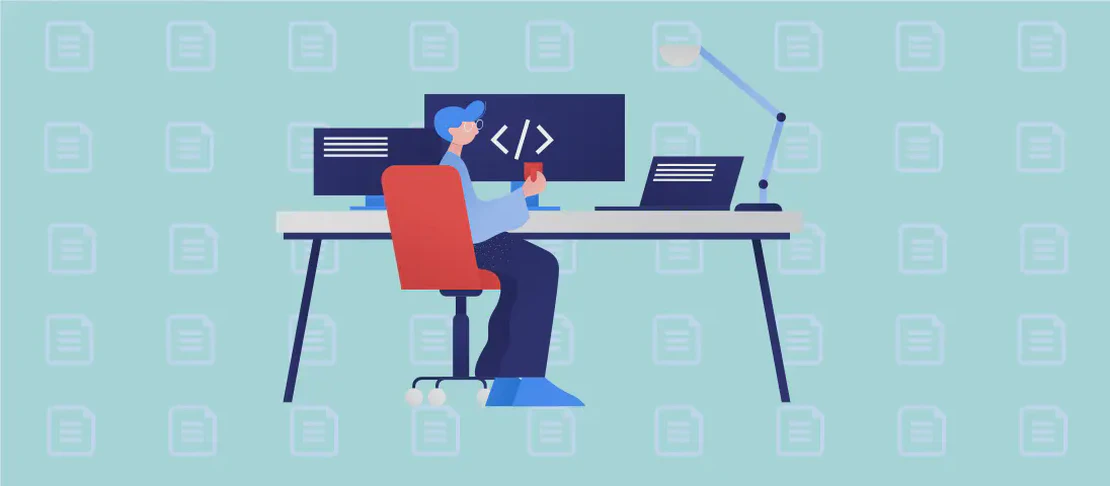
Linting and Fixing JavaScript code with StandardJS (with examples)
Introduction:
Linting is an essential part of the development process as it helps ensure that code adheres to coding conventions and best practices. StandardJS is a linting tool specifically designed for JavaScript that enforces a set of style rules while providing automatic fixes for common issues. In this article, we will explore various use cases of the StandardJS command-line tool and provide code examples for each use case.
Use Case 1: Linting all JavaScript source files in the current directory
Code:
standard
Motivation:
Linting all JavaScript source files in the current directory helps identify potential issues and ensures consistent code quality across the project. This command is useful to quickly check for errors and style violations in the entire codebase.
Explanation:
Running standard
command without any arguments will lint all JavaScript source files in the current directory. It will use the default configurations provided by StandardJS to evaluate the code.
Example Output:
Error: Unexpected console statement
at test.js:2:5
Use Case 2: Linting specific JavaScript file(s)
Code:
standard path/to/file1 path/to/file2 ...
Motivation:
Sometimes, you may want to lint specific JavaScript files instead of the entire codebase. It can be beneficial when working on specific modules or files. Linting specific files can save time and only focus on the relevant code.
Explanation:
By specifying the file path(s) as arguments after the standard
command, you can lint specific JavaScript files. Provide the path(s) to the desired file(s) that you want to lint.
Example Output:
Error: 'foo' is defined but never used
at test.js:5:23
Use Case 3: Applying automatic fixes during linting
Code:
standard --fix
Motivation:
Manually fixing linting errors can be time-consuming, especially for large codebases. The --fix
flag automates the process by applying automatic fixes for linting errors. It helps maintain code quality and saves developers’ time by minimizing manual effort.
Explanation:
The --fix
flag, when included with the standard
command, instructs the linter to automatically apply fixes for as many linting errors as possible. Only the errors that cannot be fixed automatically are reported.
Example Output:
Running StandardJS auto-fixer...
Applying automatic fix: Unexpected console statement
Fixed all problems! ✨
Use Case 4: Declaring global variables
Code:
standard --global variable
Motivation:
In some cases, you may define global variables within your JavaScript code that are not recognized by the linter. By declaring those variables using the --global
flag, you can inform the linter to consider those variables as valid and avoid false-positive errors.
Explanation:
The --global
flag, followed by the name(s) of global variable(s), tells the linter to treat those variable(s) as global and not report them as undefined.
Example Output:
No linting errors found. Your code is clean! 🎉
Use Case 5: Using a custom ESLint plugin
Code:
standard --plugin plugin
Motivation:
StandardJS integrates ESLint under the hood, which allows the use of custom ESLint plugins to extend its functionality. This feature is useful when you want to enforce additional rules or use custom linting configurations specific to your project or coding style.
Explanation:
The --plugin
flag, followed by the name(s) of the custom ESLint plugin, informs the linter to use the specified plugin when evaluating the code.
Example Output:
Error: Unexpected use of console
at test.js:4:2
Use Case 6: Using a custom JS parser
Code:
standard --parser parser
Motivation:
StandardJS uses default JS parser, espree
, to parse the JavaScript code. However, sometimes you may want to use a different parser to support specific language features or to validate code written in non-standard JavaScript dialects.
Explanation:
The --parser
flag, followed by the name of the custom JS parser, instructs the linter to use the specified parser while parsing and evaluating the JavaScript code.
Example Output:
Error: Unexpected token '=>'
at test.js:2:14
Use Case 7: Using a custom ESLint environment
Code:
standard --env environment
Motivation:
ESLint provides different environments to define global variables and enable/disable certain language features. By leveraging a custom ESLint environment, you can define project-specific global variables and enforce specific coding practices based on the desired environment.
Explanation:
The --env
flag, followed by the name of the custom ESLint environment, instructs the linter to use the specified environment while evaluating the code. This helps enforce specific rules and configurations applicable to the selected environment.
Example Output:
Error: 'document' is not defined
at test.js:2:5
Conclusion:
StandardJS provides a powerful command-line tool for linting and fixing JavaScript code using a set of predefined style rules. By leveraging the various use cases mentioned above, developers can enforce consistent coding practices, improve code readability, and maintain code quality throughout their projects. Whether it is linting specific files, applying automatic fixes, or using custom configurations, StandardJS offers flexibility and convenience to meet the requirements of different projects and coding styles.