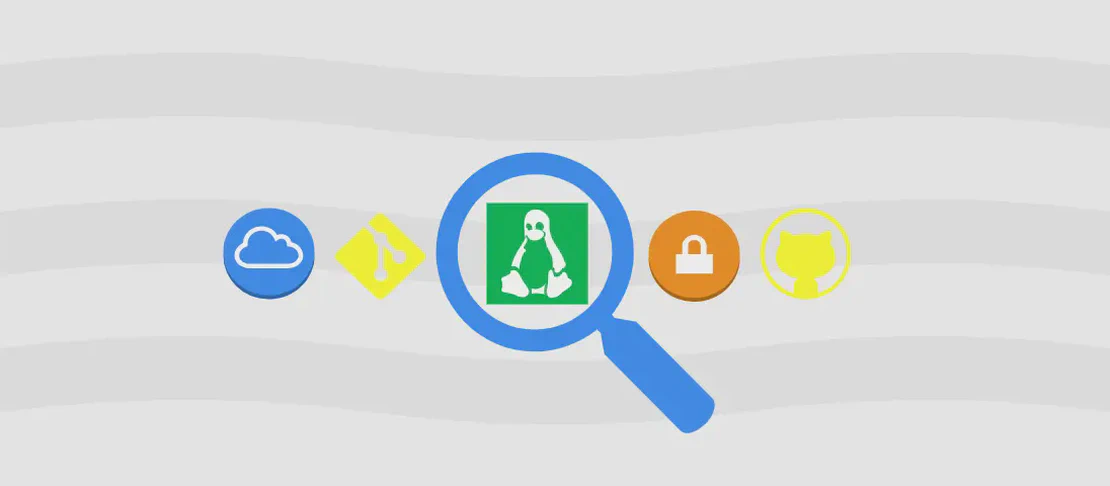
How to Use the Command 'swc' (with examples)
The swc
command is a JavaScript and TypeScript compiler written in Rust, known for its exceptional speed and performance. It is designed to transcompile complex JavaScript and TypeScript codebases into readable and efficient JavaScript code. This ongoing need is fueled by the evolving standards and syntaxes of JavaScript, paired with the adoption of TypeScript in modern web development. By leveraging the high performance of Rust, swc
provides a robust solution for developers who need to manage large codebases efficiently.
Use case 1: Transpile a specified input file and output to stdout
Code:
swc path/to/file
Motivation:
This use case is great for quickly examining how the code is transformed. It offers a way to directly see the output without needing to create additional files or directories. A developer might typically use this during debugging or while learning to understand how swc
processes specific syntax constructs.
Explanation:
swc
: The command line interface for running theswc
compiler.path/to/file
: The file path indicates the input JavaScript or TypeScript file which needs to be transpiled.
Example Output:
When you run the above command, it will display the transpiled code directly in the terminal, allowing quick verification of the transformation process.
Use case 2: Transpile the input file every time it is changed
Code:
swc path/to/file --watch
Motivation:
This scenario is particularly useful during development. When a file is frequently changed, having the ability to automatically transpile it saves time and reduces errors. By using the watch mode, developers can maintain focus on coding without manually retriggering the transpilation process.
Explanation:
swc
: The command to invoke the compiler.path/to/file
: The input file that is being monitored.--watch
: This flag makesswc
watch the specified file for changes, automatically re-running transpilation upon detected modifications.
Example Output:
Upon modification of the file being watched, the terminal will again output the transpiled code, reflecting the latest changes immediately.
Use case 3: Transpile a specified input file and output to a specific file
Code:
swc path/to/input_file --out-file path/to/output_file
Motivation:
Directing the transpiled code to a specific file is beneficial for integrating into build systems or when preparing files for production. By clearly specifying output files, developers can organize their codebase more effectively, ensuring clean and maintainable directory structure.
Explanation:
swc
: The command to run the compiler.path/to/input_file
: The input file you want to transpile.--out-file path/to/output_file
: This option designates the file where the transpiled code should be written.
Example Output:
Running this command will not produce terminal output, but instead, it will save the transpiled code to the specified output file, making it easy to use in other production processes.
Use case 4: Transpile a specified input directory and output to a specific directory
Code:
swc path/to/input_directory --out-dir path/to/output_directory
Motivation:
Transpiling all files in a directory is essential for large projects with multiple modules. This use case allows an efficient way to handle the compilation of numerous files, providing scalability and organization for complex codebases, especially useful in CI/CD pipelines.
Explanation:
swc
: Used to initiate the compiler.path/to/input_directory
: The directory containing source files you want to compile.--out-dir path/to/output_directory
: This specifies the destination directory for all transpiled files.
Example Output:
The command will compile all supported files within the input directory and place their transpiled versions within the specified output directory, preserving the directory structure.
Use case 5: Transpile a specified input directory using a specific configuration file
Code:
swc path/to/input_directory --config-file path/to/.swcrc
Motivation:
Utilizing a configuration file empowers developers with custom settings to fine-tune the transpilation process. This is beneficial when needing consistent builds or specific settings across various environments, ensuring compatibility and consistency.
Explanation:
swc
: The command to run the compiler.path/to/input_directory
: The directory with files to be transpiled.--config-file path/to/.swcrc
: Indicates the configuration fileswc
should use, allowing for customized settings including target environments and module specifications.
Example Output:
Files in the input directory are transpiled according to the instructions in the configuration file, ensuring the output meets specified development or production requirements.
Use case 6: Ignore files in a directory specified using glob path
Code:
swc path/to/input_directory --ignore path/to/ignored_file1 path/to/ignored_file2 ...
Motivation:
In some projects, certain files may not need to be transpiled, such as those already optimized, external libraries, or simply files not included in the upcoming build. By specifying files to ignore, developers enhance build efficiency and maintain focus on only necessary assets.
Explanation:
swc
: The command employed to start the transpiler.path/to/input_directory
: Designates the directory to transpile.--ignore path/to/ignored_file1 path/to/ignored_file2 ...
: Lists the files within the directory to exclude during the transpilation process, improving performance by skipping unnecessary files.
Example Output:
Running this command will transpile only the specified files while ignoring those marked by the --ignore
option, producing an output devoid of any unwanted files.
Conclusion:
The swc
command offers versatile, high-performance options for developers working with JavaScript and TypeScript, ensuring rapid development cycles and efficient codebase management. From its basic commands outputting directly to terminal, to sophisticated directory-wide transpilation with custom configurations, swc
provides a strong toolkit for modern development needs.