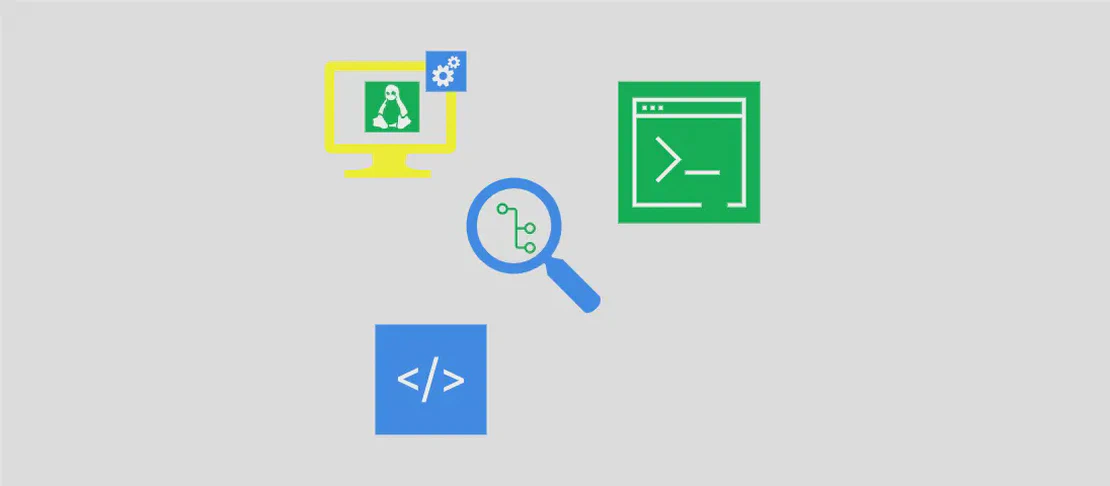
How to Use the Command 'Set-Date' in PowerShell (with examples)
The Set-Date
command in PowerShell is a versatile tool designed for managing and manipulating system time on a computer. By utilizing this command, users can adjust the system clock to any precise time or date. However, it is crucial to note that altering the system time can interfere with scheduled tasks, logging activities, and systems relying on accurate timestamps. Therefore, use this command judiciously and ensure that any changes align with the needs of your computing environment.
Use case 1: Add three days to the system date
Code:
Set-Date -Date (Get-Date).AddDays(3)
Motivation:
There are scenarios where testing future dated events or tasks is crucial. By adding three days to the current system date, a developer, tester, or IT professional can simulate a future environment to monitor how applications or systems react to the passage of time. This technique is particularly useful in testing time-sensitive functionalities such as software subscriptions or trial periods, ensuring calendar-based features, and scheduling tasks accurately.
Explanation:
Set-Date
: This is the primary command used to modify the system’s date and time.-Date
: This specifies that the argument following it will define a new date and time for the system clock.(Get-Date).AddDays(3)
: This component fetches the current date and adds three additional days.Get-Date
retrieves the present system date and time, andAddDays(3)
modifies it by adding three days to the current date.
Example Output:
If today’s date is October 1, the system will adjust to October 4. Any applications or events that check the date will now behave as if it is October 4.
Use case 2: Set the system clock back 10 minutes
Code:
Set-Date -Adjust -0:10:0 -DisplayHint Time
Motivation:
Adjusting the system clock backward by 10 minutes may be required to correct an undesired or accidental change in system time, or when synchronizing events or logs with another system that is slightly lagging behind. This is useful in network operations, database management, or any scenario where aligning timestamps for diagnostic or troubleshooting purposes is necessary.
Explanation:
Set-Date
: The command to modify the system’s date and time.-Adjust
: A parameter indicating that the change is relative to the current time, not an absolute setting to a specific date or time.-0:10:0
: The format for specifying the adjustment, representing negative 10 minutes. The format generally follows-hours:minutes:seconds
.-DisplayHint Time
: This parameter helps to visually optimize output by focusing solely on the time element of the adjustment in the console display.
Example Output:
If the current time is 2:00 PM, the system will adjust to display 1:50 PM. This backward shift in minutes is useful for aligning schedules and logs with precise system timing requirements.
Use case 3: Add 90 minutes to the system clock
Code:
$90mins = New-TimeSpan -Minutes 90; Set-Date -Adjust $90mins
Motivation:
Adding 90 minutes to the system clock can simulate future time changes that might affect an application or service. This is valuable during testing for systemic reactions over a time progression, for example, testing reminders, alarms, or any feature dependent on a specific future time.
Explanation:
$90mins = New-TimeSpan -Minutes 90;
: This creates a new time span object that represents a period of 90 minutes.New-TimeSpan
is used to define time intervals that can be easily manipulated.Set-Date -Adjust $90mins
: Applies the time span of 90 minutes to the current system time.-Adjust
shifts the system’s current time by the defined time span.
Example Output:
If the current time is 10:30 AM, after executing this command, the system will show 12:00 PM. This artificial forward shift helps to debug time-dependent features and logging dynamics.
Conclusion:
The Set-Date
command in PowerShell is a robust tool for controlling and experimenting with system time settings. It is especially beneficial for IT professionals, developers, and testers managing date and time-dependent applications and systems. By practicing these examples, users can gain a deeper understanding of how time manipulation can be effectively leveraged in diverse scenarios. However, always remember to use such modifications with caution to avoid unintended disruptions in regular system operations.