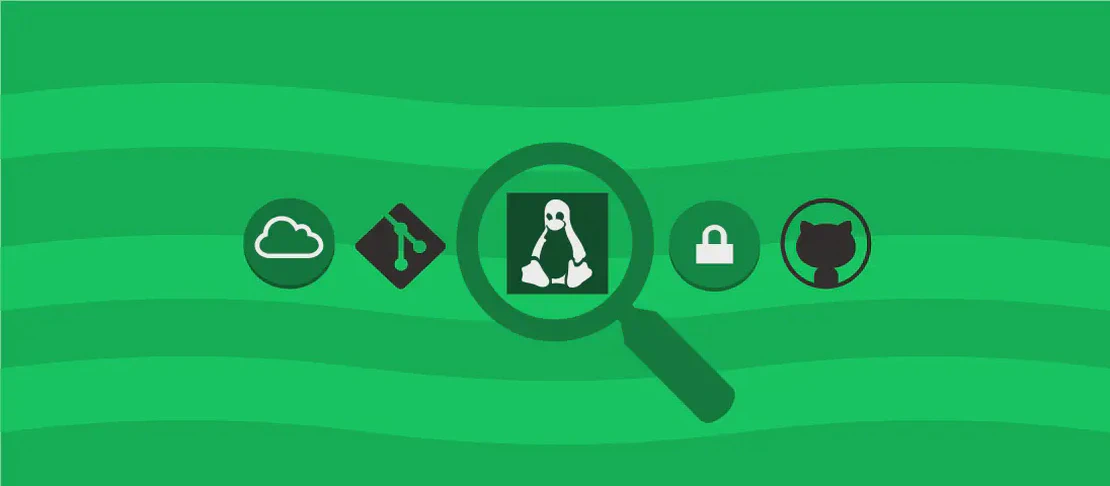
Understanding the 'true' Command in Linux (with examples)
The ’true’ command in Linux is a simple utility that does one thing and does it well: it returns a successful exit status code of 0. This command is particularly useful in shell scripting and conditional execution, especially when you want to ensure that a command always exits successfully regardless of its actual outcome. The success status of ’true’ can be leveraged to control the flow of scripts or command sequences effectively. It’s part of the GNU Core Utilities and can be exceptionally handy in various scenarios where you need a guaranteed successful execution.
Use case 1: Return a Successful Exit Code
Code:
true
Motivation:
In the realm of shell scripting, ensuring that commands execute successfully is a fundamental requirement. The ’true’ command is used when you need a fail-safe mechanism to confirm that a script’s execution reaches a particular point without errors. This is especially relevant when a script’s continuation depends on the success of a preceding operation.
Explanation:
The command ’true’ has no arguments and is as straightforward as it seems. Its primary function is to return an exit status of 0. The absence of any options or flags emphasizes its simplicity—the command’s task is solely to denote ‘success’ unequivocally.
Example Output:
There would be no visible output from this command since ’true’ only returns an exit status. To verify its success, you would typically check the exit status using the $?
variable in a shell script:
echo $?
# Output: 0
Use case 2: Using ’true’ with the ‘||’ Operator to Ensure a Command Always Exits with Success
Code:
false || true
Motivation:
In scripting, sometimes you encounter situations where the outcome of a command isn’t crucial to the successive steps. Perhaps you’re running a command that may fail without impacting the script’s overall mission. By using ’true’ in combination with the ‘||’ operator, you can guarantee that even if the preceding command fails, it won’t influence the exit status of the entire construct. This method is invaluable in testing scripts or log maintenance where certain errors are benign or expected.
Explanation:
In this scenario, the ‘false’ command is expected to return a failure status by default. The ‘||’ operator functions as a logical OR in this expression. Hence, if ‘false’ returns a non-zero status, the shell proceeds to execute ’true’, ensuring that the overall command sequence exits with a status of 0. Utilizing ’true’ ensures that the flow overcomes the failure without any detrimental interruptions to the script or sequence.
Example Output:
No direct output will be visible, similar to the first case. However, examining the exit status will affirm its success:
echo $?
# Output: 0
Conclusion:
The ’true’ command is a quintessential component of shell scripting for situations where guarantees of successful command execution are necessary. It provides an uncomplicated method to steer scripts towards success, particularly when combined with constructs like the ‘||’ operator. Employing ’true’ effectively in scripts can enhance robustness, ensuring smoother execution flows irrespective of intermediate command outcomes. Through these basic yet powerful use cases, ’true’ serves as a versatile instrument in a shell user’s toolkit.