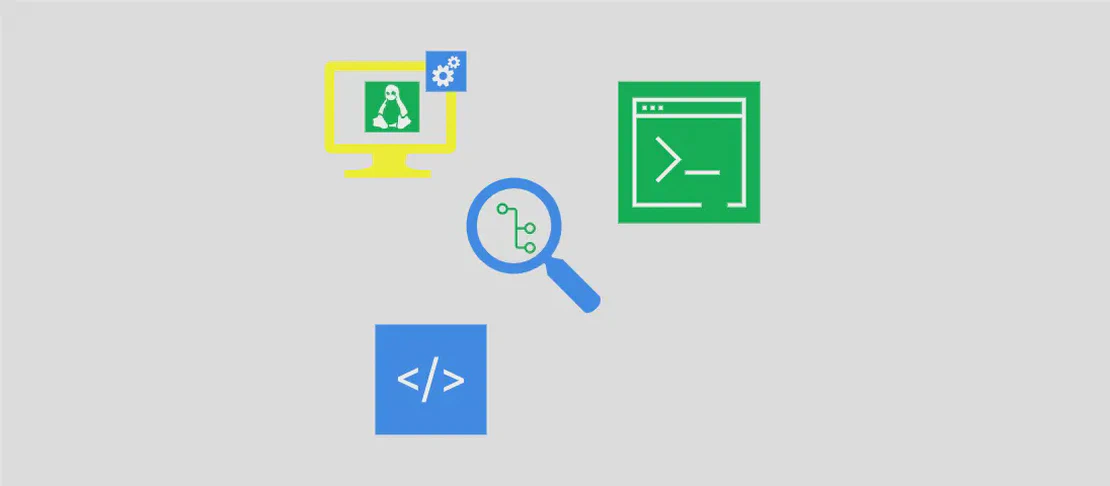
Understanding the 'trap' Command in Bash (with examples)
- Linux
- December 17, 2024
The ’trap’ command in Bash scripting is a powerful tool allowing scripts to handle signals and execute specified commands when particular events occur. By strategically using the ’trap’ command, scripts can become more robust, gracefully manage interruptions, and execute clean-up procedures at the right moments. This capability is vital for anyone looking to harness advanced Bash scripting practices for managing their processes effectively.
Use case 1: Listing Available Event Names
Code:
trap -l
Motivation:
Understanding the range of signals that the ’trap’ command can handle is crucial for a script developer who wants to make scripts fault-tolerant and responsive to various system events. By listing all available event names, you gain a comprehensive view of all signals that can be caught and handled in a script.
Explanation:
trap
: This is the command used to set up a trap to catch signals and execute commands.-l
: This option lists all the signal names that the script may trap. Knowing these signals helps you understand what events and conditions you can manage using the ’trap’ command.
Example Output:
1) SIGHUP 2) SIGINT 3) SIGQUIT 4) SIGILL 5) SIGTRAP 6) SIGABRT ...
Use case 2: Listing Commands and Expected Events
Code:
trap -p
Motivation:
Once traps have been set in a script, it can be difficult to remember which signals are being caught and what actions will occur as a result. Listing all current traps ensures you have visibility into the signal handling logic, promoting better transparency in your scripts. This is particularly beneficial during debugging or when multiple developers collaborate on a project.
Explanation:
trap
: As before, this sets up signal catching.-p
: This option stands for “print” and outputs the current trap commands and the signals they respond to. It provides a snapshot of the event-command mappings currently active in the script or environment.
Example Output:
trap -- 'echo "Caught signal SIGHUP"' SIGHUP
trap -- 'echo "Caught signal SIGINT"' SIGINT
Use case 3: Executing a Command When a Signal is Received
Code:
trap 'echo "Caught signal SIGHUP"' SIGHUP
Motivation:
In some scenarios, it is imperative to execute specific commands when certain signals are received. For instance, a script may need to perform a cleanup operation or log activity for audit purposes when a terminal disconnects unexpectedly (SIGHUP), ensuring no data is lost and that the system remains in a consistent state.
Explanation:
trap
: This command sets up signal handling.'echo "Caught signal SIGHUP"'
: This is the command that will be executed when the specified signal is received. It serves as an alert, indicating that the SIGHUP signal has been caught.SIGHUP
: This is the specific signal being trapped. It typically indicates that a terminal connection has been lost.
Example Output:
Caught signal SIGHUP
Use case 4: Removing Commands
Code:
trap - SIGHUP SIGINT
Motivation:
Overriding or removing traps that have been previously set provides flexibility and control over how signals are treated at different stages of a script’s execution. This capability is useful when you want to temporarily ignore certain signals or reset the default behaviors, enhancing the script’s adaptability to changing needs or environments.
Explanation:
trap
: Initiates the setting or removal of signal handling.-
: This signals the removal of any commands associated with the specified signals, effectively resetting their handling to default behavior.SIGHUP SIGINT
: These are the signals for which the commands are being removed. SIGHUP typically signals a terminal disconnect, while SIGINT often corresponds to an interrupt signal like a Ctrl+C from the user.
Example Output:
There is no direct output from this command, as it alters the signal behavior internally.
Conclusion:
The ’trap’ command in Bash is indispensable for writing resilient and feature-rich scripts that behave predictably under various conditions. Each use case demonstrated here—from listing available signals to setting and removing event-command pairs—illustrates an essential aspect of signal handling. Mastery of these elements is crucial for anyone aiming to enhance their Bash scripting proficiency and develop scripts robust enough to handle real-world operating conditions and user interactions gracefully.