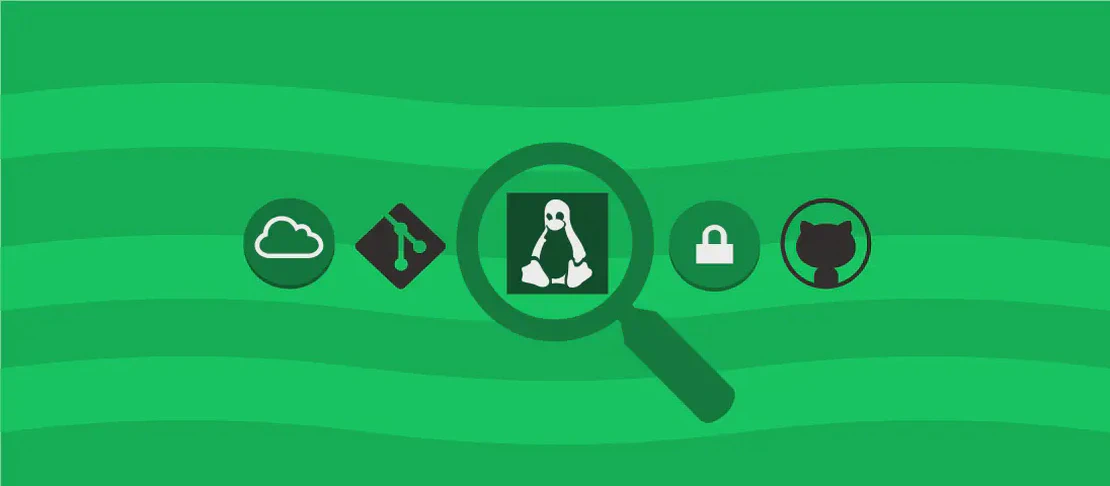
How to use the command 'serve' (with examples)
The serve
command is a popular tool designed primarily for static file serving and directory listing. Developed by Vercel, it provides a lightweight and efficient solution for anyone needing to serve static files quickly. This tool is versatile, supporting features like configuring CORS, using custom configurations, and enabling HTTPS, which makes it great for developers who want to quickly test websites and web applications.
Use case 1: Start an HTTP server listening on the default port to serve the current directory
Code:
serve
Motivation:
This use case is perfect for developers who need to quickly preview static websites without needing a full-fledged web server setup. It serves the contents of the current directory at http://localhost:3000
by default, allowing easy access to your browser.
Explanation:
By running serve
with no additional arguments, you initiate a simple static file server. The command automatically picks up files in the current directory and serves them over HTTP. The default port used is 3000, making it straightforward to remember and access.
Example output:
When you run this command, a message similar to the following appears:
Serving!
- Local: http://localhost:3000
Use case 2: Start an HTTP server on a specific [p]ort to serve a specific directory
Code:
serve -p 8080 path/to/directory
Motivation:
This use case is beneficial when you need to serve files from a particular directory other than the current one or have multiple services and need to avoid port conflicts. It offers flexibility in setting up your local environment the way you need.
Explanation:
The -p
flag allows you to specify a particular port on which the server should listen. Replacing port
with 8080
configures the server to run on port 8080 instead of the default. The path/to/directory
specifies which directory to serve, allowing you to serve files from a location different than your current directory.
Example output:
Upon executing this command, you’ll see:
Serving!
- Local: http://localhost:8080
Use case 3: Start an HTTP server with CORS enabled by including the Access-Control-Allow-Origin: *
header in all responses
Code:
serve --cors
Motivation:
Cross-Origin Resource Sharing (CORS) can be a hurdle when developing front-end applications that need to access resources from different domains. Using this command, developers can simulate a CORS-enabled server, which is crucial for testing how web applications interact with cross-origin resources.
Explanation:
The --cors
flag modifies the server response to include the Access-Control-Allow-Origin: *
header. This change means that any web page requesting resources from this server will not face the security restrictions usually imposed by browsers related to CORS.
Example output:
Once initiated, the server confirms its operational status and enables CORS support. Output resembles:
Serving with CORS enabled!
- Local: http://localhost:3000
Use case 4: Start an HTTP server on the default port rewriting all not-found requests to the index.html
file
Code:
serve --single
Motivation:
Single Page Applications (SPAs) often face issues where direct access to routes results in 404 errors because the server tries to find a matching file for the path. Using --single
, you ensure that all requests are rerouted to index.html
, which is critical for the correct functioning of SPAs.
Explanation:
The --single
flag ensures a fallback to the index.html
file for all non-static requests, allowing routers like React Router to manage navigation based on client-side routing logic.
Example output:
The server starts and outputs a message indicating its running status:
Serving and redirecting 404s to index.html!
- Local: http://localhost:3000
Use case 5: Start an HTTPS server on the default port using the specified certificate
Code:
serve --ssl-cert path/to/cert.pem --ssl-key path/to/key.pem
Motivation:
Enabling HTTPS is essential for mimicking production environments during local development. It allows secure connections, which is crucial for features like Service Workers or when interacting with APIs that enforce HTTPS.
Explanation:
The --ssl-cert
flag specifies the SSL certificate to use, while --ssl-key
specifies the private key for that certificate. These arguments are necessary to run the server over HTTPS on the default port (3000).
Example output:
When the server runs successfully, you see an output:
Serving over HTTPS!
- Local: https://localhost:3000
Use case 6: Start an HTTP server on the default port using a specific configuration file
Code:
serve --config path/to/serve.json
Motivation:
Using a configuration file helps maintain consistency across environments and allows defining custom rules and settings without altering the command line execution each time.
Explanation:
The --config
argument accepts a path to a JSON configuration file specifying settings for the serve
command, like default port, base directory, and other custom behaviors.
Example output:
After initiating, you’ll observe a confirmation of the server start and often detailed log messages depending on the JSON configuration:
Serving with custom config!
- Local: http://localhost:3000
Use case 7: Display help
Code:
serve --help
Motivation:
Consulting the help menu is an excellent way to familiarize yourself with all the capabilities and options offered by serve
. It can guide users through a detailed breakdown of available commands and usage patterns.
Explanation:
The --help
flag instructs the serve
command to output a help menu, which provides descriptions of available flags, options, and general usage advice.
Example output:
The console displays an extensive listing of instructions and options, supportive of both novice and experienced users:
Usage: serve [options] <path>
...
Conclusion:
The serve
command by Vercel is a versatile tool that offers developers a hassle-free way to serve static files and simulate production environments locally. From basic directory serving to HTTPS setups, serve
facilitates multiple web development scenarios with ease, making it an indispensable utility in a developer’s toolkit.