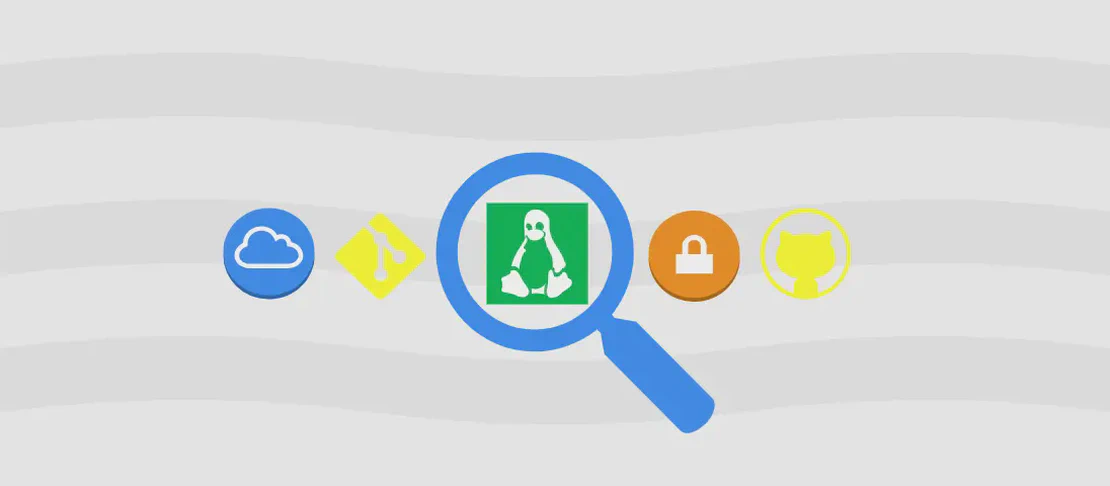
The Power of 'svgr': Transforming SVGs into React Components (with examples)
SVGR is a widely used command-line tool that allows developers to transform SVG files into React components efficiently. This tool is instrumental for front-end developers who work with React applications and need a seamless way to integrate vector graphics directly into their projects. SVGs are preferred for their scalability and performance, making SVGR a valuable tool for converting these SVGs into components that can be easily reused and styled within React applications.
Transform an SVG file into a React component to stdout
Code:
svgr -- path/to/file.svg
Motivation:
The conversion of SVG files directly into React components is a common necessity when you need to render vector graphics dynamically within a React application, retaining full control over their properties through props. This command facilitates a quick conversion directly in the terminal, displaying the React component code in the standard output, making it easy to inspect or integrate directly.
Explanation:
svgr
: Invokes the SVGR tool.--
: Separates the command from its arguments.path/to/file.svg
: Specifies the path to the SVG file that needs conversion.
Example Output:
The standard output will display a React component resembling the structure of the SVG file, complete with props that allow further customization when used in a React application.
Transform an SVG file into a React component using TypeScript to stdout
Code:
svgr --typescript -- path/to/file.svg
Motivation:
Using TypeScript in transforming SVG files to React components is advantageous for developers who are building React applications with TypeScript. This approach ensures that the generated components include TypeScript typings, aiding in robust type-checking and reducing potential errors in development.
Explanation:
svgr --typescript
: This informs SVGR to compile the SVG into a TypeScript-based React component.--
: This marks the end of the options and the start of the file arguments.path/to/file.svg
: This is the location of the SVG file for conversion.
Example Output:
The converted component output maintains TypeScript type annotations, providing additional safety and intelligence during the development process in environments like VSCode.
Transform an SVG file into a React component using JSX transform to stdout
Code:
svgr --jsx-runtime automatic -- path/to/file.svg
Motivation:
This use case is pertinent when leveraging the new JSX Transform introduced in React 17. By using the automatic JSX runtime, React automatically imports necessary functions like React.createElement
, streamlining the code and potentially reducing bundle sizes.
Explanation:
svgr --jsx-runtime automatic
: Specifies the use of the automatic JSX transformation, reducing the need for explicit React imports.--
: Delimits options from file paths in the command.path/to/file.svg
: Indicates the SVG file path that will be transformed.
Example Output:
The resulting output is a React component that benefits from the new JSX Transform, potentially simplifying imports and optimizing performance in applicable environments.
Transform all SVG files from a directory to React components into a specific directory
Code:
svgr --out-dir path/to/output_directory path/to/input_directory
Motivation:
Batch conversion of SVG files from a directory is essential for streamlining workflows, particularly when a project includes multiple SVGs. By converting all SVGs within a directory, developers can save time and ensure uniformity across their components.
Explanation:
svgr --out-dir path/to/output_directory
: Specifies where to store the output React components.path/to/input_directory
: Denotes the directory containing the SVG files to transform.
Example Output:
The command creates React component files corresponding to each SVG in the specified output directory, ready for integration into any React project.
Transform all SVG files from a directory to React components into a specific directory skipping already transformed files
Code:
svgr --out-dir path/to/output_directory --ignore-existing path/to/input_directory
Motivation:
This functionality is critical for large projects where recompilation could be redundant and time-consuming. By ignoring existing files, the process becomes more efficient by only converting new or changed SVGs.
Explanation:
svgr --out-dir path/to/output_directory
: Designates where transformed files should be placed.--ignore-existing
: Ensures that already transformed components are not overwritten.path/to/input_directory
: Path to the directory with SVG files needing transformation.
Example Output:
Only new SVGs or those that have been updated are converted, preserving existing output files and optimizing the conversion workload.
Transform all SVG files from a directory to React components into a specific directory using a specific case for filenames
Code:
svgr --out-dir path/to/output_directory --filename-case camel|kebab|pascal path/to/input_directory
Motivation:
Naming consistency is crucial for file organization, readability, and developer conventions. By specifying a filename case, developers can ensure that all component files adhere to a preferred naming convention, reducing cognitive load.
Explanation:
svgr --out-dir path/to/output_directory
: Designates the output directory.--filename-case camel|kebab|pascal
: Dictates the naming convention for output files.path/to/input_directory
: Identifies where to find the SVG files.
Example Output:
The SVG files within the input directory are transformed to React components, named consistently in the specified case (e.g., camel case).
Transform all SVG files from a directory to React components into a specific directory without generating an index file
Code:
svgr --out-dir path/to/output_directory --no-index path/to/input_directory
Motivation:
Sometimes, developers may prefer not to generate an index file, especially if they plan to manage imports manually or utilize a different module structure. This command disables automatic index creation, allowing for more flexible project setups.
Explanation:
svgr --out-dir path/to/output_directory
: Specifies where converted components will be saved.--no-index
: Directs the tool not to create an index file.path/to/input_directory
: Identifies the source directory for SVG files.
Example Output:
Each SVG file is converted to a React component, but no index file is generated, leaving the organization of components to the developer’s discretion.
Conclusion:
Using SVGR, developers can seamlessly convert SVG files into React components, optimizing their workflow and ensuring integration of scalable vector graphics into applications. By accommodating various settings such as type usage, JSX transformations, and naming conventions, SVGR proves itself as an indispensable tool for modern React development tasks.