The selected option will be stored in the variable word
. The do
and done
keywords are used to define the actions to be performed for each selected option. In this example, the selected option is echoed.
Example output:
1) apple
2) orange
3) pear
4) banana
#? 2
orange
Use case 2: Create a menu for picking a file or folder from the current directory
Code:
select file in *; do
echo $file;
done
Motivation: This use case is useful when you want to allow the user to select a file or folder from the current directory. This can be helpful for tasks like file management or selecting input/output files for a script.
Explanation: The select
command creates a menu based on the files/folders in the current directory, represented by *
. The selected option will be stored in the variable file
. The do
and done
keywords are used to define the actions to be performed for each selected option. In this example, the selected option (file or folder name) is echoed.
Example output:
1) file1.txt
2) folder1
3) script.sh
#? 3
script.sh
Use case 3: Create a menu from a Bash array
Code:
fruits=(apple orange pear banana);
select word in "${fruits[@]}"; do
echo $word;
done
Motivation: This use case is useful when you have a predefined list of choices stored in a Bash array. For example, if you are writing a script for a grocery store, you can use this menu to allow the user to select a fruit from a list of available options.
Explanation: The select
command creates a menu based on the elements of the fruits
array. The selected option will be stored in the variable word
. The do
and done
keywords are used to define the actions to be performed for each selected option. In this example, the selected option (fruit) is echoed.
Example output:
1) apple
2) orange
3) pear
4) banana
#? 1
apple
Conclusion:
The select
command is a powerful tool in bash scripting that allows you to create interactive menus. It can be used to simplify user interactions and enhance the usability of your scripts. By understanding the different use cases and examples provided above, you can leverage the select
command to create dynamic menus in your own scripts.