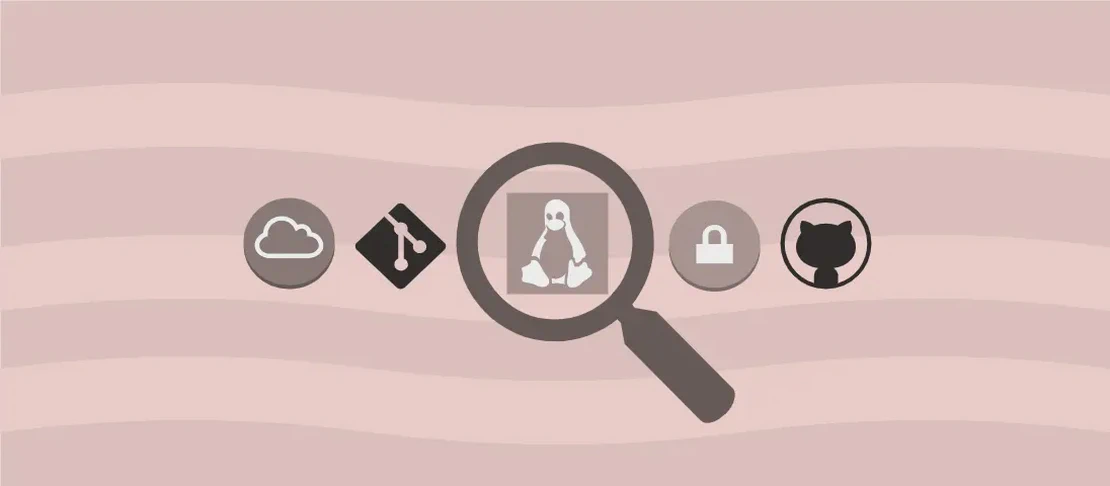
How to use the command 'terraform' (with examples)
Terraform is an open-source tool that allows developers to define and provision infrastructure as code. This powerful utility is widely used in DevOps teams to automate the setup and management of infrastructure across multiple cloud providers, all through concise configuration files. Terraform handles the life cycle of your infrastructure with a clear and consistent workflow, ensuring that your configuration files are syntactically correct and adhere to best practices.
Use case 1: Initialize a new or existing Terraform configuration
Code:
terraform init
Motivation:
Executing terraform init
is the very first step in setting up your Terraform project. It’s a crucial command because it prepares the working directory containing Terraform configuration files and initializes the backends and modules. This ensures that all necessary plugins for interacting with your cloud provider’s APIs are installed and configured correctly. You should run this command whenever you create a new Terraform directory or after pulling an existing project from version control to set up your working environment.
Explanation:
- There are no arguments provided in this basic example of the
terraform init
command. However, this command can take various optional flags to specify backends and more, though they are not needed in most straightforward use cases.
Example output:
Initializing the backend...
Initializing provider plugins...
- Finding latest version of hashicorp/aws...
- Installing hashicorp/aws v3.48.0...
- Installed hashicorp/aws v3.48.0 (signed by HashiCorp)
Terraform has been successfully initialized!
Use case 2: Verify that the configuration files are syntactically valid
Code:
terraform validate
Motivation:
After writing or modifying your Terraform configuration files, it’s important to ensure they are syntactically valid before planning or applying changes. The terraform validate
command checks the configuration files for syntax errors. This ensures that any syntax issues are caught early in the development cycle, thus saving you from potential runtime errors when you actually attempt to create or alter infrastructure.
Explanation:
- Again, this command does not require additional arguments for basic validation. It checks all configuration files in the current directory by default. It can accept directory paths as arguments if needed.
Example output:
Success! The configuration is valid.
Use case 3: Format configuration according to Terraform language style conventions
Code:
terraform fmt
Motivation:
Keeping your code clean and readable is essential for project maintenance and collaboration. terraform fmt
applies standard formatting to your Terraform configurations, ensuring your code adheres to Terraform’s style conventions. This automatic formatting helps prevent syntax issues related to misaligned indentation or missing spacing and makes the configuration files consistent for anyone reviewing the code.
Explanation:
- Without arguments,
terraform fmt
will format all Terraform configuration files in the current directory. You can specify additional options to customize the behavior, though in simple cases, the defaults are sufficient.
Example output:
main.tf
variables.tf
Use case 4: Generate and show an execution plan
Code:
terraform plan
Motivation:
Before applying changes to your infrastructure, you should know what changes Terraform intends to make. The terraform plan
command generates an execution plan, showing what resources will be created, changed, or destroyed. This is an invaluable step for reviewing changes and ensuring they match your expectations before making any real alterations to your infrastructure.
Explanation:
terraform plan
can accept options to provide additional configuration files, variables, or targets, but in its basic form, it simply uses the configuration in the current directory.
Example output:
Refreshing Terraform state in-memory prior to plan...
No changes. Infrastructure is up-to-date.
Use case 5: Build or change infrastructure
Code:
terraform apply
Motivation:
Once you are satisfied with the proposed changes shown by terraform plan
, the next step is to implement these changes by executing terraform apply
. This command applies the changes required to reach the desired state of the configuration, effectively creating, updating, or destroying resources on your cloud provider as needed. It’s a critical command for actualizing infrastructure changes.
Explanation:
- Running
terraform apply
uses your currently defined configuration files to update your infrastructure. You can pass a previously saved plan file if you want to separate the plan and apply stages strictly.
Example output:
Terraform will perform the following actions:
# aws_instance.example will be created
+ resource "aws_instance" "example" {
...
}
Plan: 1 to add, 0 to change, 0 to destroy.
Use case 6: Destroy Terraform-managed infrastructure
Code:
terraform destroy
Motivation:
Sometimes, you need to tear down your infrastructure, either because it’s no longer needed, or you want to start fresh with a new configuration. terraform destroy
is used to destroy all resources managed by Terraform within your configuration. This command is vital for resource cleanup and cost management, as it ensures that you’re not charged for resources you no longer need.
Explanation:
terraform destroy
uses the configuration files to determine which resources to remove. You can supply additional flags to confirm operations or target specific resources to be destroyed.
Example output:
Do you really want to destroy all resources?
... (yes/no)
Destroy complete! Resources: 1 destroyed.
Conclusion:
Terraform simplifies cloud infrastructure management by allowing developers and system administrators to automate the setup, changes, and destruction of infrastructure using declarative configuration files. Through commands like terraform init
, terraform validate
, terraform fmt
, terraform plan
, terraform apply
, and terraform destroy
, users can manage the entire lifecycle of their infrastructure efficiently and effectively. Each command serves a distinct and essential function in the Terraform workflow, ensuring that infrastructure is reliable, consistent, and transparent.