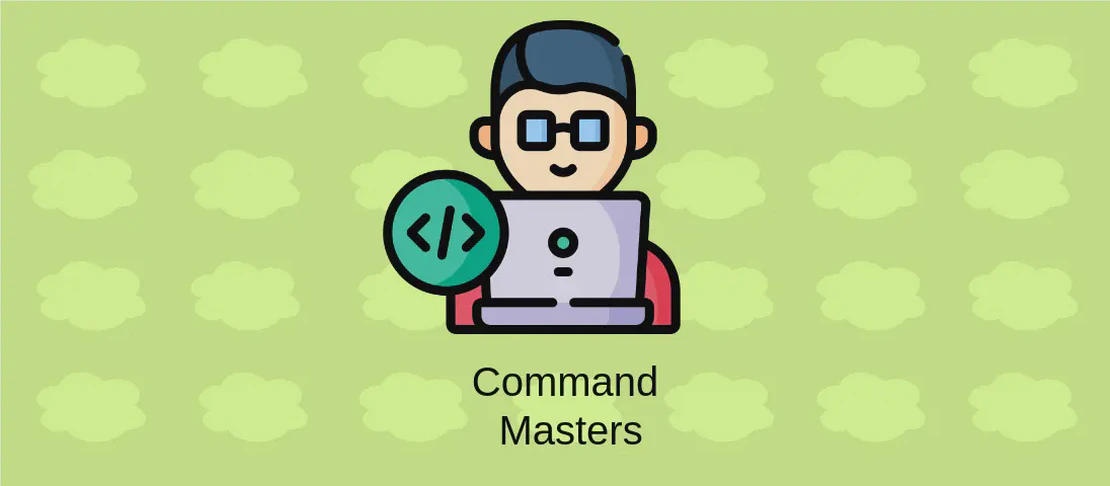
How to use the command 'simplehttpserver' (with examples)
The simplehttpserver
command is a straightforward and flexible tool used to create a simple HTTP or HTTPS server that provides features such as file uploads, basic authentication, and custom responses defined through YAML rules. It serves as a Go-based alternative to Python’s http.server
and is especially useful for developers looking for an easy-to-use server with a variety of configurable options.
Use case 1: Start the HTTP server serving the current directory with verbose output
Code:
simplehttpserver -verbose
Motivation:
Starting a local HTTP server with verbose output is quintessential for developers who need to inspect requests to the server in real time. This is particularly useful during the development and debugging processes, as it provides immediate feedback and insights into how the server handles different requests and how various resources are accessed.
Explanation:
simplehttpserver
: This is the command to start the server.-verbose
: This flag enables detailed logging of the server’s operations. It provides real-time information about each HTTP request received by the server, including headers, requests, and the server’s responses.
Example Output:
When you run the command, you might see outputs listing details of each request made to the server with timestamps, such as:
[2023-10-25T14:52:01] INFO - Serving HTTP on 0.0.0.0:8000
[2023-10-25T14:52:05] GET /index.html 200 OK
Use case 2: Start the HTTP server with basic authentication
Code:
sudo simplehttpserver -basic-auth username:password -path /var/www/html -listen 0.0.0.0:80
Motivation:
In environments where security matters, such as testing an application containing sensitive information, implementing basic authentication ensures only authorized users can access your resources. This is a basic security step that can protect against unauthorized data access.
Explanation:
sudo
: This is necessary because the server will listen on port 80, which typically requires administrative privileges.simplehttpserver
: Starts the server.-basic-auth username:password
: Sets up basic HTTP authentication with the specified username and password combination.-path /var/www/html
: This determines which directory’s contents will be served; in this case, it is/var/www/html
.-listen 0.0.0.0:80
: Configures the server to listen on all interfaces, using the default HTTP port 80.
Example Output:
A typical log entry with basic authentication might look like this upon an authorized access attempt:
[2023-10-25T15:12:45] INFO - Authenticated user: username
[2023-10-25T15:12:46] GET /home.html 200 OK
Use case 3: Start the HTTP server, enabling HTTPS using a self-signed certificate
Code:
sudo simplehttpserver -https -domain *.selfsigned.com -listen 0.0.0.0:443
Motivation:
Using HTTPS is critical as it encrypts data in transit between the server and clients, making it difficult for attackers to intercept and tamper with the communication. This is especially important when dealing with confidential information.
Explanation:
sudo
: Needed due to privileges required to listen on port 443.simplehttpserver
: Initiates the server.-https
: Enables HTTPS, allowing secure data transmission.-domain *.selfsigned.com
: Specifies a wildcard domain for the self-signed certificate, which allows the server to support multiple subdomains.-listen 0.0.0.0:443
: The server will listen on port 443 for secure HTTPS connections, accessible from all interfaces.
Example Output:
After starting, the server output might be:
[2023-10-25T16:00:00] INFO - HTTPS server running on https://0.0.0.0:443
Use case 4: Start the HTTP server with custom response headers and upload capability
Code:
simplehttpserver -upload -header 'X-Powered-By: Go' -header 'Server: SimpleHTTPServer'
Motivation:
Custom response headers are useful for providing additional information to clients or implementing additional functionality such as enabling CORS or security-related headers. Enabling upload capabilities allows users to upload files directly through the server interface.
Explanation:
simplehttpserver
: Begins running the server.-upload
: Enables the capability for users to upload files to the server.-header 'X-Powered-By: Go' -header 'Server: SimpleHTTPServer'
: Adds custom HTTP response headers, which can be used for various purposes, including branding or API versioning.
Example Output:
Upon receiving an upload request, output might display:
[2023-10-25T16:20:30] INFO - File uploaded successfully: report.pdf
Use case 5: Start the HTTP server with customizable rules in YAML
Code:
simplehttpserver -rules rules.yaml
Motivation:
The ability to define server behavior using YAML rules brings powerful flexibility, allowing detailed custom responses, request redirections, or even creating mocked server environments for testing APIs.
Explanation:
simplehttpserver
: Invokes the server.-rules rules.yaml
: Loads customizable server rules from a specified YAML file, dictating the server’s responses to various requests.
Example Output:
The output might display rule-based actions or logs, such as:
[2023-10-25T16:45:15] INFO - Rule applied: Redirecting /old-path to /new-path
Conclusion:
The simplehttpserver
command offers a wide range of functionalities to fit diverse needs, from simple file serving tasks and secure connections to detailed request tracking and customizable YAML rules handling. By explaining each use case, this article aimed to guide users through various scenarios where this tool can be effectively applied, enhancing both development and testing environments.