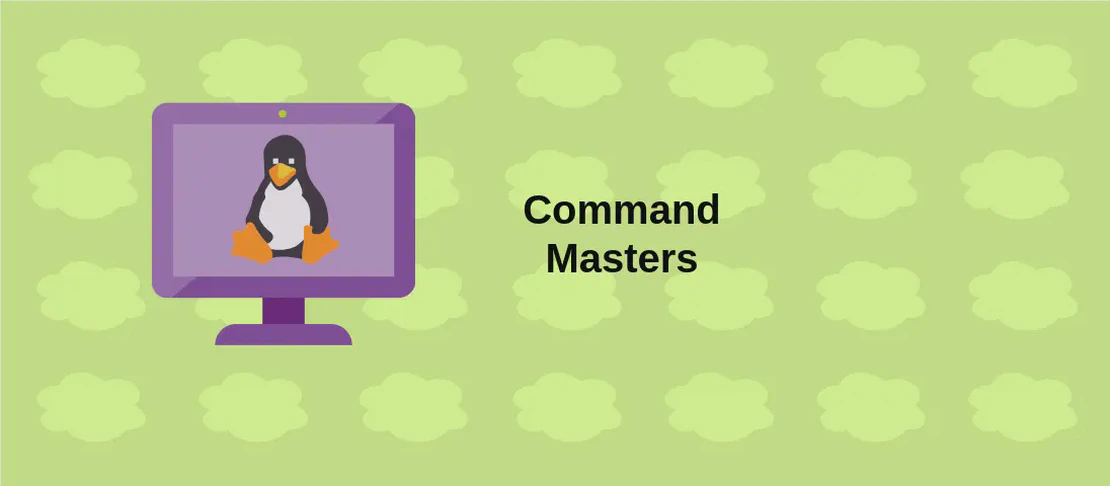
How to use the command 'Set-Location' (with examples)
The Set-Location
command in PowerShell is a versatile tool primarily used to manipulate and display the current working directory. This command allows users to navigate through the file system efficiently, enabling quick changes to different directories both within the same drive and across different drives. It supports several parameters to simplify directory navigation, making it an essential command for managing file paths and directories in PowerShell sessions.
Use case 1: Go to the specified directory
Code:
Set-Location path\to\directory
Motivation for using this example:
Navigating directly to a specified directory is a fundamental task when working in command-line environments. This use case is essential when you know the exact path and need to quickly move to that location to perform subsequent operations, such as accessing files or executing scripts located within that directory.
Explanation for every argument given in the command:
Set-Location
: This is the primary command used to change the current working directory.path\to\directory
: This argument specifies the path of the target directory to which you wish to navigate. It can be an absolute or relative path.
Example output:
After executing this command, there will be no visible output unless an error occurs. The command line will now be set to operate in the specified directory path.
Use case 2: Go to a specific directory in a different drive
Code:
Set-Location C:\path\to\directory
Motivation for using this example:
Sometimes files are stored across different drives on a system, especially on larger workstations or servers. When a user needs to access directories on different drives, quickly switching between them using Set-Location
ensures seamless navigation and enhances productivity, without needing to resort to GUIs or less efficient methods.
Explanation for every argument given in the command:
Set-Location
: Command utilized to switch directories.C:\path\to\directory
: In this example,C:
specifies the drive, and\path\to\directory
indicates the target directory on that drive you wish to navigate to.
Example output:
Similar to use case 1, executing this command will show no output, but your command line context will be changed to the specified directory on drive C.
Use case 3: Go and display the location of specified directory
Code:
Set-Location path\to\directory -PassThru
Motivation for using this example:
When scripting or working interactively, it might be beneficial to confirm the success of directory changes or output the new directory path for logging or informative purposes. The -PassThru
parameter accomplishes this by displaying the new directory location after performing the change.
Explanation for every argument given in the command:
Set-Location
: The main command to set the directory location.path\to\directory
: This specifies where to navigate within the file system.-PassThru
: An optional parameter that outputs the new location path, verifying the change for the user.
Example output:
The full path to the new directory is displayed on the console, confirming to the user the current directory location post-command execution.
Use case 4: Go up to the parent of the current directory
Code:
Set-Location ..
Motivation for using this example:
Moving upwards to a parent directory is a very common task when organizing files or scripts are located one level higher in the directory hierarchy. This is a convenient way to navigate without specifying a full path, saving time and effort, especially when moving through nested directory structures.
Explanation for every argument given in the command:
Set-Location
: Command that performs the directory change...
: A relative path shorthand that instructs PowerShell to move up one level to the parent directory of the current directory.
Example output:
No output will be produced, but the current directory is now set to the parent directory.
Use case 5: Go to the home directory of the current user
Code:
Set-Location ~
Motivation for using this example:
Accessing the home directory is particularly useful when you need to work with user-specific files, settings, or executables. The home directory is often a starting point for scripts and automation tasks that require user-specific configurations or permissions.
Explanation for every argument given in the command:
Set-Location
: The command utilized for directory change.~
: A shorthand symbol that represents the current user’s home directory, making it very easy and quick to access without explicit path information.
Example output:
Executing this command changes your working directory to the home directory of the current user context in PowerShell.
Use case 6: Go back/forward to the previously chosen directory
Code:
Set-Location -
Motivation for using this example:
When working inside nested filesystems or requiring back-and-forth navigation, using the Set-Location
command with -
as an argument allows quick toggling between two directories. This is an efficient way to retrace or re-enter directories recently navigated.
Explanation for every argument given in the command:
Set-Location
: The command for switching directory paths.-
: A special identifier which navigates to the previously chosen directory, effectively acting like a toggle between the last two directory paths you have been in.
Example output:
No output is produced, but the current directory switches to the previously active directory path.
Use case 7: Go to root of current drive
Code:
Set-Location \
Motivation for using this example:
Starting tasks from the root of the drive is sometimes necessary when working on system-wide scripts or needing to access top-level directories. This command quickly navigates the user to the base directory, essential for system administration and management.
Explanation for every argument given in the command:
Set-Location
: The standard command for changing directories.\
: This backslash is used to denote the root of the current drive, regardless of the current directory path.
Example output:
The command results in no output, but your working directory will now be the root of the current drive, allowing for operations and tasks on the drive’s full directory tree.
Conclusion:
With the Set-Location
command, PowerShell provides robust capabilities for directory navigation and management. Each of the use cases illustrates different practical applications, whether you’re navigating within drives, switching between previous directories, or accessing user-specific file locations. Mastery of these commands enhances efficiency and precision for users managing complex file systems or automating scripts in PowerShell environments.