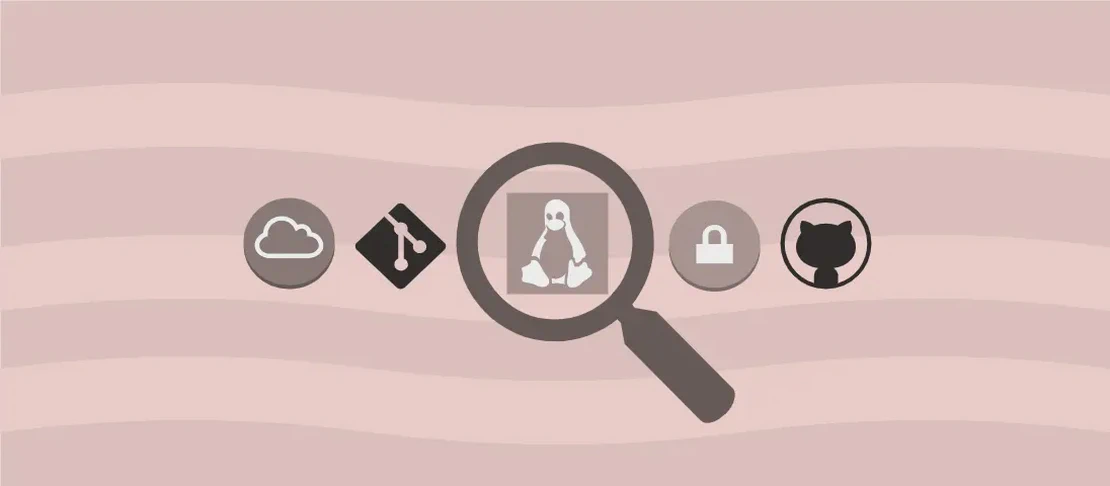
How to Use the Command 'swagger-codegen' (with Examples)
Swagger Codegen is a powerful open-source tool that facilitates the generation of client SDKs, server stubs, API documentation, and other related files from an OpenAPI/Swagger definition. This utility supports various programming languages and frameworks, allowing developers to streamline the process of creating boilerplate code and ensuring consistency across projects. Utilizing Swagger Codegen can significantly shorten development cycles by automating repetitive tasks and promoting a standardized approach to API interactions.
Use Case 1: Generate Documentation and Code from an OpenAPI/Swagger File
Code:
swagger-codegen generate -i swagger_file -l language
Motivation:
Developers often require a systematic way to generate client-side code and documentation from their well-defined API specifications. By leveraging Swagger Codegen, teams can maintain a single source of truth — the OpenAPI/Swagger file — from which they can derive both documentation and code, ensuring alignment and reducing errors.
Explanation:
swagger-codegen generate
: Initiates the generation process.-i swagger_file
: Specifies the input file, which is the OpenAPI/Swagger definition. This file acts as the blueprint for generating the output.-l language
: Sets the target programming language or framework for the generated code. This can be anything supported by Swagger Codegen, such as Java, Python, Go, etc.
Example Output:
Running this command will generate documentation and client libraries in the specified language. The generated files will typically include automatically structured folders containing libraries, configuration classes, and comprehensive markdown or HTML documentation illustrating how to effectively utilize API endpoints. This streamlines the integration process and clearly presents the API functionalities.
Use Case 2: Generate Java Code Using the Library Retrofit2 and the Option useRxJava2
Code:
swagger-codegen generate -i http://petstore.swagger.io/v2/swagger.json -l java --library retrofit2 -DuseRxJava2=true
Motivation:
When developing Android applications or Java-based projects, using Retrofit2 for HTTP client operations in conjunction with RxJava for handling asynchronous event-based programming is a common choice. Combining these libraries provides powerful capabilities for efficient networking operations while handling complex asynchronous data streams.
Explanation:
swagger-codegen generate
: Triggers the code generation process.-i http://petstore.swagger.io/v2/swagger.json
: Defines the source of the API specification, in this case, the public Petstore Swagger JSON document URL.-l java
: Chooses Java as the target programming language for code generation.--library retrofit2
: Specifies that Retrofit2 should be used to generate the HTTP client code.-DuseRxJava2=true
: An additional configuration to enable RxJava2 features in the generated client code, allowing developers to use reactive extensions in their applications.
Example Output:
Executing this command will produce a set of Java files configured with Retrofit2 and RxJava2. The generated code will include service interfaces, data models, and utility classes. These files will be immediately usable in a project to interact with the specified API effectively, promoting best practices for network operations in Java applications.
Use Case 3: List Available Languages
Code:
swagger-codegen langs
Motivation:
Developers need to know the range of supported programming languages and frameworks before utilizing Swagger Codegen for their specific use cases. Listing available languages ensures that the toolset can accommodate the technology stack in use or help make informed decisions about language adoption.
Explanation:
swagger-codegen langs
: This command fetches and displays all the languages and frameworks Swagger Codegen supports for generating code.
Example Output:
Executing this command will return a comprehensive list of all programming languages and frameworks supported by Swagger Codegen. This includes mainstream languages like Java, Python, and Node.js, as well as specialized frameworks and platforms. This information facilitates better planning and resource allocation in development projects.
Use Case 4: Display Help for a Specific Command
Code:
swagger-codegen generate|config-help|meta|langs|version --help
Motivation:
Understanding the available options and configurations for each command is critical. Accessing the help documentation directly from the CLI provides an immediate reference for developers, minimizing the need for external documentation searches and reducing trial and error when troubleshooting or learning new features.
Explanation:
swagger-codegen generate|config-help|meta|langs|version --help
: Displays detailed help information for the specified command. Users can replacegenerate|config-help|meta|langs|version
with their command of interest to get tailored guidance.
Example Output:
Upon executing this command with a specific command option, users receive a list of available flags and options, along with concise explanations for each. This immediate reference helps users understand command usage, potential parameters, and expected outputs, significantly easing the learning curve and improving productivity.
Conclusion:
Swagger Codegen is a versatile and robust tool essential for modern API development workflows. Whether generating client libraries, server stubs, or comprehensive API documentation, it quickly translates API specifications into usable code across multiple languages and frameworks. Understanding and utilizing its features through the examples provided can dramatically improve development efficiency and help maintain consistency across API-driven projects.