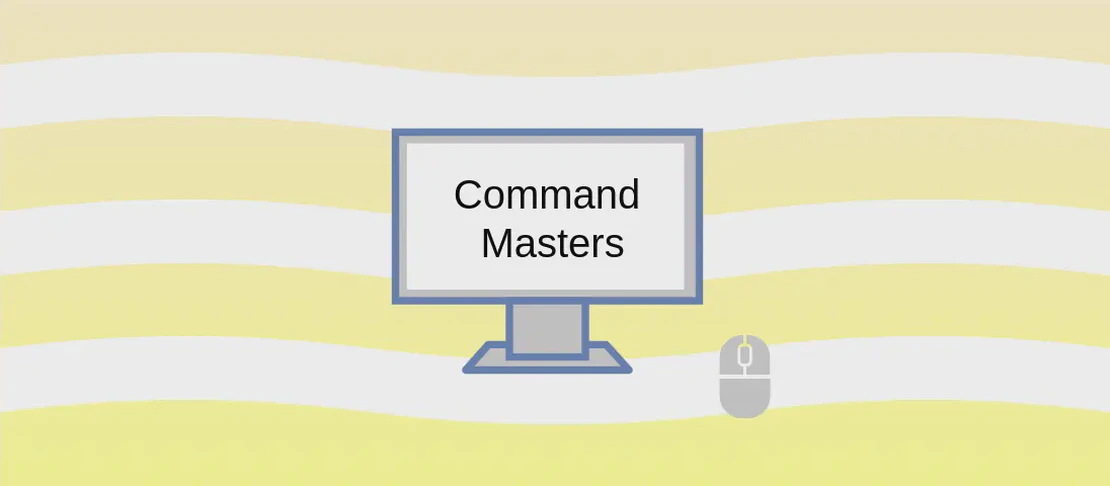
How to use the command ts (with examples)
The ts
command is a useful utility that allows users to add timestamps to the lines of text coming from the standard input. It adds an extra layer of information to the text, making it easier to track and analyze data over time.
Use case 1: Add a timestamp to the beginning of each line
Code:
command | ts
Motivation:
Let’s say you have a streaming log file, and you want to keep track of when each log entry was created. By using the ts
command, you can easily add a timestamp to the beginning of each line. This will help you analyze the log data and identify potential patterns or issues.
Explanation:
The command command | ts
takes the output from the command
and pipes it to the ts
command. This will add a timestamp to each line of output.
Example output:
2022-10-25T15:30:00 This is line 1
2022-10-25T15:30:01 This is line 2
2022-10-25T15:30:02 This is line 3
Use case 2: Add timestamps with microsecond precision
Code:
command | ts "%b %d %H:%M:%.S"
Motivation:
If you need more precise timestamps in your log entries, you can use the ts
command with a custom format. By specifying %b %d %H:%M:%.S
, you will get timestamps with microsecond precision.
Explanation:
The %b %d %H:%M:%.S
format specifies the desired timestamp format. Here, %b
represents the abbreviated month name, %d
represents the day of the month, %H
represents the hour in 24-hour format, %M
represents the minute, and %.S
represents the second with microsecond precision.
Example output:
Oct 25 15:30:00.123456 This is line 1
Oct 25 15:30:01.234567 This is line 2
Oct 25 15:30:02.345678 This is line 3
Use case 3: Add incremental timestamps with microsecond precision, starting from zero
Code:
command | ts -i "%H:%M:%.S"
Motivation:
In some cases, you might need to add incremental timestamps to your data. This can be useful when you want to measure the time elapsed between different events or track the progress of a process. The -i
option in combination with a custom format allows you to achieve this.
Explanation:
The -i
option tells the ts
command to add incremental timestamps. The specified format %H:%M:%.S
represents the hour in 24-hour format, the minute, and the second with microsecond precision.
Example output:
00:00:00.000000 This is line 1
00:00:00.000001 This is line 2
00:00:00.000002 This is line 3
Use case 4: Convert existing timestamps in a text file into relative format
Code:
cat path/to/file | ts -r
Motivation:
When analyzing logs or any other text files with timestamps, it can be beneficial to convert absolute timestamps into relative ones. This allows you to focus on the time intervals between events instead of specific dates and times. The -r
option in the ts
command helps you achieve this.
Explanation:
The -r
option tells the ts
command to convert existing timestamps into relative format. By providing the input file using cat path/to/file
, you can convert the timestamps in that file into relative format.
Example output:
00:00:01.000000 This is line 1
00:01:00.000000 This is line 2
00:02:30.000000 This is line 3
Conclusion:
The ts
command is a versatile tool for adding timestamps to text data. Whether you need absolute or relative timestamps with various levels of precision, ts
provides a straightforward way to enhance your data analysis and monitoring tasks. By applying different use cases of the command, you can gain valuable insights and make more informed decisions based on the timing information provided.