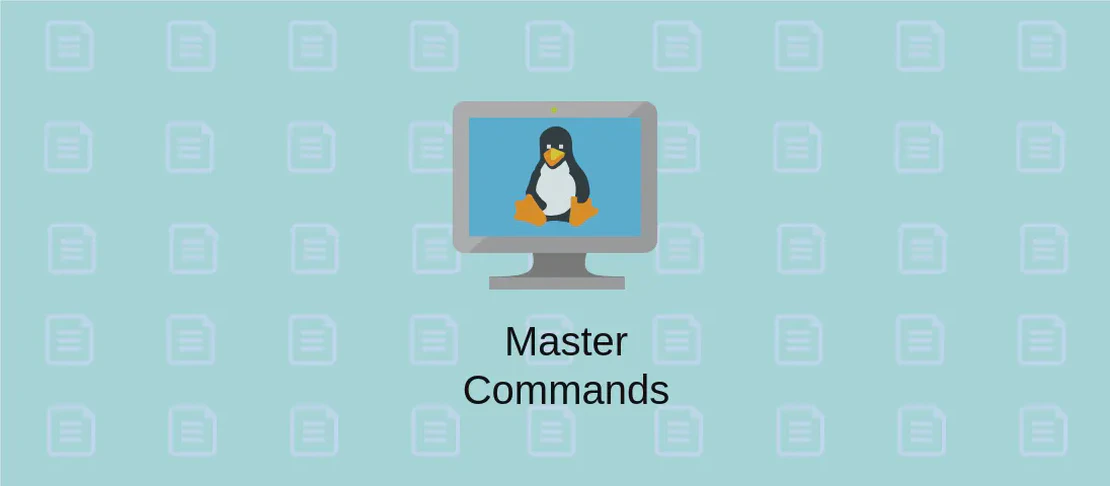
How to use the command 'shards' (with examples)
The shards
command is a dependency management tool for the Crystal language. It allows users to easily manage the dependencies of a Crystal project by providing functionality such as initializing a shard.yml
file, installing dependencies, updating dependencies, and listing installed dependencies.
Use case 1: Create a skeleton shard.yml
file
Code:
shards init
Motivation:
Creating a shard.yml
file is necessary for managing dependencies in a Crystal project. It defines the project’s dependencies and their specific versions.
Explanation:
The shards init
command initializes a skeleton shard.yml
file in the current directory. This file can then be edited to specify the project’s dependencies and their versions.
Example output:
Created shard.yml
Use case 2: Install dependencies from a shard.yml
file
Code:
shards install
Motivation:
Installing dependencies is a crucial step in setting up a Crystal project. By running this command, all the dependencies specified in the shard.yml
file will be downloaded and installed in the project.
Explanation:
The shards install
command looks at the shard.yml
file in the current directory and installs all the dependencies specified in it. It fetches the required dependencies and places them in the lib
directory of the project.
Example output:
Success
Use case 3: Update all dependencies
Code:
shards update
Motivation:
Keeping dependencies up to date is important for maintaining the stability and security of a project. By running this command, all the dependencies specified in the shard.yml
file will be updated to their latest versions.
Explanation:
The shards update
command looks at the shard.yml
file in the current directory and checks for updates to all the dependencies specified in it. It then updates the dependencies to their latest available versions.
Example output:
Fetching https://github.com/crystal-lang/crystallography.git
Fetching https://github.com/crystal-lang/crystal-mysql.git
Fetching https://github.com/crystal-lang/crystal-db.git
...
Use case 4: List all installed dependencies
Code:
shards list
Motivation: Listing installed dependencies can be helpful for understanding what external libraries and frameworks a project relies on.
Explanation:
The shards list
command lists all the dependencies that have been installed for the current project. It provides information such as the name of the dependency, its version, and the repository URL.
Example output:
marcelocr/crystagiri (0.2.4)
kemalyst/kemal-session-cookie (0.3.1)
crystal-lang/neph (v0.8.0)
...
Use case 5: List version of dependency
Code:
shards version path/to/dependency_directory
Motivation: Checking the version of a specific dependency can be useful when troubleshooting issues or verifying compatibility requirements.
Explanation:
The shards version
command retrieves the version of the dependency specified by the path/to/dependency_directory
argument. This argument should be the path to the directory where the dependency is located within the lib
directory of the project.
Example output:
crystal-mysql (0.4.0)
Conclusion:
The shards
command provides a convenient way to manage dependencies in a Crystal project. By using this command, developers can easily initialize a shard.yml
file, install and update dependencies, and view a list of installed dependencies. This tool simplifies the process of managing dependencies and ensures that the project’s external libraries and frameworks are correctly set up and maintained.