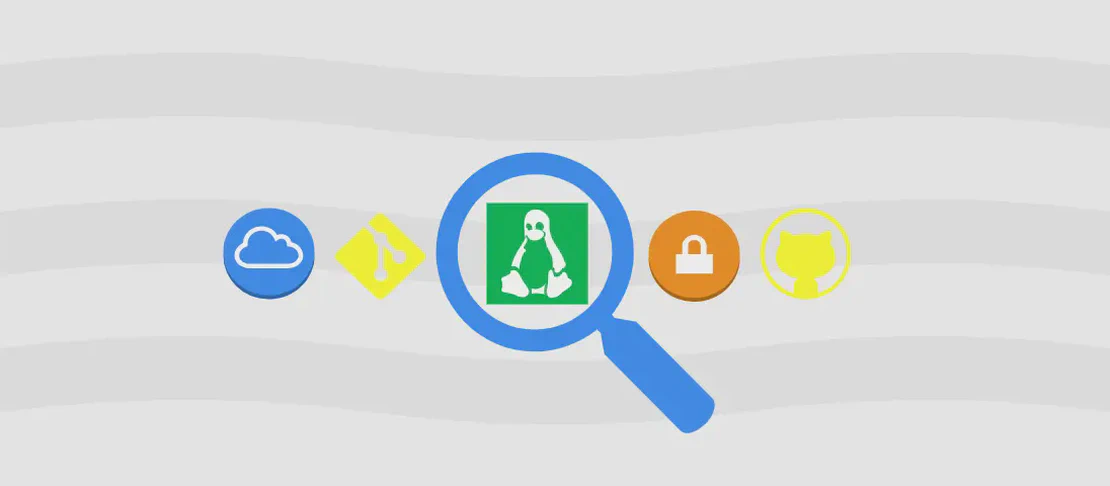
How to use the command 'sh' (with examples)
The sh
command refers to the Bourne shell, which is a standard command language interpreter used in Unix-like operating systems. It provides users and scripts the ability to execute commands and manipulate the shell environment. Though there are more advanced shells like bash
, sh
remains a vital part of many systems due to its simplicity and compatibility.
Use Case 1: Start an Interactive Shell Session
Code:
sh
Motivation:
Starting an interactive shell session using sh
allows users to engage directly with the command-line interface. This can be particularly useful for systems that use sh
as the default shell or for scripting purposes that require compatibility with a simple shell. Despite the modern prevalence of bash
, knowing how to enter an sh
session ensures that you remain versatile in different Unix environments.
Explanation:
The command sh
without any additional arguments simply launches the sh
shell in interactive mode. This means the shell will read commands from the user input and execute them immediately. It does not exit until the user explicitly chooses to do so using commands such as exit
.
Example Output:
$
This prompt indicates that the user is now in an sh
session, waiting for input.
Use Case 2: Execute a Command and Then Exit
Code:
sh -c "echo 'Hello, World!'"
Motivation:
This use case is beneficial when you want to execute a one-off command within an sh
environment without committing to an interactive session. This is particularly important for scripts that need to run commands in a predictable, straightforward shell setting or when testing commands in different shells.
Explanation:
-c
: This option tellssh
to read commands from the following string (in our example,"echo 'Hello, World!'"
)."echo 'Hello, World!'"
: This is the command string passed to the shell. It usesecho
to print “Hello, World!” to the standard output. The shell executes the command and exits immediately afterward.
Example Output:
Hello, World!
This shows that the command was successfully executed, and the shell subsequently exited.
Use Case 3: Execute a Script
Code:
sh path/to/script.sh
Motivation:
Executing scripts is one of the primary uses of sh
, facilitating automation and efficient task execution. Using sh
ensures that scripts written with basic shell syntax are run in a consistent environment, increasing reliability across various systems that support Unix-like operations.
Explanation:
path/to/script.sh
: This represents the path to the script you wish to execute. When the script is invoked usingsh
, the shell reads and executes commands found in the script file.
Example Output:
# Assuming script.sh contains:
# echo "Script is running"
# echo "Task complete"
Script is running
Task complete
This demonstrates that the shell successfully read and executed the commands within the script.
Use Case 4: Read and Execute Commands from stdin
Code:
sh -s
Motivation:
Running sh
to read commands from stdin
is advantageous for scenarios where command input may come from various dynamic sources, such as pipes. This allows for flexible command execution tailored to situations where input is not predetermined.
Explanation:
-s
: This flag initiates the shell to start reading commands from standard input (stdin
) until the input is exhausted. It is useful in scripts or when reading input interactively from a file or keyboard direct input.
Example Output:
To simulate reading from stdin
:
$ echo "echo Hello via stdin" | sh -s
Hello via stdin
This example shows the output when a command is piped into sh -s
, demonstrating the command’s execution directly from stdin
.
Conclusion:
The sh
command serves various critical functions across Unix-like systems, from initiating shell sessions to executing commands and scripts. Its versatility and simplicity make it indispensable despite advancements in other shells. Understanding sh
equips users with essential skills to handle a wide range of environments and tasks.