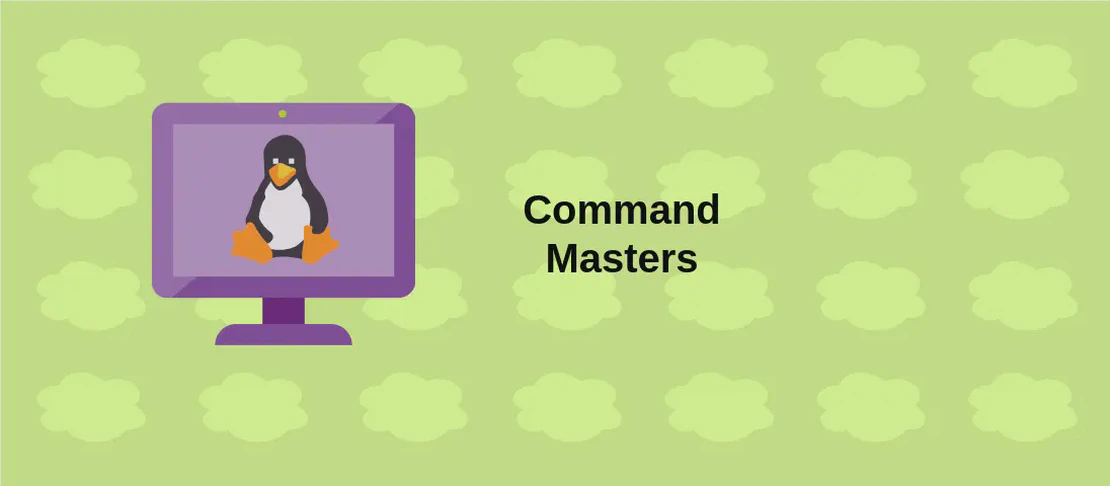
How to Use the Command 'terraform plan' (with Examples)
The terraform plan
command is a critical component of the Terraform infrastructure-as-code workflow, offering users the ability to preview the changes that Terraform will apply when executing an infrastructure update. By simulating the execution of the underlying resource actions, it provides a clear and understandable plan that allows users to confirm configurations and will help prevent unwanted consequences. This step is essential for ensuring smooth and controlled infrastructure deployments and updates.
Use case 1: Generate and Show the Execution Plan in the Current Directory
Code:
terraform plan
Motivation:
This command is often used as the first step in the Terraform workflow, allowing users to review the potential changes that Terraform will apply to their infrastructure. It provides a detailed blueprint of what Terraform intends to change, create, or destroy, aiding in transparency and foresight into infrastructure modifications.
Explanation:
terraform
: The command-line interface tool used for provisioning and managing infrastructure using code.plan
: The sub-command that generates an execution plan, displaying changes Terraform will make to reach the desired state.
Example Output:
Terraform will perform the following actions:
+ create new_instance
ami: "ami-123456"
instance_type: "t2.micro"
Plan: 1 to add, 0 to change, 0 to destroy.
Use case 2: Show a Plan to Destroy All Remote Objects That Currently Exist
Code:
terraform plan -destroy
Motivation:
This command helps in scenarios where a complete teardown of infrastructure is required. It displays a plan that would result in the destruction of all resources managed by the current Terraform configuration, helping administrators anticipate the impact and ensure that no unintended resources are removed.
Explanation:
-destroy
: This argument modifies the usual plan operation to propose destroying all resources. It is crucial for safely evaluating the impact of a full teardown.
Example Output:
Terraform will perform the following actions:
- destroy instance
instance_id: "i-0abcdef1234567890"
Plan: 0 to add, 0 to change, 1 to destroy.
Use case 3: Show a Plan to Update the Terraform State and Output Values
Code:
terraform plan -refresh-only
Motivation:
When the goal is to synchronize the state file with real infrastructure without making changes, this command is invaluable. It focuses on updating resource states and output values to reflect current realities without altering the actual infrastructure.
Explanation:
-refresh-only
: Directs Terraform to update only the state file and outputs, not making any modifications to resources. This is useful for accuracy in reporting and debugging.
Example Output:
Terraform will perform the following actions:
~ refresh instance
state: "running" -> "stopped"
Plan: 0 to add, 0 to change, 0 to destroy.
Use case 4: Specify Values for Input Variables
Code:
terraform plan -var 'name1=value1' -var 'name2=value2'
Motivation:
This use case is essential when you want to override or provide specific values for variables during the planning phase. These values might vary depending on different environments, making it adaptable for various deployment scenarios.
Explanation:
-var 'name=value'
: This flag allows you to specify custom values for input variables defined in the Terraform configuration. Each-var
flag assigns a distinct value to a variable.
Example Output:
Terraform will perform the following actions:
+ update server
name: "value1"
size: "value2"
Plan: 1 to add, 0 to change, 0 to destroy.
Use case 5: Focus Terraform’s Attention on Only a Subset of Resources
Code:
terraform plan -target resource_type.resource_name[instance index]
Motivation:
This command is particularly useful when you want to isolate and focus on specific resources within a larger Terraform configuration. It helps in applying changes incrementally, minimizing the risk of impacting unrelated resources.
Explanation:
-target resource_type.resource_name[instance index]
: Restricts the plan to only include the specified resource or resources. Useful for fine-grained control over resource management.
Example Output:
Terraform will perform the following actions:
~ update instance_type
instance_id: "i-0abcdef1234567890"
old_instance_type: "t2.micro" -> "t2.small"
Plan: 0 to add, 1 to change, 0 to destroy.
Use case 6: Output a Plan as JSON
Code:
terraform plan -json
Motivation:
Exporting the plan output as JSON is vital for automation and integration with other tools and systems. JSON output can be parsed by applications and scripts, enabling automated decision-making and analysis.
Explanation:
-json
: Produces a machine-readable JSON formatted output, facilitating integrations and automation scripts to programmatically consume the execution plan.
Example Output:
{
"resource_changes": [
{
"change": {"actions": ["no-op"]}
}
],
"format_version": "0.1"
}
Use case 7: Write a Plan to a Specific File
Code:
terraform plan -no-color > path/to/file
Motivation:
Directing output to a file is useful for record-keeping, manual review, or sharing with team members who may not have direct access to your Terraform environment. It can also help in auditing and change management processes.
Explanation:
-no-color
: This flag ensures that the output is devoid of any ANSI color codes which can make file contents hard to parse or read in plain text.> path/to/file
: Redirects the command’s output to a specified file, making it persistent and easily retrievable.
Example Output (content of file at path/to/file):
Terraform will perform the following actions:
+ create new_instance
ami: "ami-123456"
instance_type: "t2.micro"
Plan: 1 to add, 0 to change, 0 to destroy.
Conclusion:
The terraform plan
command is an indispensable tool in infrastructure-as-code practices, providing vital foresight into the potential effects of applying infrastructure changes. With its diverse usage scenarios, ranging from simple plan generation to JSON output for automated systems, it empowers users with control and clarity in managing complex cloud environments. Each example above highlights specific scenarios catered to different needs, maintaining reliability and precision across varied Terraform operations.