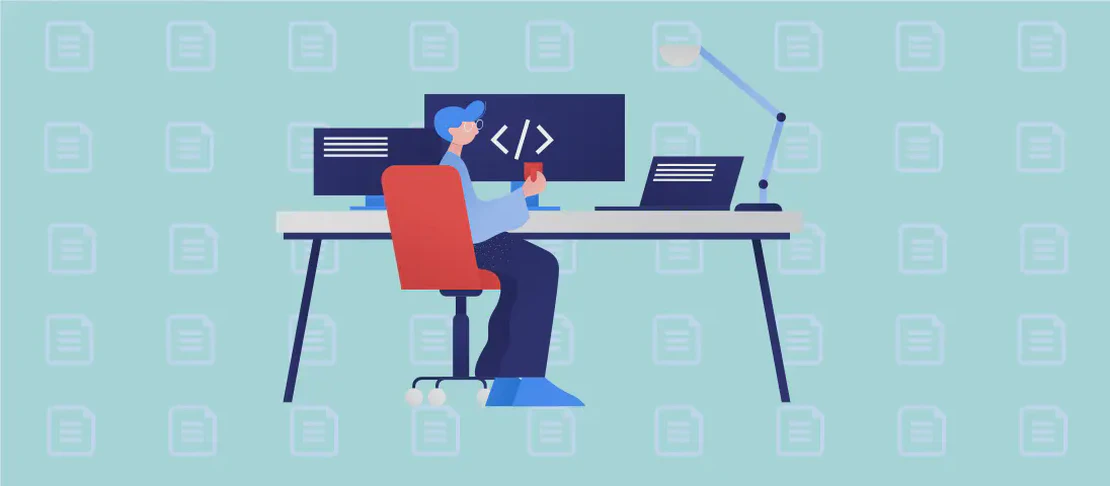
How to Use the Command 'tsc' (with Examples)
The tsc
command is the TypeScript compiler, a powerful tool that allows developers to compile TypeScript code into JavaScript, ensuring type safety and taking advantage of modern JavaScript features. TypeScript enhances JavaScript by adding strong typing, which can help catch errors during the development process rather than at runtime. The TypeScript compiler, tsc
, provides a wide range of options to customize the compilation process, making it a versatile tool for different projects.
Use case 1: Compile a TypeScript file into a JavaScript file
Code:
tsc foobar.ts
Motivation:
When you’re working with TypeScript, your code needs to be compiled into JavaScript to run it in most environments, such as browsers or Node.js. This basic command is the starting point for transforming your TypeScript code into a JavaScript file, allowing you to execute it with standard JavaScript engines.
Explanation:
tsc
: Invokes the TypeScript compiler.foobar.ts
: Specifies the TypeScript file you want to compile. The compiler takes this.ts
file and translates it into a.js
file with the same name,foobar.js
.
Example output:
- The command will generate a
foobar.js
file beside yourfoobar.ts
file. This.js
file represents the TypeScript code compiled into standard JavaScript.
Use case 2: Compile a TypeScript file with a specific target syntax
Code:
tsc --target ES5 foobar.ts
Motivation:
JavaScript runs on many different engines that might not support the latest ECMAScript features. Specifying a target ensures that your compiled code is compatible with the desired JavaScript version. This is crucial when maintaining compatibility with older browsers or environments.
Explanation:
--target ES5
: This option sets ECMAScript 5 as the target for the output JavaScript. Other possible values include ES2015, ES2016, ES2017, ES2018, and ESNEXT, each representing different levels of JavaScript syntax.foobar.ts
: The TypeScript file being compiled.
Example output:
- Outputs a
foobar.js
file with JavaScript syntax compatible with ECMAScript 5.
Use case 3: Compile a TypeScript file into a JavaScript file with a custom name
Code:
tsc --outFile output.js input.ts
Motivation:
Sometimes, project structure or naming conventions require that the output JavaScript file have a different name than the input TypeScript file. This use case allows for precise control over the output file name.
Explanation:
--outFile output.js
: Specifies the name of the output file. Instead of defaulting toinput.js
, the compiled file is namedoutput.js
.input.ts
: The input TypeScript file to be compiled.
Example output:
- The command will produce a single
output.js
file, streamlined with your project’s naming preferences.
Use case 4: Compile all .ts
files in a project as defined in a tsconfig.json
Code:
tsc --build tsconfig.json
Motivation:
Projects often consist of multiple TypeScript files and having a centralized configuration file, tsconfig.json
, allows for a more organized build process. This use case allows for the compilation of an entire project as per settings defined in the configuration file.
Explanation:
--build
: Tells the compiler to construct the entire project as specified intsconfig.json
.tsconfig.json
: The JSON configuration file that dictates how the TypeScript files in a project should be compiled, including options such as module system, target version, and more.
Example output:
- The command will process all TypeScript files defined within the
tsconfig.json
, resulting in JavaScript files as specified by your build configuration.
Use case 5: Run the compiler using command-line options from a text file
Code:
tsc @args.txt
Motivation:
This use case is useful for scenarios where numerous compiler options need to be specified. Instead of passing them all directly in the command line, they can be stored in a file, improving readability and maintenance.
Explanation:
@args.txt
: The compiler reads this text file containing a list of command-line options and arguments, with each on a new line, simplifying the build command.
Example output:
- The compiler will execute as if the commands were directly passed in the command line, outputting JavaScript files per the instructions specified in
args.txt
.
Use case 6: Type-check multiple JavaScript files and output only the errors
Code:
tsc --allowJs --checkJs --noEmit src/**/*.js
Motivation:
TypeScript’s capabilities extend to type-checking JavaScript files, providing benefits of static type checking without converting JS files into TS. This is invaluable for projects continually integrating JavaScript code.
Explanation:
--allowJs
: Enables TypeScript compiler to work with JavaScript files.--checkJs
: Activates type-checking for JavaScript files.--noEmit
: Ensures no output files are generated, the focus being strictly on error checking.src/**/*.js
: Specifies the directory and files to check, using glob patterns to match all JavaScript files within the specified folder structure.
Example output:
- The command will analyze JavaScript files, highlighting type errors in the console, but it won’t produce any compiled files.
Use case 7: Run the compiler in watch mode
Code:
tsc --watch
Motivation:
Watch mode is a dynamic way to compile TypeScript files. It automatically recompiles code when it detects changes, which is essential for development, allowing developers to see the effects of their changes in real-time.
Explanation:
--watch
: Engages the TypeScript compiler in watch mode, monitoring files for changes and recompiling whenever changes are detected.
Example output:
- This command won’t terminate after compilation. Instead, it keeps running, showing terminal logs of compile status and errors as files are modified.
Conclusion:
The tsc
command offers a robust set of options for compiling TypeScript code to JavaScript, catering to various needs such as compatibility and development efficiency. From compiling a single file to managing projects with multiple configurations, understanding these use cases empowers developers to leverage TypeScript’s capabilities fully.