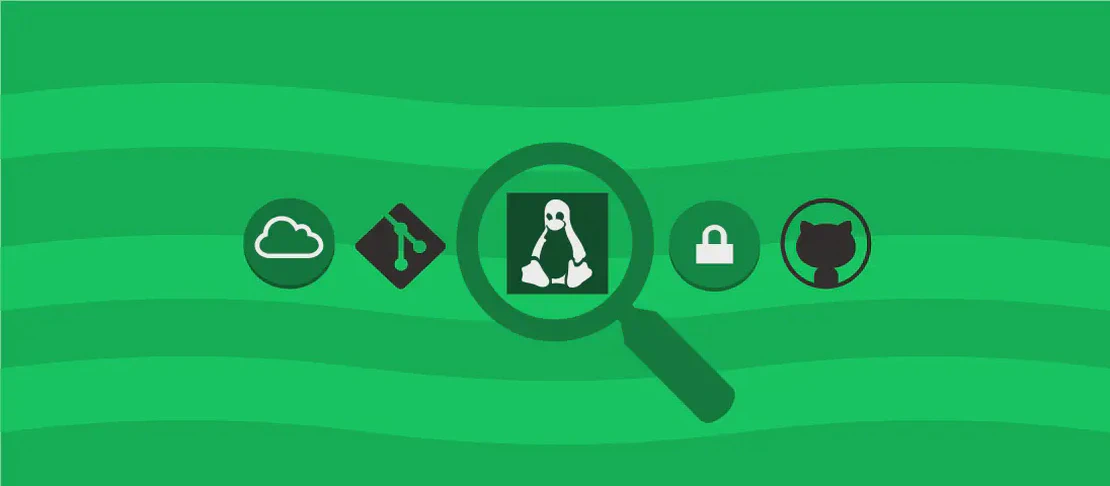
How to use the command 'Sort-Object' (with examples)
The Sort-Object
command is a powerful tool available in PowerShell that allows users to sort objects based on their property values. Whether you’re managing files, working with processes, or organizing data, sorting is often a necessary task to help you make informed decisions and streamline your workflow. Sort-Object
offers flexibility with options for ascending and descending order, uniqueness, and specific property targeting to suit various needs.
Use case 1: Sort the current directory by name
Code:
Get-ChildItem | Sort-Object
Motivation:
In many situations, organizing the contents of a directory can significantly improve file management and accessibility. By default, folders and files listed alphabetically make it easier for users to locate specific items within a directory. This command helps you achieve a neat and systematic order of your directory contents.
Explanation:
Get-ChildItem
: This part of the command retrieves the items from the current directory. It’s akin tols
in a Unix-based shell, listing both files and subdirectories.|
: The pipe symbol takes the output of theGet-ChildItem
command and passes it as input to the next command.Sort-Object
: This filters and sorts the items lexicographically by name by default, resulting in an ordered list.
Example Output:
Directory: C:\Example
Mode LastWriteTime Length Name
---- ------------- ------ ----
-a---- 10/15/2023 10:30 AM 23456 Document.docx
-a---- 10/14/2023 02:25 PM 45678 Image.png
d----- 10/13/2023 03:12 PM SubFolder1
d----- 10/12/2023 11:13 AM SubFolder2
-a---- 10/12/2023 04:56 PM 12345 Text.txt
Use case 2: Sort the current directory by name descending
Code:
Get-ChildItem | Sort-Object -Descending
Motivation:
Sometimes, you may need to view the most recently added or modified files and folders at the beginning rather than the end of a list. This is particularly useful if you’re dealing with directories with a large number of items and you are primarily interested in recent updates or additions.
Explanation:
Get-ChildItem
: Retrieves directory items.|
: Pipes the output to the next command.Sort-Object -Descending
: Sorts the directory contents in reverse alphabetical order. The-Descending
flag reverses the typical ascending order, placing ‘Z’ before ‘A’.
Example Output:
Directory: C:\Example
Mode LastWriteTime Length Name
---- ------------- ------ ----
-a---- 10/12/2023 04:56 PM 12345 Text.txt
d----- 10/12/2023 11:13 AM SubFolder2
d----- 10/13/2023 03:12 PM SubFolder1
-a---- 10/14/2023 02:25 PM 45678 Image.png
-a---- 10/15/2023 10:30 AM 23456 Document.docx
Use case 3: Sort items removing duplicates
Code:
"a", "b", "a" | Sort-Object -Unique
Motivation:
Handling duplicate data is a frequent need when processing lists or datasets. You often need to ensure that operations are performed on unique sets of data to avoid redundancy in your work. This command helps in filtering out duplicates in scenarios like preparing unique lists from user inputs, databases, or configuration files.
Explanation:
"a", "b", "a"
: This is an array of strings, with ‘a’ appearing twice.|
: Pipes the array toSort-Object
.Sort-Object -Unique
: Sorts the array and removes any duplicates, ensuring each element appears only once in the final output.
Example Output:
a
b
Use case 4: Sort the current directory by file length
Code:
Get-ChildItem | Sort-Object -Property Length
Motivation:
Sorting files by size can be crucial, especially when managing storage space. Knowing which files take up the most space can help prioritize deletions or transfers. This can also assist in optimizing backups, file sharing, or even forensic data analysis.
Explanation:
Get-ChildItem
: Lists the files and directories.|
: Transfers the listing to the sorting function.Sort-Object -Property Length
: Sorts the files based on their length property (size), from smallest to largest by default.
Example Output:
Directory: C:\Example
Mode LastWriteTime Length Name
---- ------------- ------ ----
-a---- 10/12/2023 04:56 PM 12345 Text.txt
-a---- 10/15/2023 10:30 AM 23456 Document.docx
-a---- 10/14/2023 02:25 PM 45678 Image.png
d----- 10/12/2023 11:13 AM SubFolder2
d----- 10/13/2023 03:12 PM SubFolder1
Use case 5: Sort processes with the highest memory usage based on their working set (WS) size
Code:
Get-Process | Sort-Object -Property WS
Motivation:
System administrators and power users often need to monitor and manage system resources. By sorting processes by memory usage, you can quickly identify processes that are consuming excessive resources. This insight is vital for performance tuning, resource allocation, and troubleshooting applications that may misbehave.
Explanation:
Get-Process
: Gathers a list of all processes currently running on a system.|
: Passes the list toSort-Object
.Sort-Object -Property WS
: Orders the processes by the working set memory size in an ascending manner. The WS (Working Set) property represents the amount of memory a process is using.
Example Output:
Handles NPM(K) PM(K) WS(K) VM(M) CPU(s) Id ProcessName
------- ------ ----- ----- ----- ------ -- -----------
1 0 4 4 1 0.00 4852 smartscreen
453 20 204 644 33 0.31 2424 conhost
198 14 1556 3320 68 0.47 991 cmd
1334 95 13264 234852 612 3.11 3652 powershell
Conclusion:
The Sort-Object
command in PowerShell provides versatile sorting capabilities essential for effective data management, system monitoring, and file operations. With various options like sorting by property, enforcing unique elements, and choosing order direction, it proves invaluable across diverse scenarios. By mastering these examples, you can enhance file organization, optimize system resources, and streamline data processing tasks with ease.