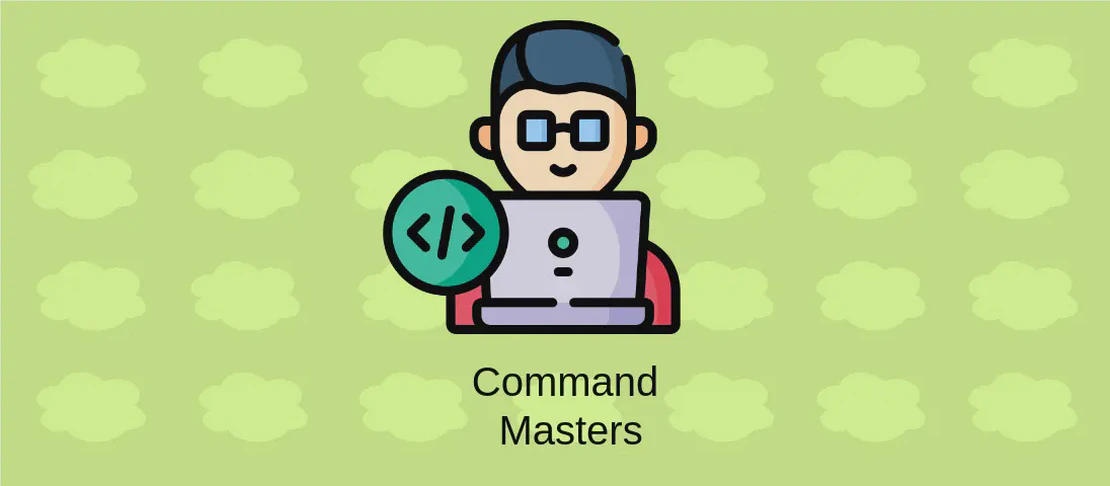
How to Use the Command 'stack' (with Examples)
Stack is a powerful tool for managing Haskell projects. It’s designed to help Haskell developers streamline their workflow, manage dependencies, and automate various aspects of the software development lifecycle. This command-line tool integrates seamlessly with the Haskell language, providing a standardized way to create, build, and test Haskell applications. More information about Stack can be found on its GitHub page .
Use Case 1: Create a New Package
Code:
stack new package template
Motivation:
When starting a new Haskell project, setting up the initial package structure can be overwhelming, especially for newcomers. Stack simplifies this process by generating a new package scaffolding based on a specified template. This ensures consistency and eliminates the need for repetitive boilerplate code setup, allowing developers to focus more on writing actual business logic.
Explanation:
stack new
: This part of the command tells Stack to create a new project from scratch.package
: This argument specifies the name of the new package you want to create.template
: This refers to the predefined template you want to use for your package. Templates provide a foundation structure including sample files and configurations.
Example output:
Downloading template "template" to create "package" ...
Using resolver: lts-19.12 ...
Creating new package "package" ...
Package "package" created successfully.
Use Case 2: Compile a Package
Code:
stack build
Motivation:
Compiling a Haskell package is a crucial step in the development process. The stack build
command compiles all the modules in the package, resolves dependencies, and checks for errors. This step ensures that the codebase is up-to-date and free of compilation errors, making it ready for testing or production deployment.
Explanation:
stack build
: This simple yet powerful command invokes the build process for the entire project, taking care of downloading and compiling any missing dependencies and ensuring all source files are compiled.
Example output:
Building all executables for `package' once. After a successful build of all of them, only specified executables will be rebuilt.
package> configure
Configuring package-0.1.0.0...
package> build
Building package-0.1.0.0...
package> copy/register
Installing library in...
Completed 2 action(s).
Use Case 3: Run Tests Inside a Package
Code:
stack test
Motivation:
Testing is a fundamental part of software development. Running tests ensures that new changes haven’t broken existing functionality and that new features work as expected. The stack test
command allows developers to execute the test suites defined in their package, providing valuable feedback on the stability and reliability of the code.
Explanation:
stack test
: Executes the test suites within the package, automatically compiling the test code and providing a report of the test results.
Example output:
package> test
Running 1 test suites...
Test suite package-test: RUNNING...
package-test: Test suite package-test passed
Use Case 4: Compile a Project and Re-Compile Every Time a File Changes
Code:
stack build --file-watch
Motivation:
The stack build --file-watch
command enhances productivity by automatically rebuilding the project whenever a file is edited. This feature is incredibly helpful during development as it removes the need to manually trigger a build after each change, reducing the feedback loop time and allowing developers to see the effects of their changes immediately.
Explanation:
stack build
: Initiates the build process for the project as usual.--file-watch
: This flag tells Stack to watch the file system for changes and recompile automatically whenever a source file is modified.
Example output:
Watching for changes...
Project successfully built.
Rebuilding due to change in src/Main.hs ...
Use Case 5: Compile a Project and Execute a Command After Compilation
Code:
stack build --exec "command"
Motivation:
Sometimes it is necessary to perform additional actions after building the project, such as running a particular setup script or executing a newly compiled executable. The --exec
option enables automated execution of specified commands, streamlining workflows and eliminating unnecessary manual steps in the development and deployment process.
Explanation:
stack build
: As with previous examples, this command triggers the project build process.--exec "command"
: This flag specifies a command to run immediately after a successful build. Replace"command"
with any shell command or program you want to execute.
Example output:
Building all executables for `package' once...
package> configure
Compiling package-0.1.0.0...
Run command after build: `command`
Executing command: command
Command successfully executed.
Use Case 6: Run a Program and Pass an Argument to It
Code:
stack exec program -- argument
Motivation:
Haskell programs often need command-line arguments to function correctly in various contexts. stack exec
provides a convenient way to run an executable within the project’s environment, ensuring all dependencies are properly set up, while also allowing command-line arguments to be passed seamlessly.
Explanation:
stack exec
: Runs the specified program in the context of the project’s build environment.program
: The name of the executable to run. This can be a program defined within the package or a tool available in the project’s environment.--
: This separator indicates that what follows are command-line arguments for theprogram
.argument
: Any command-line argument thatprogram
requires for execution.
Example output:
Running program with argument: argument
Program output: "Argument received."
Conclusion:
The Stack command-line tool is an invaluable resource for Haskell developers, providing a range of functionalities from creating new packages to running and testing programs. Each use case highlighted above serves a specific purpose, demonstrating the versatility and convenience of Stack in managing Haskell projects efficiently. By leveraging these commands, developers can improve their workflows, maintain code consistency, and ultimately deliver robust Haskell applications.