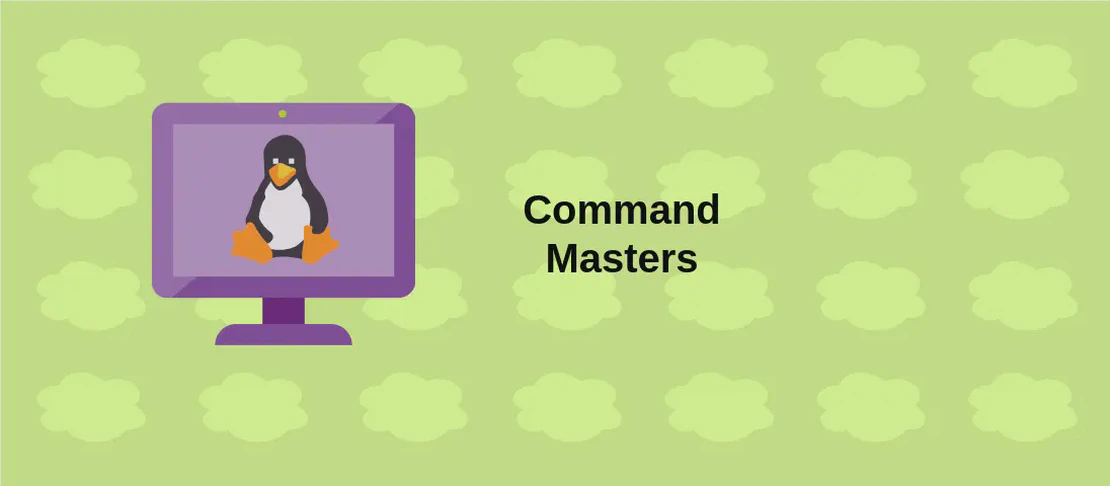
How to Use the Command 'solcjs' for Compiling Solidity Contracts (with Examples)
‘solcjs’ is a versatile command-line tool providing JavaScript bindings for the Solidity compiler. It plays a crucial role in blockchain development, particularly for Ethereum-based projects. By translating Solidity code into bytecode, it enables smart contracts to be deployed and executed on the Ethereum blockchain. ‘solcjs’ offers various use cases tailored to different needs in the contract compilation process, each with specific arguments and outputs.
Compile a Specific Contract to Hex
Code:
solcjs --bin path/to/file.sol
Motivation:
Compiling a Solidity contract into hexadecimal bytecode is a critical step in deploying a contract onto the Ethereum blockchain. This bytecode is what the Ethereum Virtual Machine (EVM) executes. By generating the binary, developers can test bytecode correctness, perform security audits, and prepare for deployment.
Explanation:
solcjs
: This is the command-line tool invoking the Solidity compiler through JavaScript bindings.--bin
: This flag instructs ‘solcjs’ to generate the binary (hexadecimal bytecode) for the specified Solidity file.path/to/file.sol
: This represents the file path to the Solidity contract you intend to compile into hex bytecode.
Example Output:
If your Solidity source code is in MyContract.sol
, the output in the terminal would be something like:
path/to/file_sol_MyContract.bin
And within this file, you will find the hexadecimal representation of your contract’s bytecode, ready for deployment.
Compile the ABI of a Specific Contract
Code:
solcjs --abi path/to/file.sol
Motivation:
The Application Binary Interface (ABI) is essential for interacting with a deployed contract through code, as it defines the contract’s callable functions and their parameters. Generating the ABI is crucial for integrating your smart contract with web applications or other contracts.
Explanation:
solcjs
: Invokes the Solidity compiler tool through JavaScript.--abi
: This flag tells ‘solcjs’ to produce the ABI file, describing the interface of the compiled contract.path/to/file.sol
: Specifies the Solidity source file for which the ABI is being generated.
Example Output:
Running this command on MyContract.sol
will create a file such as:
path/to/file_sol_MyContract.abi
This file contains JSON-formatted data representing the contract’s interface.
Specify a Base Path to Resolve Imports From
Code:
solcjs --bin --base-path path/to/directory path/to/file.sol
Motivation:
Solidity contracts often include imports of other contracts or libraries. Specifying a base path helps the compiler know where to resolve these imports, ensuring all dependencies are correctly compiled together with the main contract.
Explanation:
solcjs
: The command-line interface of the Solidity compiler.--bin
: Directs ‘solcjs’ to generate the hexadecimal bytecode.--base-path path/to/directory
: Sets a base directory from which relative import statements in the Solidity contracts are resolved.path/to/file.sol
: Path to the Solidity file being compiled.
Example Output:
For MyContract.sol
with appropriate imports, you get a binary file:
path/to/file_sol_MyContract.bin
This result means all imports were correctly resolved using the base path specified.
Specify One or More Paths to Include Containing External Code
Code:
solcjs --bin --include-path path/to/directory path/to/file.sol
Motivation:
Sometimes contracts rely on external libraries or code that reside outside the current directory. By specifying include paths, ‘solcjs’ can locate and integrate these external pieces into the final compiled product.
Explanation:
solcjs
: Activate the Solidity compiler via JavaScript.--bin
: Generates the binary output required for deployment.--include-path path/to/directory
: Sets paths to look for external code required in the contracts.path/to/file.sol
: The primary Solidity contract needing compilation.
Example Output:
With external references resolved, executing the command will produce a binary file like:
path/to/file_sol_MyContract.bin
This indicates successful external dependency integration.
Optimise the Generated Bytecode
Code:
solcjs --bin --optimize path/to/file.sol
Motivation:
Optimizing bytecode is essential for ensuring efficiency and lowering contract deployment costs on Ethereum. An optimized contract uses fewer resources on the blockchain, making it more economical for users and developers.
Explanation:
solcjs
: The tool for compiling Solidity contracts.--bin
: Command to output binary code.--optimize
: Optimizes the compiled bytecode, improving performance and reducing size.path/to/file.sol
: The file path to the Solidity contract being compiled and optimized.
Example Output:
The command produces a more efficient binary file, shown as:
path/to/file_sol_MyContract.bin
This file contains an optimized version of the contract’s bytecode, often smaller and faster.
Conclusion:
The ‘solcjs’ command-line tool offers a powerful way to work with Ethereum Solidity contracts, enabling developers to compile, optimize, and prepare contracts for deployment efficiently. Each command and argument provide specific functionalities crucial for the full spectrum of blockchain development tasks, from initial setup to final deployment.