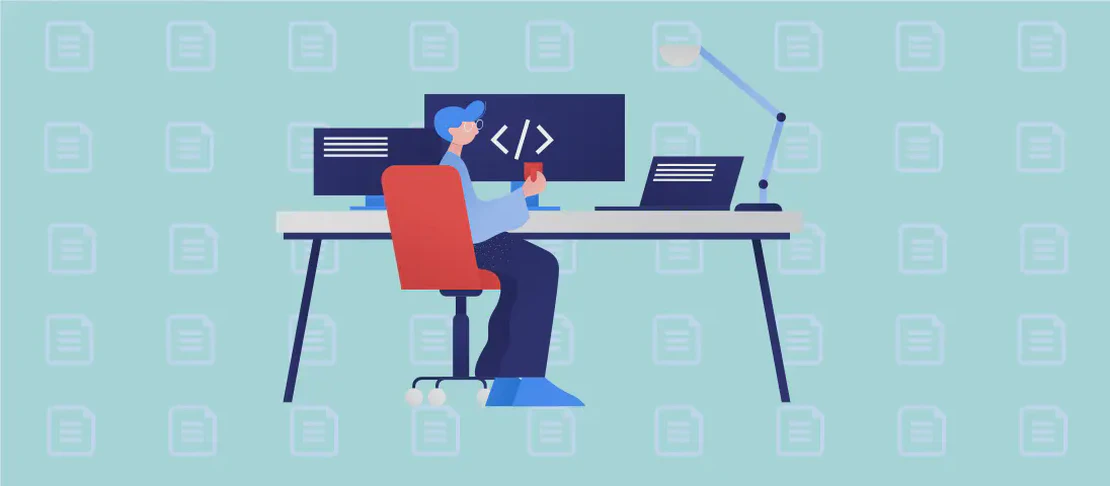
How to use the command 'swig' (with examples)
SWIG (Simplified Wrapper and Interface Generator) is a powerful tool that connects programs written in C or C++ with various high-level programming languages. By using interface files with SWIG directives, it automates the creation of the wrapper code required to build extension modules for languages such as Python, Java, Go, and Ruby. This allows developers to utilize existing C/C++ libraries in a more accessible and often more intuitive high-level language, speeding up development time and enhancing the use of legacy code.
Use case 1: Generate a binding between C++ and Python
Code:
swig -c++ -python -o path/to/output_wrapper.cpp path/to/swig_file.i
Motivation:
Generating a binding between C++ and Python is immensely useful when you have a C++ library that implements complex algorithms or performs at a higher performance level and you want to expose this functionality to Python. Python is renowned for its simplicity and vast ecosystem of libraries, and code written in Python is usually more concise and easier to read. By creating bindings, developers can take the advantage of C++’s performance and Python’s ease of use.
Explanation:
-c++
: This argument tells SWIG that the source code is in C++.-python
: This flag indicates that the target language for the wrapper is Python, instructing SWIG to produce Python bindings.-o path/to/output_wrapper.cpp
: This specifies the output file that will contain the generated wrapper code. The fileoutput_wrapper.cpp
will include glue code that translates Python function calls into C++ library function calls, allowing seamless interoperability between the languages.path/to/swig_file.i
: This is the SWIG interface file that contains directives for how the C++ code should be exposed to Python. The interface file typically includes C++ header files and defines what functions are accessible in Python.
Example Output:
After running the above command, you will get an output_wrapper.cpp
file, which serves as the bridge between your C++ library and Python. Typically, you will then compile this file into a shared library using a C++ compiler, which can then be imported and used in Python as a module.
Use case 2: Generate a binding between C++ and Go
Code:
swig -go -cgo -intgosize 64 -c++ path/to/swig_file.i
Motivation:
Go is a statically typed, efficient, and easy-to-use language that is well-suited for concurrent programming, which might be lacking in C++. By creating bindings, developers can leverage C++ libraries within Go programs, thus enabling Go applications to manage parallel workloads or otherwise incorporate C++’s strengths where they are most needed.
Explanation:
-go
: This switch signals that the target language for binding is Go, and SWIG will generate Go wrapper code.-cgo
: It instructs SWIG to enable cgo mode, which is necessary for interfacing C (and C++) code with Go. Cgo acts as a bridge, allowing Go programs to call C/C++ codes efficiently.-intgosize 64
: This specifies the size of Go’sint
type in bits. The argument is critical because it affects the data types in the binding, ensuring data consistency between C++ and Go, especially on 64-bit architectures.-c++
: It indicates that the source code is in C++.path/to/swig_file.i
: The SWIG interface file outlining which parts of the C++ code should be made available to Go.
Example Output:
This command generates Go wrapper code that, together with Go’s cgo tool, allows Go programs to call functions in the C++ code seamlessly. This results in a Go file which can then be compiled along with the C++ source to create a Go module.
Use case 3: Generate a binding between C and Java
Code:
swig -java path/to/swig_file.i
Motivation:
Java is one of the most popular programming languages worldwide due to its portability across various platforms thanks to the Java Virtual Machine (JVM). Running C libraries within a Java application is beneficial when you need to leverage an existing C codebase with minimal performance overhead while maintaining platform independence.
Explanation:
-java
: This argument specifies that SWIG should generate bindings for Java. SWIG will create the necessary Java classes and native JNI (Java Native Interface) code to allow direct interaction between Java and the underlying C code.path/to/swig_file.i
: The SWIG interface file that serves as the blueprint for what parts of the C code should be accessible from Java. This file often includes C headers and SWIG-specific instructions.
Example Output:
Using this command, SWIG produces Java classes and header files for JNI. The generated code will handle Java’s interaction with C, enabling functions defined in C to be directly used in Java applications, often resulting in quicker operations as compared to pure Java solutions.
Use case 4: Generate a binding between C and Ruby and prefix the Ruby module with foo::bar::
Code:
swig -ruby -prefix "foo::bar::" path/to/swig_file.i
Motivation:
Ruby is known for its elegant syntax and is widely favored among web developers due to frameworks like Ruby on Rails. Sometimes, it is necessary to harness C libraries in a Ruby application to enhance performance or to incorporate functionality that would be cumbersome to recreate in Ruby. Adding a prefix to the module can help in organizing and namespacing the Ruby components to avoid conflicts and make the API more readable.
Explanation:
-ruby
: This flag indicates the target language is Ruby, for which SWIG should generate bindings.-prefix "foo::bar::"
: The-prefix
option adds a specified prefix to all Ruby classes and modules generated by SWIG. This is particularly useful to prevent naming collisions and to maintain a clean, organized namespace when integrating C libraries into larger Ruby projects.path/to/swig_file.i
: This file is the SWIG interface file setting the guidelines for which parts of the C code are exposed to Ruby.
Example Output:
The command will produce Ruby extension code that, when compiled, results in a Ruby module prefixed with foo::bar::
. This structured namespace aids in maintaining clean integration between Ruby and C libraries, allowing for a seamless and organized development approach.
Conclusion:
SWIG simplifies the creation of interfaces between complex C/C++ libraries and high-level programming languages across various platforms. It generates necessary wrapper code, allowing developers to utilize performant C/C++ code within the ecosystem of other languages like Python, Go, Java, and Ruby. By automating the binding process, SWIG reduces manual coding work, helps in leveraging existing robust codebases, and therefore optimizes both the development process and the overall application performance across different computing environments.