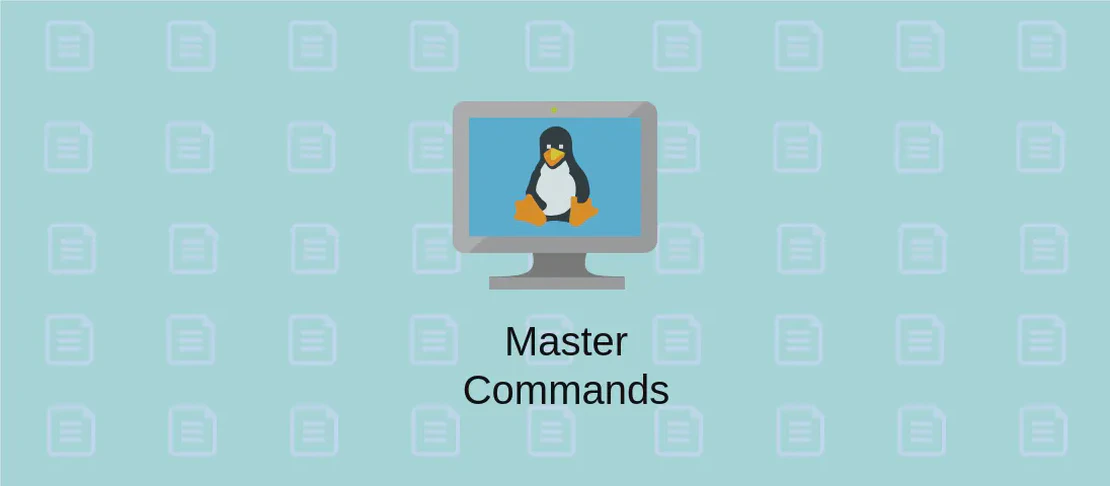
How to Use the Swift Command (with Examples)
Swift is a powerful and intuitive programming language developed by Apple for building apps on iOS, macOS, watchOS, and tvOS, and even for cloud and server-side programming. It is designed to provide a robust, safe, and expressive means of development, allowing developers to create compelling and interactive apps by leveraging concise syntax and modern language technology. The Swift command-line interface (CLI) provides an environment to experiment with code, manage projects, handle dependencies, and facilitate other essential tasks related to Swift projects.
Start a REPL (interactive shell)
Code:
swift repl
Motivation: Swift’s REPL (Read-Eval-Print Loop) is an interactive programming environment that evaluates inputs, executes code, and returns results immediately. It’s crucial for testing out snippets of code, experimenting with different programming ideas, or learning Swift without needing to create a full-fledged project. This interactive shell accelerates learning and enables rapid prototyping by allowing developers to see the outputs of their code instantaneously.
Explanation: In the command above, swift
indicates the Swift CLI, and repl
is the argument that tells the interface to launch the Swift interactive shell. This tool is a ground staple for Swift education, testing, and debugging.
Example output:
Welcome to Swift version x.x.x (swift-x.x-xxx.xx.x).
Type :help for assistance.
1>
Execute a program
Code:
swift file.swift
Motivation: Executing a Swift program with ease is a fundamental requirement for developers. By running this command, developers can quickly test and verify the functionality of a Swift script or piece of code outlined in a file, rather than setting up an entire project.
Explanation: Here, swift
calls the Swift command-line tool to execute the script specified. The file.swift
represents a file containing Swift code that has been written by the developer. Upon execution, the CLI reads and runs the code within that file, providing results or any error messages.
Example output:
Hello, World!
Start a new project with the package manager
Code:
swift package init
Motivation: Setting up a complete Swift package project is streamlined through this command. It quickly scaffolds the directories and files needed for a new project, following standard conventions. This is immensely beneficial as it reduces manual setup time and ensures that a consistent structure is maintained across Swift projects.
Explanation: The command swift package init
uses swift
to initiate the package management tool and package init
to trigger the creation of a new package structure. This includes creating essential files and directories that form a working skeleton of a Swift project, such as Package.swift
and source folders.
Example output:
Creating library package: MyPackage
Creating Package.swift
Creating README.md
Creating .gitignore
Creating Sources/
Creating Sources/MyPackage/MyPackage.swift
Creating Tests/
Creating Tests/LinuxMain.swift
Creating Tests/MyPackageTests/
Creating Tests/MyPackageTests/MyPackageTests.swift
Generate an Xcode project file
Code:
swift package generate-xcodeproj
Motivation: Xcode is one of the most widely used IDEs for Swift development on macOS. Generating an Xcode project file from a Swift package enables developers to leverage Xcode’s extensive debugging tools, UI design features, and resource management within a Swift package. This is crucial for integrating package projects into larger, more intricate app structures.
Explanation: The command swift package generate-xcodeproj
starts with swift
to initiate the CLI, package
refers to actions related to package management, and generate-xcodeproj
specifics the creation of an Xcode project file from the existing Swift package. This bridges the gap between command-line enhancements and sophisticated IDE capabilities.
Example output:
generated: ./MyPackage.xcodeproj
Update dependencies
Code:
swift package update
Motivation: Keeping package dependencies up-to-date is vital for maintaining the security and functionality of a project. This command checks for the latest versions of dependencies specified in the Package.swift
file and downloads updates, ensuring that the project remains aligned with the latest improvements and security patches.
Explanation: When you run swift package update
, swift
initiates the Swift CLI, and then package
and update
work together to fetch and apply updates to the dependencies defined in the project’s package file. This is an essential maintenance task that optimizes package stability and performance.
Example output:
Updating https://github.com/apple/example-package-fisheryates
Updated:
https://github.com/apple/example-package-fisheryates (from v1.0.0 to v1.1.0)
Compile project for release
Code:
swift build -c release
Motivation: Compiling a project for release is a critical step in the development lifecycle, transforming the code into a final product that end-users can interact with. The release configuration ensures the application is optimized for performance with additional compiler optimizations applied, delivering a fast, efficient, and refined software product.
Explanation: The swift build -c release
command begins with swift
to access the CLI, build
to start the project compiling process, and -c release
to specify that the build should use the release configuration. This setup applies optimizations, reducing the application’s size and improving execution performance compared to a debug build.
Example output:
Building for release...
Compile Swift Module 'MyPackage' (2 sources)
Linking ./.build/release/MyPackage
Conclusion
In summary, the Swift command-line interface is an indispensable toolset for Swift developers, providing essential functionalities from developing and testing Swift code to managing and compiling projects. Whether you are a beginner learning coding concepts or an experienced developer working on sophisticated project structures, mastering these commands can significantly streamline and enhance your Swift programming experience.