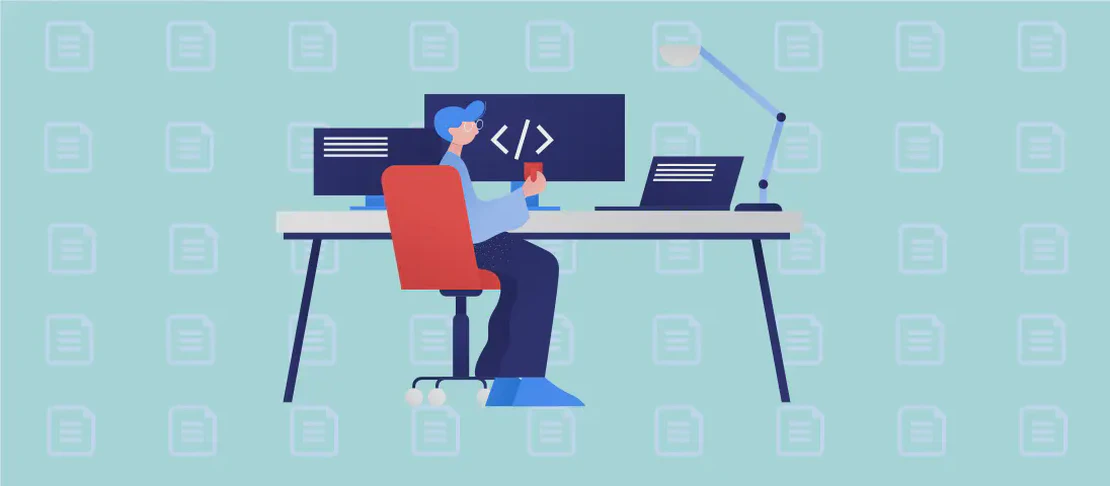
How to Use the Command 'Test-Json' (with examples)
The Test-Json
command is a feature in PowerShell that allows users to validate whether a string is in JSON format. This command is incredibly useful for developers and system administrators who frequently work with APIs, configuration files, or any data interchange formats where JSON is commonly used. By ensuring data is valid JSON, you can prevent potential errors in data processing and enable seamless data exchange between systems.
Use case 1: Testing if a string from stdin is in JSON format
Code:
'{"name":"John", "age":30, "city":"New York"}' | Test-Json
Motivation:
The first use case illustrates a situation where you may have JSON data stored in a variable or received as input during a script execution. You want to verify the data’s integrity and correctness before processing it further. By piping a string into Test-Json
, you can confirm whether the data you’re working with is valid JSON.
Explanation:
'{"name":"John", "age":30, "city":"New York"}'
: This is a simple JSON string representing an object with properties forname
,age
, andcity
.|
: The pipe operator sends the output of the left-hand command to the right-hand command in PowerShell.Test-Json
: The command that tests if the input string is a valid JSON document.
Example Output:
True
Use case 2: Testing if a string is JSON format directly
Code:
Test-Json -Json '{"name":"Doe", "email":"doe@example.com"}'
Motivation:
In this case, you have direct access to the string within your script or terminal, and you want to ensure it is a valid JSON string. By providing the -Json
argument and the string directly, you validate its format without needing to pipeline input, ideal for one-off checks or debugging.
Explanation:
Test-Json
: Initializes the command to validate JSON.-Json
: This parameter specifies that the following string is to be tested for being a valid JSON document.'{"name":"Doe", "email":"doe@example.com"}'
: The string you wish to validate. It represents a JSON object containing properties such asname
andemail
.
Example Output:
True
Use case 3: Testing if a string from stdin matches a specific schema file
Code:
'{"name":"Alice", "age":25}' | Test-Json -SchemaFile path\to\schema_file.json
Motivation:
When working with JSON data, validating not only its format but also its structure against a predefined schema is essential. This ensures that the JSON data conforms to specific rules and constraints, such as data types or required fields. This use case is crucial when enforcing data contracts in APIs or ensuring database inputs meet certain criteria.
Explanation:
'{"name":"Alice", "age":25}'
: A JSON string representing an object you wish to validate.|
: Pipes the JSON string into the next command.Test-Json
: The PowerShell command to test JSON validity.-SchemaFile
: This parameter allows you to specify a JSON Schema file against which the JSON string will be validated.path\to\schema_file.json
: This is the path to the JSON Schema file. This file contains defined constraints and structures that the input data should adhere to.
Example Output:
True
Conclusion
The Test-Json
command offers a set of versatile capabilities for ensuring JSON data’s validity and conformity to expected structures. By checking JSON format and schema compliance, developers can guarantee data integrity before further processing, ultimately leading to fewer runtime errors and consistent data handling. These examples illustrate how this command is used effectively in various scenarios, emphasizing its crucial role in a wide range of data management operations.