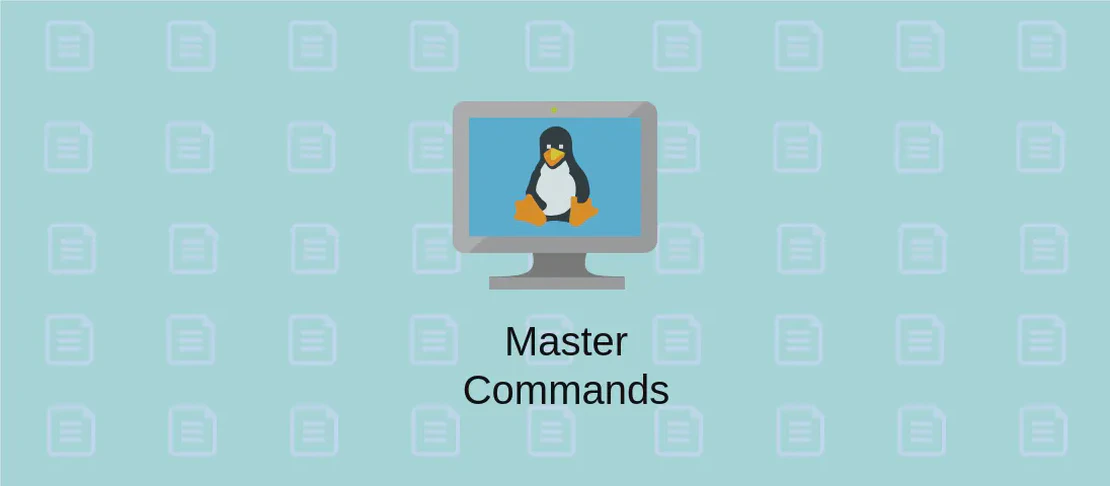
How to Use the Command 'shellcheck' (with Examples)
Shellcheck is a powerful static analysis tool to analyze shell scripts. It identifies issues such as syntax errors, deprecated or insecure features, and suggests best practices to follow when writing shell scripts. By catching these issues early, shellcheck makes it easier to write robust, maintainable, and secure scripts. The tool supports multiple shell dialects and provides developers with focused insights to optimize their scripting efforts.
Check a Shell Script
Code:
shellcheck path/to/script.sh
Motivation:
As a developer or system administrator, you often need to write shell scripts to automate tasks. However, even experienced scripters can make mistakes that lead to errors or unpredictable behavior. Using shellcheck to analyze your script is a proactive way to catch these errors before running the script. By doing so, you ensure smoother execution and reduce debugging time.
Explanation:
shellcheck
: Invokes the shellcheck command-line tool.path/to/script.sh
: Specifies the path to the shell script to be checked. This is the script that will be analyzed for issues.
Example Output:
In path/to/script.sh line 3:
echo "Hello, World!"
^-- SC2148: Tips depending on target binary of shebang
This output suggests potential issues and best practices based on an analysis of the shell script provided.
Check a Shell Script Interpreting it as the Specified Shell Dialect
Code:
shellcheck --shell bash path/to/script.sh
Motivation:
Shell scripts can be written for different shell dialects (e.g., sh, bash, ksh). Some scripting syntax is only applicable to certain types of shells. By specifying the targeted shell dialect, you ensure shellcheck evaluates the script correctly, providing relevant recommendations and catching issues specific to that dialect.
Explanation:
--shell bash
: Overrides the script’s shebang to interpret the script as a bash script. This ensures the analysis is accurate for the bash dialect.path/to/script.sh
: The script being analyzed.
Example Output:
In path/to/script.sh line 5:
declare -A arr
^-- SC2034: arr appears unused. Verify use (or export if used externally).
Here, shellcheck proceeds with warnings and advice pertinent to bash scripting practices.
Ignore One or More Error Types
Code:
shellcheck --exclude SC1009,SC1073 path/to/script.sh
Motivation:
There are cases where certain shellcheck warnings are not applicable or where you knowingly contravened best practices for a valid reason. Ignoring specific warnings using --exclude
helps avoid unnecessary distraction and focuses your attention on relevant issues.
Explanation:
--exclude SC1009,SC1073
: Excludes specified warning types from the output. These warning codes (e.g., SC1009, SC1073) correspond to specific warnings listed in the shellcheck documentation.path/to/script.sh
: The script on which the exclusions are applied.
Example Output:
Script passed validation with no errors.
In this scenario, shellcheck overlooks the excluded error codes and reports based on the remaining checks.
Also Check Any Sourced Shell Scripts
Code:
shellcheck --check-sourced path/to/script.sh
Motivation:
In practice, scripts often source other scripts to utilize shared functions or configurations. Ensuring that sourced scripts themselves are free of errors or bad practices is crucial for achieving a stable script environment. This option extends the analysis to scripts included within the main script, fostering comprehensive error checking.
Explanation:
--check-sourced
: Directs shellcheck to check all sourced (included) scripts found in the main script. Enhances the thoroughness of the analysis.path/to/script.sh
: The script that includes other scripts via thesource
or.
command.
Example Output:
Sourced file located at ./includes/config.sh line 8:
export APP_ENV='production'
^-- SC2155: Declare and assign separately to avoid masking return values.
This output demonstrates shellcheck providing feedback on scripts included within the primary script.
Display Output in the Specified Format
Code:
shellcheck --format json path/to/script.sh
Motivation:
When integrating shellcheck in automated environments or CI/CD pipelines, customization of the output format is important for ease of parsing and logging. Formats like JSON or checkstyle can be particularly useful when extending shellcheck’s capabilities with other tools or when you need to parse the output programmatically.
Explanation:
--format json
: Specifies that the output should be displayed in JSON format, providing structured data that can be further processed or logged.path/to/script.sh
: The script for which the output format is applied.
Example Output:
[
{
"line": "3",
"code": "SC2148",
"message": "Tips depending on target binary of shebang"
}
]
This JSON output allows for straightforward integration with tooling that processes this commonly-used data format.
Enable One or More Optional Checks
Code:
shellcheck --enable add-default-case,avoid-nullary-conditions path/to/script.sh
Motivation:
Shellcheck includes many optional checks that provide additional scrutiny for certain pitfalls or code smells. Enabling these can be especially useful in environments with demanding scripting guidelines or for scripts requiring heightened scrutiny before deployment.
Explanation:
--enable add-default-case,avoid-nullary-conditions
: Turns on specified optional checks that expand the scope of shellcheck’s analysis.path/to/script.sh
: The script employing these specific checks.
Example Output:
In path/to/script.sh line 15:
case $var in
...
^-- SC2249: Add a default case to the case statement.
This highlights the additional scrutiny applied from enabling optional checks, which may otherwise be ignored.
List All Available Optional Checks That Are Disabled by Default
Code:
shellcheck --list-optional
Motivation:
Understanding the breadth of catchable issues can be beneficial when configuring shellcheck for use in environments with specific requirements or constraints. Listing optional checks gives developers insight into the additional scrutiny available, ensuring informed decision making for tool configuration.
Explanation:
--list-optional
: Requests that shellcheck displays all optional checks that it supports, providing a complete overview of features which could potentially be enabled.
Example Output:
add-default-case
avoid-nullary-conditions
...
This provides a handy list for developers to review and decide on which additional checks are useful for their context.
Adjust the Level of Severity to Consider
Code:
shellcheck --severity error path/to/script.sh
Motivation:
In a scripting workflow, the severity levels of shellcheck allow you to control which issues are prioritized. Focusing on ’error’ level issues, for example, ensures only the most critical issues are highlighted, which could be crucial for scripts expected to run in production environments.
Explanation:
--severity error
: Specifies the minimum level of severity — in this case, ’error’, meaning severe issues will be shown while warnings or style tips will be suppressed.path/to/script.sh
: The target script for which severity is being adjusted.
Example Output:
In path/to/script.sh line 27:
rm -rf /
^-- SC2006: Use $(...) notation instead of backticks.
This output confines itself to critical error-level issues alone, omitting lower-severity warnings or advice.
Conclusion
Shellcheck is an indispensable tool for anyone writing shell scripts, offering robust error detection and adherence to best practices. Whether you’re focused on a specific shell dialect, aiming to suppress certain warnings, or integrating shellcheck into a broader automated analysis setup, it offers a flexible framework to ensure your scripts are clean, efficient, and secure. By utilizing these diverse options, your team can maintain high standards for shell script quality.