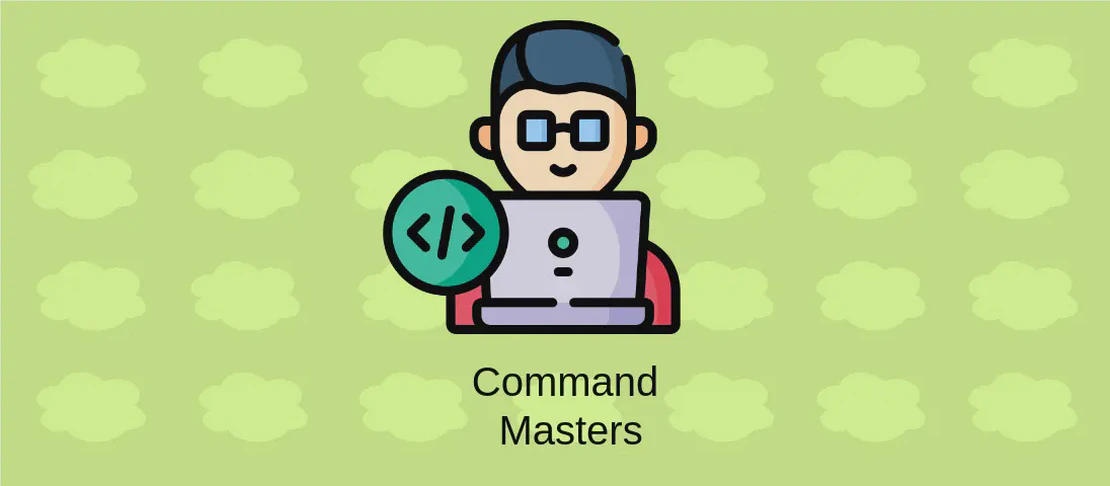
How to use the command 'shift' (with examples)
The shift
command is a built-in shell command commonly used in bash and other shell environments. Its primary function is to manipulate the list of positional parameters in a script. By removing the first positional parameter, or a specified number of them, it allows scripts to handle arguments dynamically and efficiently, especially when dealing with numerous arguments or a complex input structure. Below, we illustrate how this command can be utilized effectively in different scenarios.
Use case 1: Removing the first positional parameter
Code:
shift
Motivation:
Imagine you have a script that processes a list of filenames passed as arguments. With each iteration, you want to focus on processing one file at a time, and then discard it from the list to move on to the next. By using shift
, you can easily remove the first file from the argument list and efficiently manage the remainder. This is particularly useful in loops where each argument is processed individually.
Explanation:
shift
: This command, without any arguments, removes the first positional parameter from the list. Positional parameters are essentially the arguments passed to the script or function, which are accessed as$1
,$2
,$3
, and so on. After executingshift
,$1
becomes$2
,$2
becomes$3
, and so forth. Essentially, it shifts all parameters down by one position, effectively discarding the original first parameter.
Example output:
Suppose you’re running a script with the following command: ./myscript.sh file1.txt file2.txt file3.txt
.
Initially, the parameters are:
$1
: file1.txt$2
: file2.txt$3
: file3.txt
After executing shift
, the parameters become:
$1
: file2.txt$2
: file3.txt
Use case 2: Removing the first N
positional parameters
Code:
shift N
Motivation:
When you have a script designed to handle large batches of inputs, or you need to dynamically skip a predetermined set of parameters, removing multiple parameters in a single command can streamline your script significantly. For example, if the first few parameters are options or flags that are only relevant at the beginning of processing, you can discard them all at once to work directly with the remaining arguments, which could represent a set of data files or commands.
Explanation:
shift N
: In this version of the command,N
is a numeric argument that specifies the number of positional parameters to be removed. It allows you to shift the parameter list byN
places. For example, ifN
is 2, the command shifts all parameters so that the third parameter becomes the new first, the fourth becomes the second, and so on. It’s a more generalized form ofshift
that extends its utility to more complex argument handling.
Example output:
Given a command: ./myscript.sh -a -b file1.txt file2.txt file3.txt
, where -a
and -b
are option flags you wish to disregard after initial processing.
Initially, the parameters are:
$1
: -a$2
: -b$3
: file1.txt$4
: file2.txt$5
: file3.txt
After executing shift 2
, the parameters become:
$1
: file1.txt$2
: file2.txt$3
: file3.txt
Conclusion:
The shift
command is a powerful tool for managing positional parameters in shell scripts. Whether you need to process arguments one by one or skip over several at once, shift
helps maintain a clean and efficient workflow. By understanding how to apply shift
in these use cases, script writers can create more dynamic and adaptable scripts capable of handling diverse sets of input arguments seamlessly.