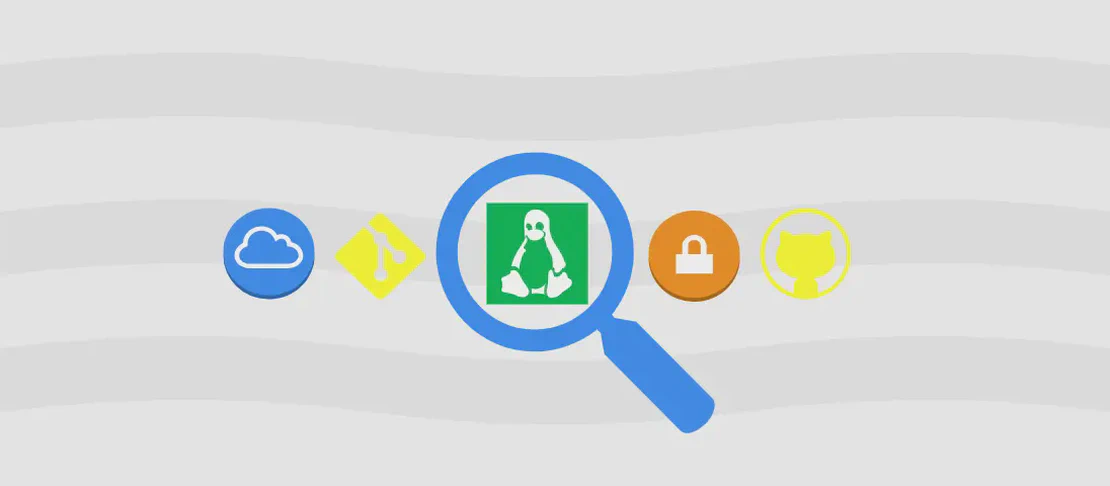
Using the PowerShell Command 'Tee-Object' (with examples)
The Tee-Object
command in PowerShell is a versatile tool designed to handle output streams effectively. This command allows users to simultaneously direct output to a file or a variable while continuing to pass the data through the pipeline. The benefit of this command is that it negates the need for multiple executions of potentially resource-intensive commands just to capture and display data, thereby optimizing both performance and readability of scripts.
Use case 1: Output processes to a file and to the console
Code:
Get-Process | Tee-Object -FilePath path\to\file
Motivation:
This use case is particularly valuable for system administrators or developers who need to periodically monitor and log the running processes on a system. By using Tee-Object
, they can effortlessly generate a snapshot of the current processes, saving them to a file for further analysis while still displaying the data in real-time on the console for immediate inspection.
Explanation:
Get-Process
: This is a commandlet in PowerShell that retrieves information about the processes that are currently running on the system. It provides a detailed overview, including process identifiers, memory usage, and more.|
: This is a pipeline operator in PowerShell, used to pass the output from one command as the input into another. Here, it passes the list of processes to theTee-Object
command.Tee-Object
: The command at the heart of this example, which duplicates the output to a file while continuing to pass it down the pipeline.-FilePath path\to\file
: This argument specifies where the output data should be saved. You need to replacepath\to\file
with the desired file path where you want to store the process list. This file will then contain all the process details captured at the moment the command was executed.
Example Output:
Upon execution, this command will write the output of Get-Process
to both the specified file and the console. For instance, the console might display a list of processes with their names, IDs, and memory usage, while the exact information gets stored in the file outlined.
Use case 2: Output processes to a variable and Select-Object
Code:
Get-Process notepad | Tee-Object -Variable proc | Select-Object processname,handles
Motivation:
This example caters to scenarios where we need a mixture of data processing and data capture for on-demand inspection or debugging purposes. For instance, a developer may want to monitor the notepad
process over time, storing its process-specific data for later analysis and dynamically inspecting particular attributes without storing extraneous data.
Explanation:
Get-Process notepad
: Here, theGet-Process
commandlet is specifically seeking out the ’notepad’ process, meaning it filters to display only information pertinent to the Notepad application if it is currently running.|
: The pipeline operator sends the data about the ’notepad’ process down the pipeline toTee-Object
.Tee-Object
: Again, this command is used for duplicating the output — this time directing it to a variable instead of a file.-Variable proc
: This argument tells PowerShell to store the output into a variable namedproc
. Thus, you can later access the full data on the ’notepad’ process via this variable within your script without needing to rerun theGet-Process
command.| Select-Object processname,handles
: Finally, this part of the pipeline filters and selects specific attributes from the output — in this case,processname
andhandles
. This means only these two attributes will appear as the outward-facing result at the console, providing a focused view while the entire dataset is stored in the variable.
Example Output:
Executing this command will populate the proc
variable with comprehensive details about the ’notepad’ process. At the same time, the console will display only the selected fields, such as:
processname handles
------------ -------
notepad 54
Conclusion:
In conclusion, the Tee-Object
command in PowerShell is instrumental in scenarios where you need to capture command output for later use while continuing to process or inspect the data immediately. It streamlines data handling and ensures that the output is accessible for both immediate viewing and future reference, enhancing the efficiency of scripts and system management tasks.