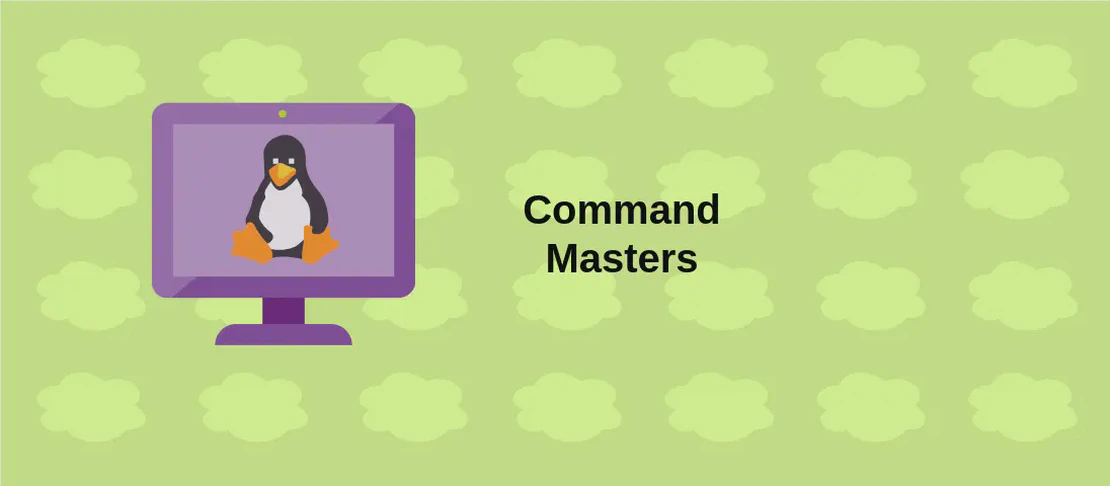
How to Use the Command 'test' (with examples)
The test
command is a fundamental utility commonly used in Unix-like operating systems to perform different types of checks in shell scripts. It evaluates expressions and returns a status code based on the outcome—0 if the condition is true and 1 if false. This command is instrumental for controlling the flow of scripts by checking file types, variable comparisons, and the existence of files or directories.
Test if a Given Variable is Equal to a Given String
Code:
test "$MY_VAR" = "/bin/zsh"
Motivation: Checking whether a variable is equal to a specific string is fundamental in scripting. It is often used to verify the current environment or condition, such as verifying which shell is being used. Such checks can determine the script’s next actions or facilitate compatibility checks.
Explanation: The test
command uses the equality operator =
to assess if the content of the variable MY_VAR
is exactly equal to the string /bin/zsh
. The variable is enclosed with double quotes to ensure the command handles cases where the variable could be empty or contain spaces.
Example Output: If MY_VAR
is set to /bin/zsh
, the command outputs nothing (indicative of a successful test with zero exit status). If MY_VAR
does not equal /bin/zsh
, the command outputs nothing, but it will return a non-zero exit status.
Test if a Given Variable is Empty
Code:
test -z "$GIT_BRANCH"
Motivation: Determining if a variable is empty is a frequent operation in scripts where the presence or absence of a value affects the next steps. Testing for empty variables can be crucial in conditional configurations, such as identifying if a certain feature or environment variable has been set.
Explanation: The -z
flag within the test
command checks whether the length of the variable GIT_BRANCH
is zero. By using this option, you’re essentially asking, “Is this variable empty?” The variable is double-quoted to protect against any special characters or spaces that could disrupt the evaluation.
Example Output: If GIT_BRANCH
is indeed empty, the test
command will return 0. No output is displayed on the console, but you can verify the result by checking the return status. If it contains any value, the exit status will be 1.
Test if a File Exists
Code:
test -f "path/to/file_or_directory"
Motivation: Checking the presence of a file is a common operation whenever scripts need to read from or write to a specific file. This use case enables scripts to prevent errors related to non-existent files and take appropriate actions, such as creating a file if it does not exist or skipping operations on files absent from the filesystem.
Explanation: The -f
option checks for the existence of a file at the given path. If the path leads to a regular file, the test
command returns true. Note that this does not apply to directories or special files. The path should be quoted to handle spaces or unusual characters.
Example Output: If the specified file exists, the command will succeed with a return status of 0. If the file does not exist, no output will be displayed, but the exit status will be 1.
Test if a Directory Does Not Exist
Code:
test ! -d "path/to/directory"
Motivation: Ensuring that a directory does not exist can be as important as ensuring one does. Scripts often need to prevent overwriting or altering data in an existing directory or ensure cleanliness by verifying the absence of a directory before executing certain operations, such as initializing a new version control repository.
Explanation: The -d
flag checks for the presence of a directory. Accompanied by a logical NOT (!
), it inverts the result, succeeding only if the directory does not exist at the specified path. As with file checks, the path should be quoted for safety.
Example Output: If the directory does not exist, the command returns 0. In the case where the directory is present, it returns a non-zero status with no console output.
If A is True, Then Do B, or C in the Case of an Error
Code:
test condition && echo "true" || echo "false"
Motivation: This compound command structure allows for conditional logic in scripts, enabling alternate actions based on the evaluation of a condition. Such logic is essential in scripting for error handling and providing informative outputs when conditions are met or fail.
Explanation: Here, the test condition
evaluates some expression. The logical AND &&
operator ensures that echo "true"
runs only if the test condition is true. If the condition is false, the logical OR ||
will cause the following command, echo "false"
, to execute. This simple structure allows for clear bifurcation in script logic.
Example Output: If test condition
is true, the console will display “true”. If false, it will display “false”. This method provides a straightforward, terminal-friendly way to communicate the result of the test.
Conclusion:
The test
command is an invaluable tool in scripting within Unix-like environments. Its simplicity yet powerful capacity to evaluate expressions offers flexibility and control over scripts’ behaviors. By understanding and leveraging its use cases, such as comparing strings, checking for empty variables, or verifying file and directory existence, you can write robust and efficient scripts that handle various conditions gracefully.