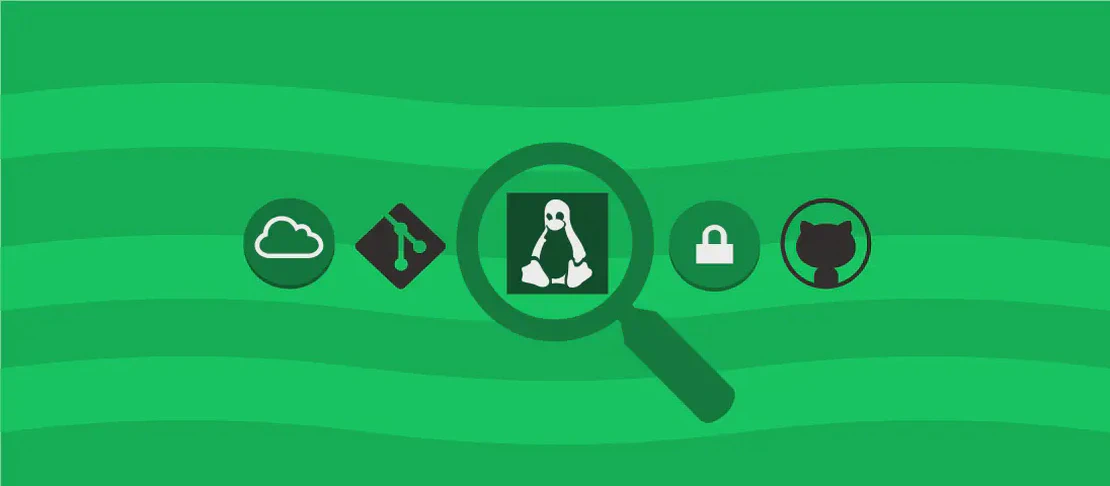
How to Use the Command 'tcc' (with Examples)
The Tiny C Compiler (tcc
) is a lightweight and incredibly fast compiler for C language, designed to perform not only compilation but also linking of the source code. Its standout feature is its ability to execute C source files like scripts directly from the command line, without the need for a separate compilation step. This simplicity and speed make it an attractive tool for developers who want rapid iteration and testing. Along with these features, tcc
offers a set of command-line options that are similar to the more widely known GNU Compiler Collection (gcc
).
Use case 1: Compile and Link 2 Source Files to Generate an Executable
Code:
tcc -o executable_name path/to/file1.c path/to/file2.c
Motivation:
When working with C projects that include multiple source files, quickly compiling and linking them into a single executable is essential. This is where the tcc
command shines—it allows you to compile and link multiple files in one simple command. By doing so, it allows a developer to produce a standalone executable ready for execution almost instantaneously, which is particularly useful during the development phase when frequent testing and updates are required.
Explanation:
tcc
: This is the command to invoke the Tiny C Compiler.-o executable_name
: This option specifies the name of the output file. Here,tcc
is directed to create an executable file with the nameexecutable_name
.path/to/file1.c path/to/file2.c
: These are the paths to the C source files that you want to compile and link.tcc
takes these files and processes them according to the provided options.
Example Output:
After executing the command, you should have an executable file named executable_name
in your current directory. Running this executable will produce the output programmed in your C source code, such as printing messages to the console or performing calculations.
Use case 2: Directly Run an Input File Like a Script and Pass Arguments to It
Code:
tcc -run path/to/source_file.c arguments
Motivation:
Sometimes, the overhead of constant compilation can interrupt the workflow, especially during the testing phase of development. If you’re iterating on a command-line tool or a small script written in C, using tcc
to run the file directly can save time and streamline the process. This functionality allows developers to use C code as if it were a script, facilitating rapid feedback and debugging.
Explanation:
tcc
: This invokes the Tiny C Compiler.-run
: This option tellstcc
to compile and execute the C source file immediately, without creating an intermediate binary output file for execution.path/to/source_file.c
: This specifies the path to the C source file that you wish to execute.arguments
: These are the arguments that you pass to the C program, much like the arguments passed to a command-line script. They are directly available in your C program via theargc
andargv
variables inmain()
.
Example Output:
When you execute this command, the output will be directly generated as per the logic in your C program. For example, if your C script were designed to echo its arguments, you would see each argument listed one after another in the console.
Use case 3: Interpret C Source Files with a Shebang Inside the File
Code in the beginning of your C file:
#!/full/path/to/tcc -run
Motivation:
The use of a shebang (#!
) at the beginning of a file is a standard method in Unix-like operating systems to indicate which interpreter should be used to execute the file. For developers who wish to combine the powerful language features of C with the convenience of scripting, integrating a shebang with tcc
offers a seamless, script-like execution model. This is exceptionally practical in cases where a program needs to be shared and used like a script but benefits from being written in C for performance reasons.
Explanation:
#!
: Known as a shebang, this tells the operating system to consider this script as executable and identifies which interpreter should be used./full/path/to/tcc -run
: This is the full path to thetcc
executable with the-run
option, which is used to compile and run the script. The path should point to the location wheretcc
is installed on your system.
Example Output:
After giving the source file execution permissions and running it, the output will be whatever is specified in your C program. For example, if your code included a printf
statement that outputs “Hello, World!”, running the file would display “Hello, World!” in the terminal.
Conclusion:
The tcc
utility provides an efficient and straightforward toolset for C developers, offering a variety of powerful command-line options to compile, link, and run C programs. Its speed and ability to execute C code directly as scripts make it an indispensable tool for rapid development and testing, offering several use cases that significantly enhance coding and development workflows in C.