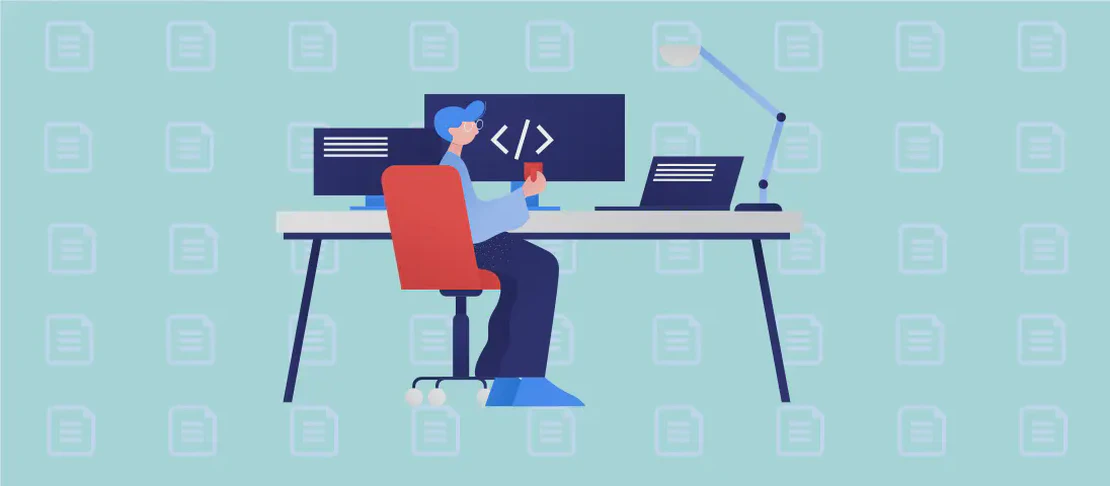
How to use the command 'tee' (with examples)
The tee
command in Unix-like systems is an exceptionally versatile tool that allows users to read from standard input (stdin
) and write to both standard output (stdout
) and one or more files simultaneously. This command is particularly useful in pipelines, enabling the user to display outputs on the terminal while simultaneously saving it to a file or further processing it through additional commands. The functionality of tee
makes it an indispensable tool for anyone looking to manipulate data streams efficiently.
Use case 1: Copy input to file and terminal
Code:
echo "example" | tee path/to/file
Motivation:
This use case is useful when you want to monitor the output on the terminal while also saving it directly to a file for future reference. By using tee
, you can avoid running the command twice and save time and processing power.
Explanation:
echo "example"
: This command generates the string “example” and sends it tostdout
.|
: This pipe operator directs the output of the preceding command into the input of thetee
command.tee path/to/file
: Thetee
command receives the input from theecho
command and writes it to “path/to/file”. Simultaneously, it also displays the input on the terminal (stdout
).
Example output:
example
Where the word “example” is both printed to the terminal and saved in the specified file.
Use case 2: Append input to an existing file
Code:
echo "example" | tee -a path/to/file
Motivation:
In many situations, it’s crucial to add content to the end of a file without overwriting the existing data. For instance, when logging data, appending provides a seamless record of activities without losing historical information.
Explanation:
echo "example"
: Produces the input “example”.|
: Sends the output totee
.tee -a path/to/file
: The-a
flag tellstee
to append the input to the file, rather than overwriting it. As before, it also prints tostdout
.
Example output:
example
The word “example” is shown on the terminal and appended to the file.
Use case 3: Pipe output to another program
Code:
echo "example" | tee /dev/tty | xargs printf "[%s]"
Motivation:
Sometimes it’s necessary to process data further while also visualizing it on the terminal. This pipeline enables such flexibility by displaying output, then formatting it through another command like xargs
.
Explanation:
echo "example"
: Generates “example”.|
: Directs the data flow into thetee
command.tee /dev/tty
: Writes the input to the terminal (/dev/tty
) and also passes it down the line.| xargs printf "[%s]"
: Takes the input fromtee
, usesxargs
to format it, and wraps it in square brackets withprintf
.
Example output:
example
[example]
Where “example” initially appears on the terminal, followed by its formatted version.
Use case 4: Create a directory and character count
Code:
echo "example" | tee >(xargs mkdir) >(wc -c)
Motivation:
This command showcases the power of process substitution, where input can be handled by multiple subshells simultaneously. Here, it creates a directory named after the input string and counts the characters in that string.
Explanation:
echo "example"
: Creates the input “example”.|
: Directs theecho
output intotee
.tee >(xargs mkdir) >(wc -c)
: Uses process substitution>(...)
to send the input to commands in separate shells.xargs mkdir
uses the input to make a directory,wc -c
counts characters in the input and displays the count.
Example output:
7
The number “7” represents the character count, while a directory named “example” is created.
Conclusion:
The tee
command is a versatile and powerful utility for handling input and output simultaneously across Unix-like systems. Its ability to duplicate its input, display it, and send it for further processing or storage makes it an indispensable tool for efficient command-line operations. With the examples above, users can understand and leverage the capabilities of tee
to enhance their workflow and data management skills.