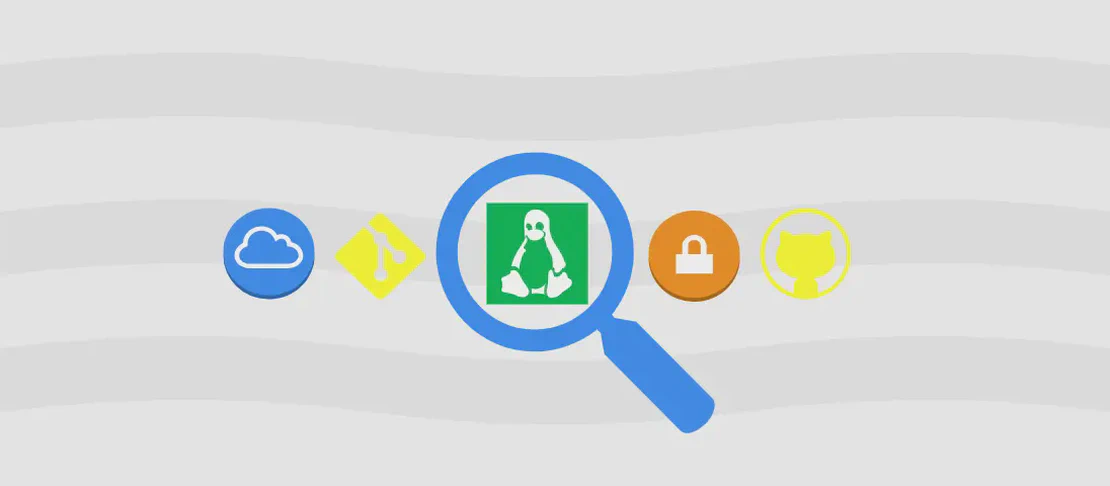
Mastering the `shuf` Command (with examples)
The shuf
command is a powerful utility part of the GNU Core Utilities package, designed to generate random permutations of input data. Its primary function is to randomize the order of lines from a file or standard input. This makes shuf
particularly useful in scenarios where randomness is desired, such as in statistical sampling, creating randomized lists, or simply shuffling data entries.
Use case 1: Randomize the order of lines in a file and output the result
Code:
shuf path/to/file
Motivation:
Randomizing the lines of a file can be beneficial in various contexts. For example, you might have a list of email addresses to whom you wish to send a promotional message, and you need to pick winners for a prize randomly. By shuffling the lines, you ensure randomness without bias.
Explanation:
shuf
: This is the command itself, which will begin the process of shuffling.path/to/file
: This represents the specific file whose lines are to be randomized. Replacing this with an actual file path will cause the lines within that file to be shuffled and printed to standard output.
Example output:
Assume the file contains:
Line 1
Line 2
Line 3
Line 4
After running shuf path/to/file
, an output might be:
Line 3
Line 1
Line 4
Line 2
Use case 2: Only output the first 5 entries of the result
Code:
shuf --head-count=5 path/to/file
Motivation:
When dealing with large datasets, you might not need to randomize all entries but rather just a subset. For example, in a randomized controlled trial, selecting a specific number of participants at random from a pool is crucial for unbiased sampling.
Explanation:
shuf
: Initiates the shuffling process.--head-count=5
: This argument limits the output to the first 5 lines after shuffling. Tailoring the number can optimize data handling and ensure scalability for large data sets.path/to/file
: Specifies the input file whose lines need to be shuffled and partially displayed.
Example output:
The file contains:
A
B
C
D
E
F
G
H
Output may look like:
G
A
C
F
E
Use case 3: Write the output to another file
Code:
shuf path/to/input_file --output=path/to/output_file
Motivation:
There are times when maintaining a record of the shuffled data is necessary, especially for audit purposes or further processing. Saving the output directly to another file can help in preserving the randomized state for future reference.
Explanation:
shuf
: Command to shuffle the lines.path/to/input_file
: The original file that contains the lines to be shuffled.--output=path/to/output_file
: Specifies where to write the shuffled output. This is efficient for cases where immediate access to the randomized data file is necessary without displaying it on screen.
Example output:
With the input file sample.txt
containing:
X
Y
Z
W
Running the command results in the file output.txt
containing:
W
X
Z
Y
Use case 4: Generate 3 random numbers in the range 1-10 (inclusive)
Code:
shuf --head-count=3 --input-range=1-10 --repeat
Motivation:
Generating random numbers within a range can be broadly applicable in simulations or lottery number generation. This method leverages randomness to facilitate various algorithmic needs that demand non-sequential inputs.
Explanation:
shuf
: Initiates the number shuffling process.--head-count=3
: Limits output to 3 random numbers.--input-range=1-10
: Sets the range from which numbers are randomly picked, ensuring inputs are within the specified values.--repeat
: Allows numbers to be picked repeatedly, which is useful for scenarios where repetition is not only allowed but expected.
Example output:
A possible output could be:
4
9
2
Conclusion:
The versatility of the shuf
command makes it an indispensable tool for anyone working with data files requiring random ordering. Whether it’s rearranging file entries, selecting a random subset, writing results to an output file, or generating random numbers, shuf
provides simplicity alongside robust functionality. By using shuf
, you can effectively incorporate randomness in your data handling tasks, ensuring unpredictability and variation in your processes.