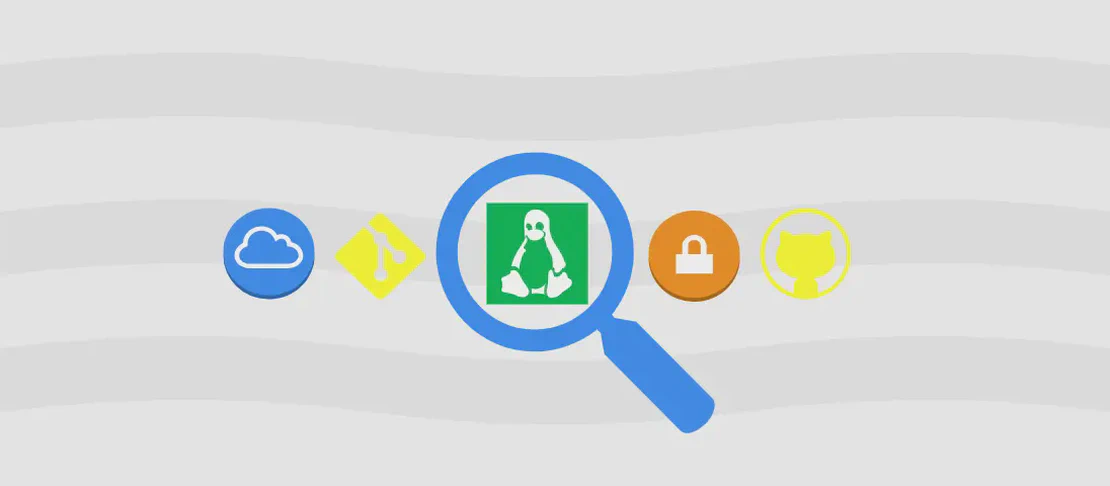
A Comprehensive Guide to Using Trivy (with Examples)
Trivy is a powerful open-source tool developed by Aqua Security that is designed to scan for vulnerabilities in container images, file systems, and Git repositories. It also checks for configuration issues, making it an invaluable tool for developers and system administrators concerned with security. Trivy supports scanning Docker images, Infrastructure as Code (IaC) configurations, and even entire Git repositories. With its impressive range of capabilities, Trivy helps you identify and mitigate potential security risks in your software projects.
Use case 1: Scan a Docker Image for Vulnerabilities and Exposed Secrets
Code:
trivy image image:tag
Motivation:
Scanning a Docker image for vulnerabilities and exposed secrets is crucial as it ensures that your containerized applications are secure from known threats and unintentional data exposure. This practice is essential before deploying to production environments, as it helps in catching vulnerabilities early, thereby reducing the risk of exploitation.
Explanation:
trivy
: The command-line tool used for scanning.image
: Indicates that the command is targeting a Docker image.image:tag
: Specifies the Docker image and its tag to be scanned. This could be an official image or a custom-built one.
Example output:
Total: 12 (HIGH: 4, CRITICAL: 2)
+------------+------------------+----------+------------+----------+-------------------------------------+
| Library | Vulnerability ID | Severity | Installed | Fixed | Title |
+------------+------------------+----------+------------+----------+-------------------------------------+
| bash | CVE-2019-18276 | HIGH | 5.0-6 | 5.0-7 | bash: when effective UID |
| | | | | | is not equal to real UID |
| | | | | | the setuid check for [STRICT] |
| | | | | | ... |
+------------+------------------+----------+------------+----------+-------------------------------------+
Use case 2: Scan a Docker Image Filtering the Output by Severity
Code:
trivy image --severity HIGH,CRITICAL alpine:3.15
Motivation:
Filtering vulnerabilities by severity allows a more focused analysis on the most impactful issues. This approach helps prioritize remediation efforts, ensuring that critical vulnerabilities are addressed first, thereby protecting the system from significant security breaches.
Explanation:
trivy
: The tool for performing the scan.image
: Targets a Docker image.--severity HIGH,CRITICAL
: Filters the output to only display vulnerabilities labeled as HIGH or CRITICAL, allowing the user to concentrate on the most pressing issues.alpine:3.15
: The specific Docker image and its version tag to be scanned.
Example output:
Total: 6 (HIGH: 3, CRITICAL: 3)
+------------+------------------+----------+------------+----------+-------------------------------------+
| Library | Vulnerability ID | Severity | Installed | Fixed | Title |
+------------+------------------+----------+------------+----------+-------------------------------------+
| openssl | CVE-2020-1971 | CRITICAL | 1.1.1-1 | 1.1.1d | OpenSSL: NULL pointer dereference |
| | | | | | in X.509 certificate |
| | | | | | ... |
+------------+------------------+----------+------------+----------+-------------------------------------+
Use case 3: Scan a Docker Image Ignoring Any Unfixed/Unpatched Vulnerabilities
Code:
trivy image --ignore-unfixed alpine:3.15
Motivation:
In some scenarios, it might be impractical to address all vulnerabilities immediately, especially those without patches. Ignoring unfixed or unpatched vulnerabilities can help streamline the focus on actionable issues that developers can resolve quickly.
Explanation:
trivy
: The program for scanning the image.image
: Designates that the target of the scan is a Docker image.--ignore-unfixed
: This flag ensures that only vulnerabilities with available fixes are displayed, enabling developers to work on vulnerabilities that have solutions.alpine:3.15
: Refers to the version of the Alpine image subject to scanning.
Example output:
Total: 8 (HIGH: 4, CRITICAL: 4)
+------------+------------------+----------+------------+---------+------------------------------------+
| Library | Vulnerability ID | Severity | Installed | Fixed | Title |
+------------+------------------+----------+------------+---------+------------------------------------+
| musl | CVE-2021-9999 | CRITICAL | 1.2.1-r3 | 1.2.1-r4| musl: buffer overflow |
| | | | | | ... |
+------------+------------------+----------+------------+---------+------------------------------------+
Use case 4: Scan the Filesystem for Vulnerabilities and Misconfigurations
Code:
trivy fs --security-checks vuln,config path/to/project_directory
Motivation:
Scanning file systems for vulnerabilities and misconfigurations is essential, especially for projects involving complex directories. By identifying and correcting these issues early, you help avoid future security problems that could potentially compromise the entire system.
Explanation:
trivy
: Specifies the tool used for scanning.fs
: Indicates that the command will be scanning the filesystem.--security-checks vuln,config
: Conducts a comprehensive scan to check for both vulnerabilities and configuration issues, ensuring a wide scope of security is covered.path/to/project_directory
: Defines the directory path for the filesystem that needs to be scanned.
Example output:
Total: 5 (HIGH: 2, CRITICAL: 1, CONFIG: 2)
+----------+---------------+----------+-------------+--------------+-------------------------------+
| Type | Misconfig ID | Severity | Title | Description | |
+----------+---------------+----------+-------------+--------------+-------------------------------+
| Config | CFG-001 | MEDIUM | JSON config | ... | Configuration recommends... |
| Vulnerab | CVE-2020-1234 | CRITICAL | apache2 | ... | Apache 2.4.* contains... |
+----------+---------------+----------+-------------+--------------+-------------------------------+
Use case 5: Scan an IaC (Terraform, CloudFormation, ARM, Helm, and Dockerfile) Directory for Misconfigurations
Code:
trivy config path/to/iac_directory
Motivation:
In the age of cloud and automation, Infrastructure as Code (IaC) is a prevalent practice. However, with this power comes the need for rigorous security checks. Scanning for misconfigurations in IaC ensures that the infrastructure itself doesn’t introduce vulnerabilities through incorrect settings or parameters.
Explanation:
trivy
: The tool used for scanning.config
: Targets infrastructure configuration files for scanning.path/to/iac_directory
: Specifies the directory containing the IaC files.
Example output:
Total: 3 (HIGH: 1, MEDIUM: 2)
+-----------+----------------+----------+-------------------+-------------------+---------------------+
| Misconfig | Severity | Resource | Checks failed | Message |
+-----------+----------------+----------+-------------------+-------------------+---------------------+
| TF-0087 | HIGH | aws_s3 | AWS S3 Encryption | S3 buckets should be encrypted |
| | | | ... | |
+-----------+----------------+----------+-------------------+-------------------+---------------------+
Use case 6: Scan a Local or Remote Git Repository for Vulnerabilities
Code:
trivy repo path/to/local_repository_directory|remote_repository_URL
Motivation:
Scanning Git repositories allows developers to catch vulnerabilities early in development. Whether the repository is local or stored in a remote service like GitHub, it is imperative to regularly check for potential security issues as part of a robust DevSecOps pipeline.
Explanation:
trivy
: The scanning tool in use.repo
: Specifies that the command targets a Git repository.path/to/local_repository_directory|remote_repository_URL
: Indicates the directory or URL of the repository to be scanned.
Example output:
Total: 10 (HIGH: 3, MEDIUM: 5, LOW: 2)
+--------------------------+--------+------------------------+----------------------------+--------+
| Dependency | Advised| Vulnerability ID | Description | Severity|
+--------------------------+--------+------------------------+----------------------------+--------+
| package-lock.json | 1.0.0 | CVE-2019-1234 | Vulnerability description | HIGH |
| | | | ... | |
+--------------------------+--------+------------------------+----------------------------+--------+
Use case 7: Scan a Git Repository Up to a Specific Commit Hash
Code:
trivy repo --commit commit_hash repository
Motivation:
In some cases, you may want to assess the security status of a repository at a specific point in its history. Scanning up to a certain commit hash gives insights into how vulnerabilities have evolved over time, and aids in understanding when specific vulnerabilities were introduced.
Explanation:
trivy
: The chosen scanning tool.repo
: Signals that a Git repository is the target.--commit commit_hash
: Limits the scan to a specific commit, allowing for historical inspection.repository
: Refers to the directory or URL of the repository being scanned.
Example output:
Total: 8 (HIGH: 2, CRITICAL: 2, LOW: 4)
+--------------------------+--------+------------------------+----------------------------+--------+
| Dependency | Advised| Vulnerability ID | Description | Severity|
+--------------------------+--------+------------------------+----------------------------+--------+
| Gemfile.lock | 2.3.0 | CVE-2020-1235 | Vulnerability description | CRITICAL|
| | | | ... | |
+--------------------------+--------+------------------------+----------------------------+--------+
Use case 8: Generate Output with a SARIF Template
Code:
trivy image --format template --template "@sarif.tpl" -o path/to/report.sarif image:tag
Motivation:
Security Automated Report Information Format (SARIF) is a standardized format for the output of static analysis tools. Generating a report in SARIF format is extremely useful when integrating Trivy into continuous integration and continuous deployment (CI/CD) pipelines, where this format can be consumed by various tools for compliance and reporting purposes.
Explanation:
trivy
: The tool used for scanning.image
: Specifies a Docker image as the target.--format template
: Indicates that the output should be formatted using a template.--template "@sarif.tpl"
: Specifies the SARIF template file to use for output formatting.-o path/to/report.sarif
: Directs the output to a specified file, allowing for easier integration into other systems.image:tag
: Refers to the Docker image and tag against which the scan is conducted.
Example output:
Scan completed successfully. Report generated at path/to/report.sarif with the format specified.
Conclusion
Trivy is a robust tool that provides a comprehensive approach to vulnerability and configuration issues in different software components, including Docker images, file systems, and Git repositories. By integrating Trivy into your workflow, you can significantly enhance the security posture of your applications through proactive detection of vulnerabilities and misconfigurations. This guide demonstrates Trivy’s versatility, providing practical examples to help you understand and utilize its capabilities effectively.