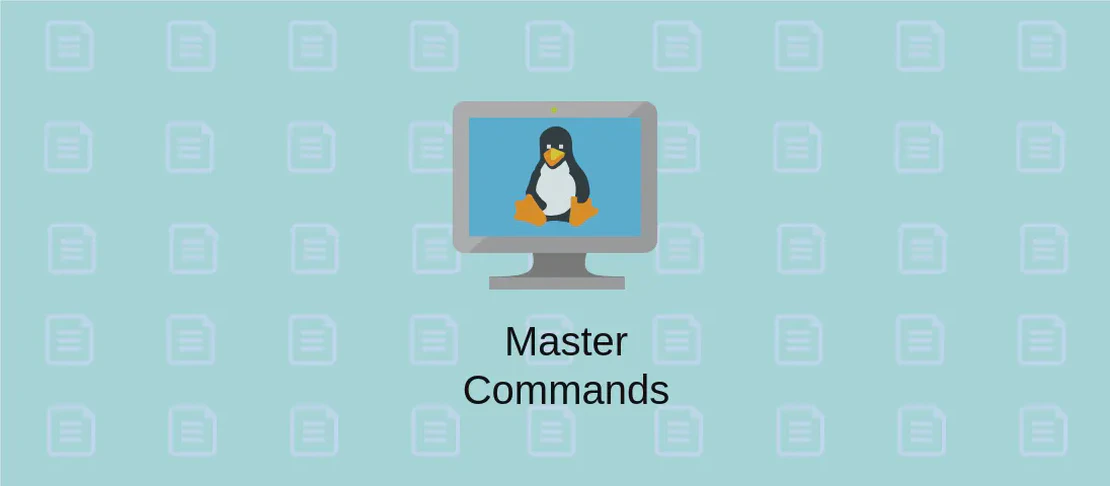
How to Use the Command 'truffle' (with examples)
Truffle is a popular development framework for Ethereum that simplifies the process of writing, testing, and deploying smart contracts. It’s a comprehensive suite of tools that assists developers in managing the entire smart contract development lifecycle, from compiling Solidity code to deploying it on the blockchain, making it easier to build robust and reliable decentralized applications (dApps) on Ethereum. More information on Truffle’s capabilities and detailed documentation can be found at its official site: https://www.trufflesuite.com/docs/truffle/reference/truffle-commands .
Use Case 1: Download a Pre-Built Truffle Project (Truffle Box)
Code:
truffle unbox box_name
Motivation:
Utilizing a Truffle Box is an efficient way to kick-start a blockchain project. These boxes come with pre-configured templates that provide a variety of examples and boilerplate code to help developers focus more on dApp logic rather than setup configuration. Whether you are starting a new project or exploring various features, Truffle Boxes are extremely beneficial.
Explanation:
truffle
: This is the command line interface for the Truffle suite.unbox
: This specifies the command to download and set up a Truffle Box, which is a packaged project template.box_name
: This is a placeholder for the name of the Truffle Box you wish to download. Boxes can range from basic starter templates to more complex setups with React or other technologies integrated.
Example Output:
Following the command, you might see an output indicating the progress of downloading and setting up the box. It will include messages like “Unpacking…” and “Setting up…” and concludes with a success message such as “Unbox successful!”
Use Case 2: Compile Contract Source Files in the Current Directory
Code:
truffle compile
Motivation:
Compiling smart contracts is a crucial step as it converts the Solidity code into bytecode, which can be executed on the Ethereum Virtual Machine (EVM). The compilation process ensures that the contracts are syntactically and semantically valid before deployment.
Explanation:
truffle
: This invokes the Truffle framework.compile
: This command is used to compile all the Solidity files located in the contracts directory of your current Truffle project. It processes the files and generates the necessary JSON artifacts.
Example Output:
You will see a series of messages indicating the compilation progress. For example, “Compiling ./contracts/MyContract.sol…” followed by “Writing artifacts to ./build/contracts…” once the process is complete.
Use Case 3: Run JavaScript and Solidity Tests
Code:
truffle test
Motivation:
Testing smart contracts is essential to detect bugs and ensure functionality before deploying a contract to the blockchain. Truffle provides a robust testing framework that supports both JavaScript and Solidity tests, enabling developers to create comprehensive test suites.
Explanation:
truffle
: This is the tool being utilized to manage the testing process.test
: This command executes all the test files located in the ’test’ directory of the Truffle project. Truffle automatically recognizes files with.js
and.test.js
extensions for JavaScript tests, and.sol
for Solidity tests.
Example Output:
As the tests run, the console will display test results, showing passed/failed tests, any errors, and assertions. It typically includes a summary that shows how many tests were executed and how many succeeded or failed.
Use Case 4: Run Migrations to Deploy Contracts
Code:
truffle migrate
Motivation:
Deploying smart contracts onto a blockchain is a critical aspect of dApp development, allowing the contract to interact with other contracts and users on the network. The migrate
command in Truffle automates this deployment process, recording deployment status so that developers can manage and track changes efficiently.
Explanation:
truffle
: This represents the command line functionality for Truffle.migrate
: This command executes migration scripts found in the ‘migrations’ directory, deploying the smart contracts to the specified Ethereum network. It creates a structured way to deploy updates and ensure contracts are up-to-date.
Example Output:
The output includes messages about each migration script being run, the deployment status, transaction hashes, and network interaction results. You’ll see confirmations like “Deploying Migrations…” and “Migrations dry-run successful”, highlighting each step of the deployment.
Use Case 5: Display Help for a Subcommand
Code:
truffle help subcommand
Motivation:
Understanding the full range of options and features available for each Truffle command is vital for developers who wish to maximize their productivity and precision in managing projects. Using the help function provides detailed information about subcommands, aiding both new and experienced users.
Explanation:
truffle
: Calls the Truffle framework’s command line interface.help
: Requests guidance or more details.subcommand
: Replace this with the specific Truffle command or subcommand for which you need assistance. This reveals a more extensive list of options and arguments available for that particular operation.
Example Output:
Running this command will generate detailed usage information, explanations, and examples for the specified subcommand. It will look like a typical help output seen in command line interfaces, detailing available flags, options, and usage patterns.
Conclusion
The Truffle suite of commands provides Ethereum developers with a powerful set of tools to develop, test, and deploy smart contracts efficiently. Whether you are setting up a new project, compiling code, or deploying dApps, Truffle reliably covers the entire process, empowering developers to focus more on innovation and less on monotonous setup tasks.