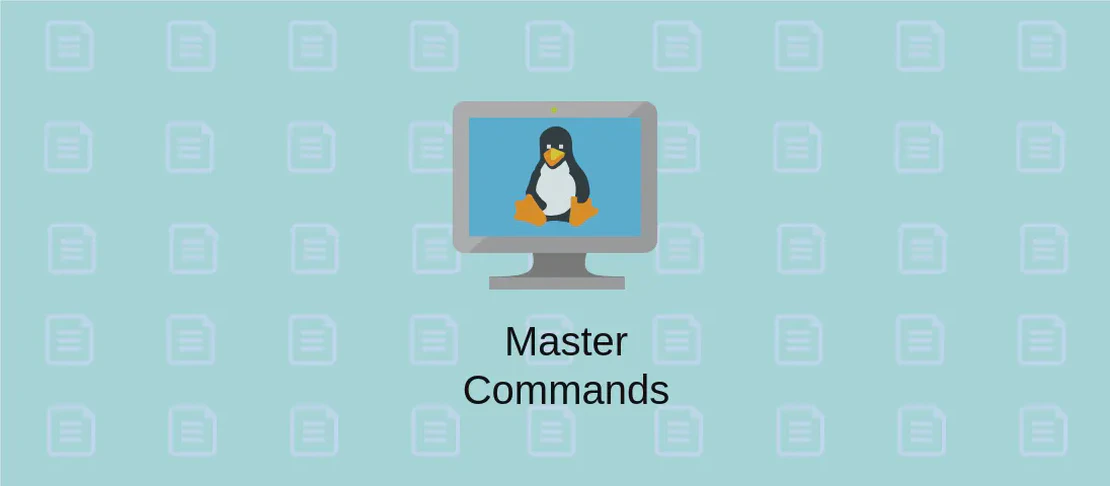
How to Use the Command 'Select-String' (with examples)
The Select-String
cmdlet in PowerShell is a powerful tool designed to search text and strings in files. It’s often compared to the grep
tool in UNIX and findstr.exe
in Windows, given its ability to search through large amounts of data efficiently. Whether you are trying to filter logs, search for specific patterns in text files, or need to conduct a string search over a batch of files, Select-String
offers a versatile solution.
Use case 1: Search for a pattern within a file
Code:
Select-String -Path "path\to\file" -Pattern 'search_pattern'
Motivation:
In any situation where you have a large file and you need to extract specific lines containing a particular string or pattern, this command becomes incredibly useful. For instance, when dealing with log files containing thousands of lines, identifying entries related to a specific event can be done swiftly through this command.
Explanation:
-Path
: Specifies the file path where PowerShell should search.path\to\file
refers to the location of your intended file.-Pattern
: Instructs PowerShell to look for lines matching the'search_pattern'
. This pattern represents the string or regular expression you want to search for.
Example Output:
Suppose your file contains the following lines:
Error: The system cannot find the file specified.
Warning: Low disk space.
Info: Task completed successfully.
Using the command with a pattern of "Error"
, the output would be:
Error: The system cannot find the file specified.
Use case 2: Search for an exact string (disables regular expressions)
Code:
Select-String -SimpleMatch "exact_string" path\to\file
Motivation:
Sometimes using regular expressions isn’t necessary, or it can be too complicated for a simple search. When you want to search for an exact match without regular expression interpretation, using -SimpleMatch
can be more efficient and straightforward, ensuring no special characters are misinterpreted.
Explanation:
-SimpleMatch
: Forces the search to treat the pattern as a simple string match, bypassing any regular expression functionality."exact_string"
: Represents the exact text you need to find.path\to\file
: The file path where the command will search for the exact string.
Example Output:
If your file includes:
This is a test string.
Another test line.
Exactly matching string.
Searching for "Exactly matching string."
would return:
Exactly matching string.
Use case 3: Search for a pattern in all .ext
files in the current directory
Code:
Select-String -Path "*.ext" -Pattern 'search_pattern'
Motivation:
When working in a directory that contains multiple files of the same type, and you need to find a common pattern, this command solution allows for a simultaneous scan of all those files. It saves time over manually searching through each file one by one.
Explanation:
-Path "*.ext"
: Commands PowerShell to perform the search in all files with the.ext
extension within the current directory.-Pattern 'search_pattern'
: Directs PowerShell to search for the specified pattern within those files.
Example Output:
Imagine you have files file1.ext
and file2.ext
that include:
file1.ext:
Sample text with search_pattern.
Another line.
file2.ext:
Different file line with nothing.
The command would output:
file1.ext: Sample text with search_pattern.
Use case 4: Capture the specified number of lines before and after the line that matches the pattern
Code:
Select-String --Context 2,3 "search_pattern" path\to\file
Motivation:
This is particularly useful in analyzing logs and scripts where context is crucial. Capturing surrounding lines helps in understanding the circumstances around the occurrence of the pattern, rather than evaluating the match in isolation, which can provide more insights into the issue or event.
Explanation:
--Context 2,3
: Captures 2 lines before and 3 lines after the matching line, providing context."search_pattern"
: The string or pattern you are investigating.path\to\file
: The specific file you need to search through.
Example Output:
Given a file:
First line.
Second line.
Third line with search_pattern.
Fourth line.
Fifth line.
Sixth line.
Seventh line.
The above command will return:
First line.
Second line.
Third line with search_pattern.
Fourth line.
Fifth line.
Sixth line.
Use case 5: Search stdin
for lines that do not match a pattern
Code:
Get-Content path\to\file | Select-String --NotMatch "search_pattern"
Motivation:
In many instances, it is equally beneficial to filter out lines that do not match a pattern, especially when cleansing data or removing irrelevant information. This can be instrumental in refining data for subsequent processing steps.
Explanation:
Get-Content path\to\file
: Reads the file’s content line-by-line.|
: A pipeline operator that takes the output of one command and uses it as input for another.Select-String --NotMatch "search_pattern"
: Filters the lines, removing those that match the “search_pattern”.
Example Output:
For a file:
Keep this line.
Remove this search_pattern line.
Another line to keep.
Line with search_pattern again.
The output after executing the command would be:
Keep this line.
Another line to keep.
Conclusion:
The Select-String cmdlet in PowerShell proves to be a versatile and robust tool for searching text within files and streams. From simple string matching to contextual searches, Select-String
offers various options for tailoring your searches to meet specific objectives. Whether you are analyzing logs, cleansing data, or sifting through numerous files for specific patterns, harnessing the functionalities of Select-String
ensures that you can efficiently and accurately process textual data.