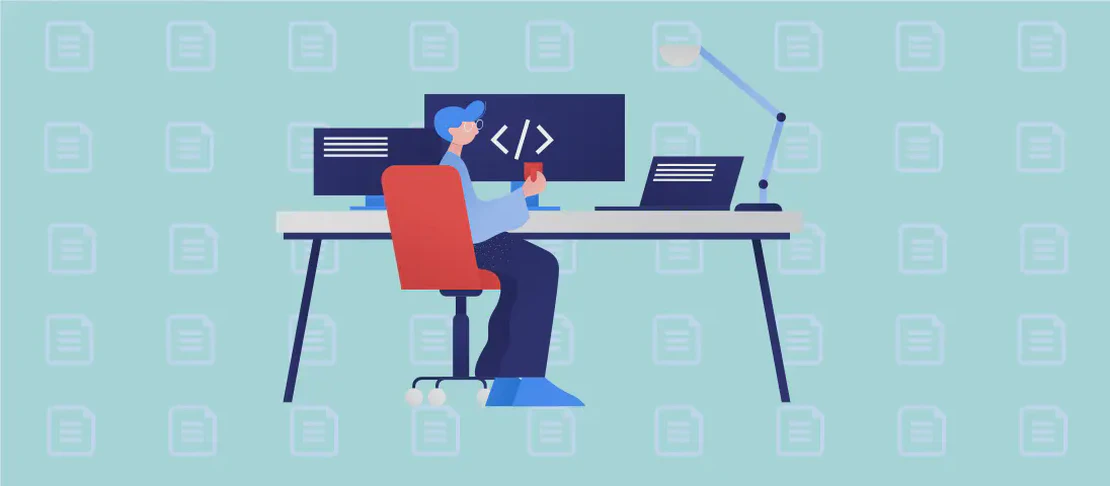
Managing File Security Descriptors with PowerShell's Set-Acl Command (with examples)
The Set-Acl
cmdlet in PowerShell is a versatile tool designed to manage security descriptors of various system objects, including files and registry keys. It’s a part of the Microsoft PowerShell Security module and allows administrators to alter access control lists (ACLs) to manage user permissions efficiently. Altering these security descriptors is crucial in a multi-user environment to maintain data integrity and system security by defining who has access to specific files or registry entries.
Use Case 1: Copy a Security Descriptor from One File to Another
Code:
$OriginAcl = Get-Acl -Path path\to\file; Set-Acl -Path path\to\file -AclObject $OriginAcl
Motivation:
Imagine a scenario where you’ve meticulously set up the permissions for a particular file, ensuring only specified users have read or write access. Now, suppose you need another file, likely a new version or a related document, to have the exact same permissions as the original. Instead of manually setting each permission again, which can be time-consuming and error-prone, you can directly copy the security descriptor from the existing file to the new one using this command. This not only saves time but also ensures consistency across your file permissions.
Explanation:
$OriginAcl = Get-Acl -Path path\to\file: This part of the command retrieves the ACL, which includes all the permission settings, from the specified source file. The
Get-Acl
cmdlet stores this information in the$OriginAcl
variable.Get-Acl
: A cmdlet that fetches the security descriptor, which includes the owner, access control list, and auditing information of the specified file or directory.-Path path\to\file
: Specifies the path of the file from which the ACL is to be read. You have to replacepath\to\file
with the actual path location of your file.
Set-Acl -Path path\to\file -AclObject $OriginAcl: This part applies the retrieved ACL (stored in
$OriginAcl
) to the target file.Set-Acl
: This cmdlet is responsible for setting the security descriptors specified in its parameters.-Path path\to\file
: Indicates the target file path where the ACL will be applied.-AclObject $OriginAcl
: Assigns the previously fetched ACL to the specified file, effectively altering its permissions to match those of the source.
Example Output:
Upon execution, there is no direct text output from these commands in the PowerShell interface. However, the permissions of the target file are now identical to those of the origin file. You can verify this by running Get-Acl -Path path\to\file
on the target file.
Use Case 2: Use the Pipeline Operator to Pass a Descriptor
Code:
Get-Acl -Path path\to\file | Set-Acl -Path path\to\file
Motivation:
Pipelines are a powerful feature in PowerShell that allow for streamlined command execution by passing the output of one cmdlet directly as input to another. This use case demonstrates a more efficient way to transfer ACLs from one command to another without having to store them in a variable first. This method is not only concise but also aligns with PowerShell’s philosophy of chaining commands for more readable and maintainable scripts.
Explanation:
Get-Acl -Path path\to\file: As explained previously, this fetches the ACL information from the specified file.
-Path path\to\file
: Specifies the path of the file to be read. This needs to be replaced with the actual path of your file in use.
| Set-Acl -Path path\to\file: The pipeline operator
|
takes the output of theGet-Acl
command and feeds it into theSet-Acl
command.Set-Acl
: Sets the ACL using the data obtained from the pipeline.-Path path\to\file
: Specifies the target file where the ACL data from the previous command is applied.
Example Output:
Just as with the first use case, there isn’t any direct output visible on the command line. The action is performed silently, and permissions are set as intended. Verification can be executed via Get-Acl -Path path\to\file
.
Conclusion:
PowerShell’s Set-Acl
cmdlet offers a flexible and powerful way to modify security descriptors on files and registry keys, allowing system administrators to efficiently manage permissions and security policies across their environments. Whether copying permissions from one file to another or utilizing pipelines for streamlined command execution, Set-Acl
helps ensure consistency and security in systems management.