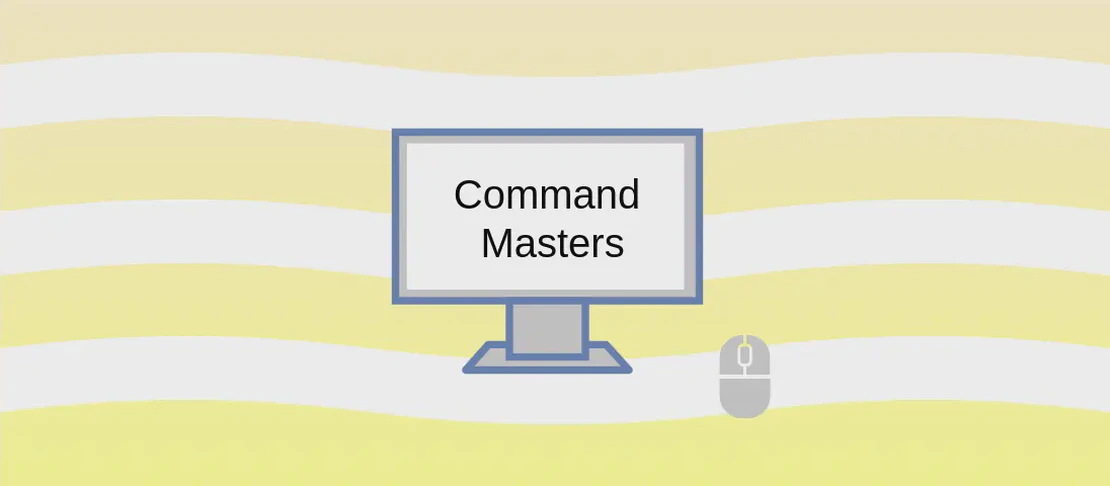
Running TypeScript with 'ts-node' (with examples)
The ts-node
command is a powerful tool for developers working with TypeScript. It allows you to execute TypeScript code directly without the need for separate compilation to JavaScript. This can significantly streamline the development process by allowing developers to write and test code more efficiently. By offering a range of options, ts-node
can adapt to different development needs, whether you want to bypass configuration files or simply evaluate a few lines of code.
Execute a TypeScript file without compiling (node
+ tsc
):
Code:
ts-node path/to/file.ts
Motivation:
When developing TypeScript applications, running TypeScript files usually requires two steps: first compiling the TypeScript files into JavaScript using the tsc
command, and then executing the JavaScript files with node
. With ts-node
, this process is streamlined, allowing developers to execute TypeScript files directly without the manual compilation step. This can be particularly useful during development and testing when changes are frequent.
Explanation:
ts-node
: Invokes thets-node
command to execute TypeScript files without separate compilation.path/to/file.ts
: Specifies the TypeScript file to be executed. The tool will handle compiling it on-the-fly as part of the execution process.
Example Output:
Hello, TypeScript world!
Execute a TypeScript file without loading tsconfig.json
:
Code:
ts-node --skip-project path/to/file.ts
Motivation:
The tsconfig.json
file is often used to specify configuration options for TypeScript projects, like compiler options and which files to include. However, there may be instances where you want to quickly test a file without these configurations, perhaps to verify a quick change or test a snippet independently of project settings. --skip-project
helps achieve that by bypassing any tsconfig.json
.
Explanation:
--skip-project
: This flag tellsts-node
to ignore any existingtsconfig.json
configurations during execution.path/to/file.ts
: Indicates the TypeScript file to run, unaffected by project-level TypeScript configurations.
Example Output:
Running standalone TypeScript file without project settings.
Evaluate TypeScript code passed as a literal:
Code:
ts-node --eval 'console.log("Hello World")'
Motivation:
Sometimes you need to quickly run a small snippet of TypeScript code to test functionality or debug a piece of logic. The --eval
option allows developers to directly evaluate TypeScript code from the command line, akin to a REPL (Read-Eval-Print Loop). This is particularly helpful for experimental purposes or when working within script confines without needing a dedicated .ts file.
Explanation:
--eval
: This option specifies that what follows is a TypeScript expression or series of expressions to be evaluated directly.'console.log("Hello World")'
: A simple TypeScript statement that logs “Hello World” to the console.
Example Output:
Hello World
Execute a TypeScript file in script mode:
Code:
ts-node --script-mode path/to/file.ts
Motivation:
Script mode is useful when you want to run a TypeScript file that behaves more like a script, focusing primarily on executing top-level code rather than exporting or importing modules. This mode is beneficial when writing small utility scripts or executable tasks.
Explanation:
--script-mode
: This flag runs the file as if it were a script, treating it differently from module execution.path/to/file.ts
: Specifies the file to be executed in script mode, enabling its use as a standalone script.
Example Output:
Script execution complete.
Transpile a TypeScript file to JavaScript without executing it:
Code:
ts-node --transpile-only path/to/file.ts
Motivation:
Sometimes you only need the transpiled JavaScript output from your TypeScript code without executing it. This is particularly useful for debugging, preparing precompiled files, or integrating with systems that collect JavaScript content as part of a build process. The --transpile-only
option provides this utility.
Explanation:
--transpile-only
: Specifies that the TypeScript file should be transpiled to JavaScript without running the resulting code.path/to/file.ts
: Indicates the TypeScript file to transpile, generating JavaScript output.
Example Output:
Transpiled 'path/to/file.ts' to JavaScript successfully.
Display TS-Node help:
Code:
ts-node --help
Motivation:
When working with a command-line tool, understanding the options available and how to use them can significantly improve workflow efficiency. Accessing the help documentation via --help
provides valuable information about different flags and configurations possible with ts-node
.
Explanation:
--help
: This flag invokes the help documentation forts-node
, detailing various options and usage guidelines.
Example Output:
Usage: ts-node [options] [ -e script | script.ts ] [arguments]
...
Options:
--help Show help
--version Show version number
--eval, -e [code] Evaluate script
...
Conclusion:
ts-node
simplifies the execution of TypeScript code by integrating compilation and execution into a single step. Whether you’re running entire TypeScript files, evaluating small snippets, or bypassing project configurations, ts-node
offers flexibility and efficiency for developers focused on TypeScript environments.