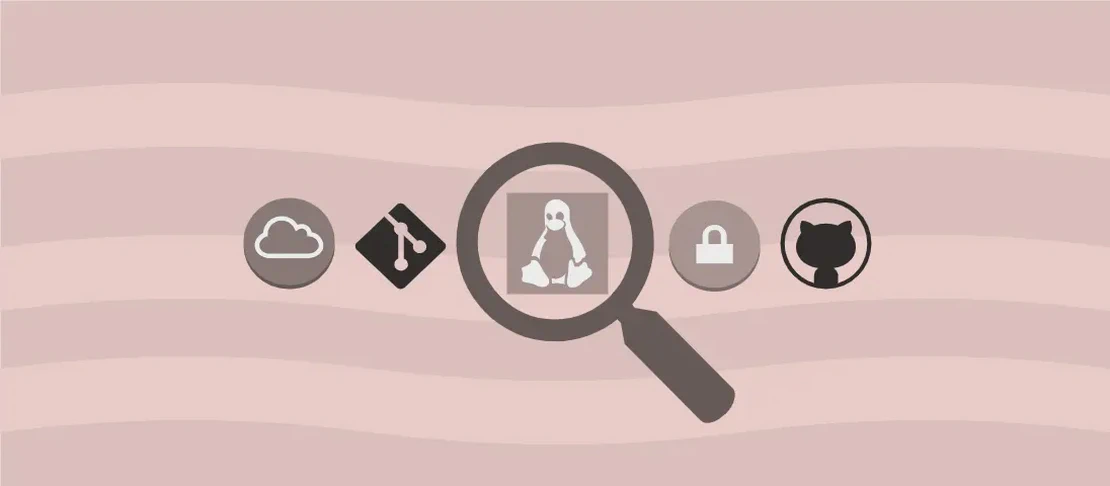
How to Use the Command 'tar' (with Examples)
The tar
command is a versatile archiving utility widely used in Unix and Unix-like operating systems. It stands as a cornerstone utility for file archiving and distribution. While the main purpose of tar
is to collect multiple files into a single archive file, it is often used in conjunction with compression algorithms like gzip
or bzip2
to reduce the archive’s size. This makes tar
indispensable for backup, storage, or transferring collections of files over a network.
Use Case 1: Create an Archive and Write It to a File
Code:
tar cf path/to/target.tar path/to/file1 path/to/file2 ...
Motivation:
Users often find themselves needing to consolidate multiple files into a single package for storage or transfer purposes. Creating a tar
archive simplifies this process by bundling files together.
Explanation:
tar
: The command to invoke the tar utility.c
: Stands for “create,” instructing tar to create a new archive.f
: Specifies that the next argument will be the name of the archive file.path/to/target.tar
: Destination path and filename for the resulting archive.path/to/file1 path/to/file2 ...
: The source files that are to be included in the archive.
Example Output:
There is no output when the tar
command is successfully run unless there is an error. The output is the target.tar
file in the specified directory.
Use Case 2: Create a Gzipped Archive and Write It to a File
Code:
tar czf path/to/target.tar.gz path/to/file1 path/to/file2 ...
Motivation:
Compression saves storage space and bandwidth, which is vital when transferring files over a network. Using gzip
for compression adds convenience and efficiency.
Explanation:
tar
: Initiates the tar command.c
: Instructs to create a new archive.z
: Compresses the archive withgzip
.f
: Denotes that the following argument is the filename of the archive.path/to/target.tar.gz
: Specifies the destination path and filename of the compressed archive.path/to/file1 path/to/file2 ...
: Lists the files to be archived and compressed.
Example Output:
The output is a compressed .tar.gz
file at the specified path, with no additional terminal output unless errors occur.
Use Case 3: Create a Gzipped Archive from a Directory Using Relative Paths
Code:
tar czf path/to/target.tar.gz --directory=path/to/directory .
Motivation: When archiving an entire directory, users often prefer to retain the directory structure in a relative manner within the archive for organizational purposes.
Explanation:
tar
: Calls the tar utility.c
: Signals to create an archive.z
: Appliesgzip
compression.f
: Indicates the following argument is the output file.path/to/target.tar.gz
: Specifies where the compressed archive will be saved.--directory=path/to/directory
: Changes to the specified directory before processing..
: Denotes the current directory, implying all files and subdirectories will be included.
Example Output:
A new archive, target.tar.gz
, that contains the entire directory structure as it was when the command was run.
Use Case 4: Extract a Compressed Archive File into the Current Directory Verbosely
Code:
tar xvf path/to/source.tar[.gz|.bz2|.xz]
Motivation: Data recovery or file access demands that archived files be extracted. Verbose mode provides detailed feedback on the unpacking process, beneficial for ensuring all files are correctly extracted.
Explanation:
tar
: Utilizes the tar tool.x
: Represents “extract,” instructing tar to unpack the archive.v
: Engages verbose mode, yielding detailed output about the files being processed.f
: Indicates that the following parameter is the archive file.path/to/source.tar[.gz|.bz2|.xz]
: Specifies the archive file to extract, supporting several compression formats.
Example Output: Terminal output listing each file contained within the archive as they are extracted.
Use Case 5: Extract a Compressed Archive File into the Target Directory
Code:
tar xf path/to/source.tar[.gz|.bz2|.xz] --directory=path/to/directory
Motivation: Situations often arise where extracted files need to be placed directly into a specific directory, facilitating organized data management and workflow integration.
Explanation:
tar
: Deploys the tar program.x
: Commands tar to extract files.f
: Designates the following string as the archive file name.path/to/source.tar[.gz|.bz2|.xz]
: Input archive file to be extracted.--directory=path/to/directory
: Guides tar to a specific directory for file extraction.
Example Output: Files from the specified archive are extracted into the targeted directory with no verbose output unless errors are reported.
Use Case 6: Create a Compressed Archive and Write It to a File Using Automatic Compression Program
Code:
tar caf path/to/target.tar.xz path/to/file1 path/to/file2 ...
Motivation: This command simplifies the process of choosing a compression method by automatically selecting one based on the file extension, thus optimizing file handling processes.
Explanation:
tar
: Engages the tar command.c
: Signifies the creation of a new archive.a
: Automatically selects the compression program based on the file extension.f
: Confirms the next string as the archive file name.path/to/target.tar.xz
: Designates the output archive file with.xz
suggesting high compression.path/to/file1 path/to/file2 ...
: Specifies the input files for archiving.
Example Output:
The resulting target.tar.xz
file is stored in the specified location.
Use Case 7: List the Contents of a Tar File Verbosely
Code:
tar tvf path/to/source.tar
Motivation: Before extraction, users may want to verify the contents of an archive. This listing helps in understanding what files would be extracted without actually extracting them.
Explanation:
tar
: Calls the tar utility.t
: Triggers the list action to display archive contents.v
: Activates verbose output for comprehensive file details.f
: Signifies the archive filename follows.path/to/source.tar
: Designates the archive file to list.
Example Output:
A detailed listing of all files in source.tar
with information like file sizes, modification times, and names.
Use Case 8: Extract Files Matching a Pattern from an Archive File
Code:
tar xf path/to/source.tar --wildcards "*.html"
Motivation: Selective extraction provides efficiency by allowing users to pull only necessary files, such as HTML files from an archive containing mixed file types.
Explanation:
tar
: Invokes the tar utility.x
: Instructs tar to extract files.f
: Confirms the forthcoming argument is the file name.path/to/source.tar
: Name of the archive from which to extract files.--wildcards "*.html"
: Matches files with the.html
extension for extraction.
Example Output: All HTML files from the specified archive are extracted to the current directory.
Conclusion:
The various functionalities of tar
, along with the added benefits of compression tools like gzip
and bzip2
, make this command indispensable for tasks involving archiving, compressing, and extracting files efficiently. Understanding these diverse use cases enriches users’ ability to manage file systems adeptly and ensures the seamless handling of significant data storage and transfer tasks. Whether for backup solutions, organizational tasks, or bandwidth-conscious transfers, the tar
command holds an essential place in the toolkit of anyone working in Unix-based environments.