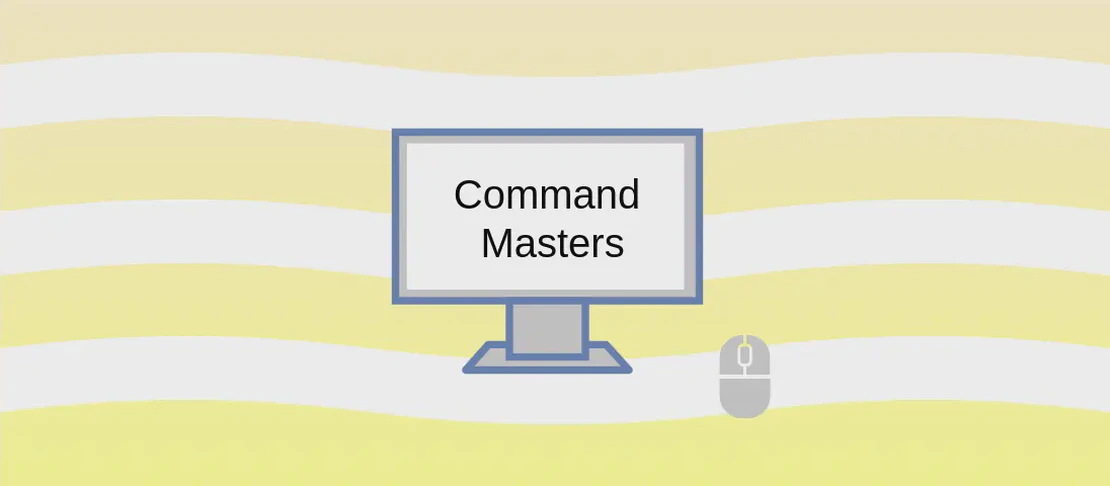
How to use the command 'sqlite-utils' (with examples)
The sqlite-utils
command-line tool is a versatile utility designed to facilitate interaction with SQLite databases. It allows users to easily create and manipulate SQLite databases and their associated tables from the command line. This powerful tool focuses on simplifying the management of SQLite databases through a clear and concise command syntax. With sqlite-utils
, users can execute a variety of operations such as creating databases and tables, inserting or updating records, querying for data, and more, all with straightforward commands.
Use case 1: Create a Database
Code:
sqlite-utils create-database path/to/database.db
Motivation for the Example: Creating a database is the fundamental first step when working with any database management system, including SQLite. This command is useful for initializing a new workspace where data can be stored and managed. If you’re starting a new project that requires data storage or data manipulation, you’ll need to create a database as your initial step.
Explanation of the Command:
sqlite-utils
: This is the main command that invokes the SQLite utilities.create-database
: This specific command tellssqlite-utils
that you want to create a new database.path/to/database.db
: This is the file path where the new SQLite database will be created. You can specify the directory and the name of your database file.
Example Output:
Upon execution, no explicit output will be shown if successful, but a new file named database.db
is created at the specified path, representing the new SQLite database ready for use.
Use case 2: Create a Table
Code:
sqlite-utils create-table path/to/database.db table_name id integer name text height float photo blob --pk id
Motivation for the Example: Once you have a database, you’ll want to organize your data within it, and creating tables is how you achieve that. This command provides a way to define the structure of your table, which is essential for enforcing data integrity and organizing your information in a meaningful way.
Explanation of the Command:
sqlite-utils
: The primary command used to access SQLite utilities.create-table
: This action specifies that you want to create a new table within the designated database.path/to/database.db
: Indicates the location of the database where the table will be created.table_name
: This is the name you assign to your new table.id integer name text height float photo blob
: These represent the columns of your table and their respective data types.id
is an integer,name
is text,height
is a float, andphoto
is a binary large object (blob).--pk id
: This flag indicates that the columnid
is to be used as the primary key for the table. A primary key uniquely identifies each record in the table.
Example Output:
No direct output is visible on the terminal, but a table named table_name
is now part of your database.db
with the specified structure.
Use case 3: List Tables
Code:
sqlite-utils tables path/to/database.db
Motivation for the Example: Listing the tables in a database helps you verify the structure you’ve set up and understand what data structures are available within a database. It’s a quick way to validate your database’s setup or become familiar with its organization.
Explanation of the Command:
sqlite-utils
: Invokes the SQLite utility.tables
: This command is used specifically to list all tables that exist within a given database.path/to/database.db
: The path specifies which database’s tables you want to list.
Example Output: The command outputs a list of table names within the database. For instance:
table_name
Use case 4: Upsert a Record
Code:
echo '[ {"id": 1, "name": "Linus Torvalds"}, {"id": 2, "name": "Steve Wozniak"}, {"id": 3, "name": "Tony Hoare"} ]' | sqlite-utils upsert path/to/database.db table_name - --pk id
Motivation for the Example: The ability to upsert records (a combination of update and insert) allows for the seamless management of data, especially when you are uncertain whether the data already exists. This can prevent duplication and ensure that if a record already exists, it is updated instead of creating a new entry.
Explanation of the Command:
echo '[...]'
: This part usesecho
to provide JSON data representing the records to be inserted or updated.|
: The pipe operator sends the output of the echo command tosqlite-utils
.sqlite-utils
: The main command for SQLite actions.upsert
: This instructssqlite-utils
to either update an existing record or insert it if it does not exist.path/to/database.db
: The database in which the records will be upserted.table_name
: The table within the database that will receive the records.-
: A placeholder that signalssqlite-utils
to read JSON data from standard input.--pk id
: Identifiesid
as the primary key, which is used to determine if the record should be an update or an insert.
Example Output:
The table table_name
in database.db
is populated or updated with the following records:
id name
1 Linus Torvalds
2 Steve Wozniak
3 Tony Hoare
Use case 5: Select Records
Code:
sqlite-utils rows path/to/database.db table_name
Motivation for the Example: Fetching records from a table is crucial for viewing and analyzing data stored within your database. This command serves as a basic way to check the contents of your database tables and verify that your data is correctly stored.
Explanation of the Command:
sqlite-utils
: The core SQLite utility command.rows
: This command fetches and displays records from the specified table.path/to/database.db
: Specifies the database from which to select records.table_name
: The table from which data will be retrieved.
Example Output:
The rows stored in the table named table_name
are displayed. For example:
id name
1 Linus Torvalds
2 Steve Wozniak
3 Tony Hoare
Use case 6: Delete a Record
Code:
sqlite-utils query path/to/database.db "delete from table_name where name = 'Tony Hoare'"
Motivation for the Example: There may be times when incorrect data needs to be removed or when records need to be deleted to maintain data relevancy. This command provides a direct way to remove specific records based on given criteria.
Explanation of the Command:
sqlite-utils
: The SQLite utilities command initiator.query
: Allows for direct execution of any SQL query.path/to/database.db
: Points to the specific database where the deletion should occur."delete from table_name where name = 'Tony Hoare'"
: An SQL query that instructs SQLite to delete records fromtable_name
where the name isTony Hoare
.
Example Output:
There is no explicit output, but the record with the name “Tony Hoare” is removed from table_name
. Verifying with a select
command will show:
id name
1 Linus Torvalds
2 Steve Wozniak
Use case 7: Drop a Table
Code:
sqlite-utils drop-table path/to/database.db table_name
Motivation for the Example: Dropping a table is a critical operation used during database maintenance and restructuring. It allows you to remove an entire table and its data from the database, which may be necessary if the table is no longer needed or requires a redesign.
Explanation of the Command:
sqlite-utils
: Invokes the SQLite toolkit.drop-table
: Specifies the operation to drop a table.path/to/database.db
: Identifies the database containing the table to be dropped.table_name
: The name of the table you intend to remove.
Example Output:
The table table_name
is deleted from the database database.db
. Any attempt to list the tables afterward will show that table_name
no longer exists.
Use case 8: Display Help
Code:
sqlite-utils -h
Motivation for the Example: Accessing help documentation is vital for learning more about available commands, their options, and usage nuances. This command acts as a guide for both new users and experienced developers needing a refresher or looking to explore additional functionalities.
Explanation of the Command:
sqlite-utils
: The initial SQLite utilities command.-h
: A flag that prints the help documentation, detailing all commands, parameters, and usage examples provided bysqlite-utils
.
Example Output: The help documentation is displayed, offering a comprehensive list of commands and options:
Usage: sqlite-utils [OPTIONS] COMMAND [ARGS]...
Command-line tool for manipulating SQLite databases.
Options:
-h, --help Show this message and exit.
Commands:
create-database Create a new SQLite database
create-table Create a new table in the database
...
Conclusion:
The sqlite-utils
command-line tool is an indispensable resource for anyone working with SQLite databases, offering intuitive commands for performing a breadth of operations from database creation to complex data manipulation. By leveraging sqlite-utils
, users can efficiently interact with SQLite databases directly from their terminal, streamlining processes that might otherwise require extensive GUI navigation or advanced SQL scripting skills.