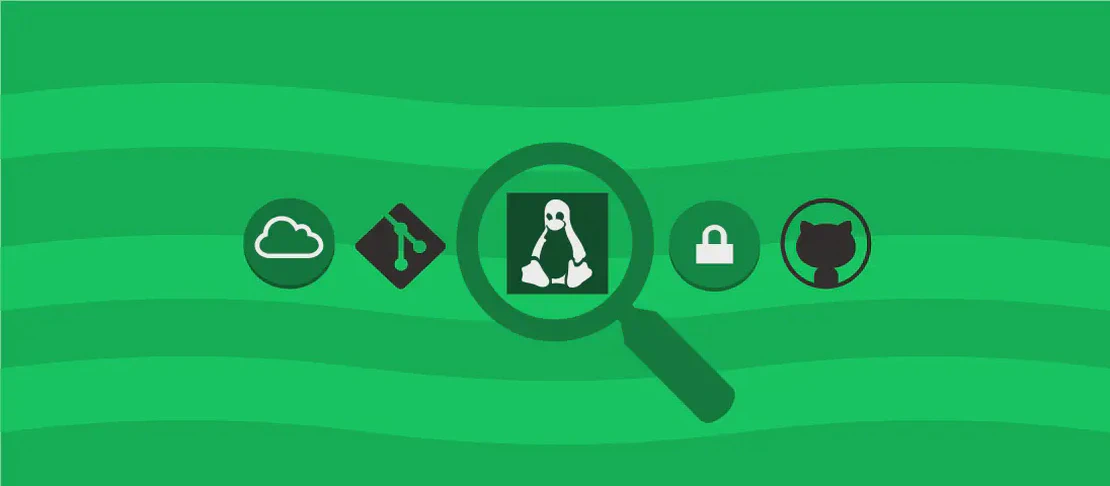
How to use the command 'tr' (with examples)
The tr
command in Unix-based systems is a powerful utility used for translating or modifying characters in text. It stands for “translate” and is part of the GNU Core Utilities. The command processes text streams, converting specified characters into alternative characters, deleting characters, or squeezing multiple occurrences of a character into a single instance. As a versatile tool, it’s frequently used in shell scripting and command-lining for data transformation tasks.
Use case 1: Replace all occurrences of a character in a file, and print the result
Code:
tr 'a' 'e' < path/to/file
Motivation:
Imagine you have a text file containing a large number of documents where every occurrence of the letter ‘a’ needs to be transformed into the letter ’e’ for language processing or data transformation purposes. This task can be mundane and error-prone if done manually. Automating the process using tr
helps ensure accuracy and saves time.
Explanation:
tr
: Invokes the translate command.'a'
: Specifies the character to find, which is ‘a’ in this case.'e'
: Specifies the character to replace ‘a’ with, which is ’e’.< path/to/file
: Directs the content of a file to be processed by thetr
command.
Example output:
Before: “A cat is at the table.” After: “E cet is et the teble.”
Use case 2: Replace all occurrences of a character from another command’s output
Code:
echo 'banana' | tr 'a' 'o'
Motivation:
You might be working with outputs from other scripts or commands, such as database queries or web scraping results, and need to replace specific characters within them. By piping the output to tr
, you can immediately transform the output without storing it in a separate file.
Explanation:
echo 'banana'
: Generates a string ‘banana’ as standard output.|
: Pipes the output of the previous command as input totr
.tr 'a' 'o'
: Replaces every occurrence of ‘a’ with ‘o’ in the piped output.
Example output:
Original: banana Transformed: bonono
Use case 3: Map each character of the first set to the corresponding character of the second set
Code:
tr 'abcd' 'jkmn' < path/to/file
Motivation:
Suppose you have a file containing a string of text, and you want to apply consistent letter substitution, such as ciphers or encoding text, where each original character is replaced with its corresponding new character. This method ensures an accurate mapping from one character set to another.
Explanation:
tr 'abcd' 'jkmn'
: Translates characters ‘a’, ‘b’, ‘c’, ’d’ to ‘j’, ‘k’, ’m’, ’n’, respectively.< path/to/file
: Indicates that the content of a file will be read and transformed.
Example output:
Input: “a bad cad” Output: “j kjk mjm”
Use case 4: Delete all occurrences of the specified set of characters from the input
Code:
tr -d 'aeiou' < path/to/file
Motivation:
In some cases, you might need to remove certain vowels or specific characters from text data for analysis that excludes these characters. This functionality simplifies the process by eliminating the unnecessary characters in one go.
Explanation:
tr -d 'aeiou'
: Deletes all vowels ‘a’, ’e’, ‘i’, ‘o’, ‘u’ from the input stream.< path/to/file
: Specifies the file whose content is processed.
Example output:
Before: “This is an example.” After: “Ths s n xmpl.”
Use case 5: Compress a series of identical characters to a single character
Code:
tr -s ' ' < path/to/file
Motivation:
Documents or data received from different sources might contain inconsistent spacing, such as multiple spaces between words. To clean up this data, compressing multiple spaces into a single space ensures consistent formatting and improves readability.
Explanation:
tr -s ' '
: The-s
option compresses sequences of consecutive spaces down to a single space.< path/to/file
: Designates the file whose spaces need to be squeezed.
Example output:
Before: “This is a test.” After: “This is a test.”
Use case 6: Translate the contents of a file to upper-case
Code:
tr "[:lower:]" "[:upper:]" < path/to/file
Motivation:
Upper-casing all text is a frequent requirement when preparing data for case-insensitive comparisons, formatting, or shouting (in the internet’s parlance). By translating all lowercase letters to uppercase, you ensure consistency in data representation.
Explanation:
tr "[:lower:]" "[:upper:]"
: Translates all lowercase alphabetic characters to their uppercase equivalents.< path/to/file
: File input to be processed for upper-casing.
Example output:
Before: “good morning!” After: “GOOD MORNING!”
Use case 7: Strip out non-printable characters from a file
Code:
tr -cd "[:print:]" < path/to/file
Motivation:
Data files sometimes include non-printable characters, such as control characters, which can cause problems during data processing. Stripping these characters ensures cleaner data and can prevent errors in data parsing or display.
Explanation:
tr -cd "[:print:]"
: The-c
option complements the set, and-d
deletes the complemented set, therefore removing non-printable characters.< path/to/file
: Specifies that the file content should be processed to remove unwanted characters.
Example output:
Before: “Hello, \x02world!\x03” After: “Hello, world!”
Conclusion:
The tr
command is a versatile tool for text transformation and manipulation, capable of substituting, deleting, and translating character strings within text files or streams. By understanding and applying the above use cases, you can leverage tr
to handle a wide array of text processing tasks efficiently. Whether updating file contents, cleaning data, or modifying command outputs, tr
remains an essential utility in any command-line toolkit.