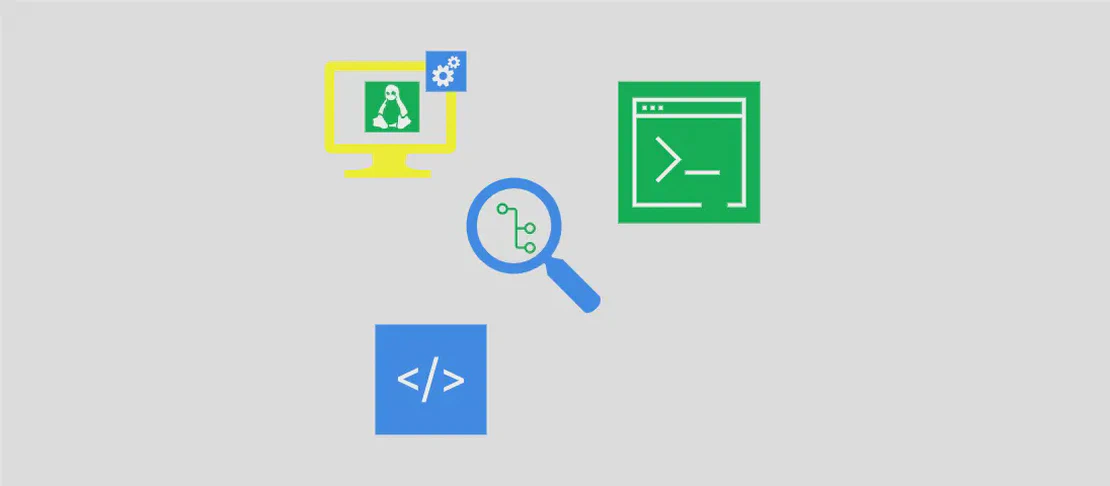
Comprehensive Guide to Using TSLint for TypeScript Projects (with examples)
TSLint is a powerful, pluggable linting tool specifically created for TypeScript, aimed at identifying and ensuring the adherence to consistent code style in TypeScript projects. By enforcing coding conventions, TSLint helps improve code readability, maintainability, and overall quality. This article explores various use cases of the TSLint command, providing examples and explanations for each scenario, empowering developers to effectively utilize this tool in their TypeScript workflows.
Use case 1: Create TSLint Config
Code:
tslint --init
Motivation:
Creating a TSLint configuration file is the fundamental first step for setting up linting in any TypeScript project. This command initializes a tslint.json
file, which serves as the linting configuration tailored to your specific project needs. The configuration file allows developers to set rules, extend existing rule sets, and define custom rules, aligning linting practices with individual project requirements.
Explanation:
--init
: This flag signals TSLint to generate a blueprint configuration file (tslint.json
) in your project’s root directory. The initialized file contains basic settings and can be modified to include additional rules or change configurations as your project evolves.
Example Output:
Upon executing the command, you may see output similar to the following:
Configuration file "tslint.json" created in the current directory.
This message confirms that the tslint.json
file has been successfully generated, which you can now edit to customize your linting rules.
Use case 2: Lint on a Given Set of Files
Code:
tslint path/to/file1.js path/to/file2.js ...
Motivation:
Specifying individual file paths when running TSLint is particularly useful when you want to perform linting on specific files rather than the entire project. This approach is beneficial during development, testing, or when you’re dealing with new files that are yet to be integrated into the project’s main build process.
Explanation:
path/to/file1.js
,path/to/file2.js
, etc.: Here, the arguments represent the paths to the specific files you want to lint. By listing the file paths explicitly, TSLint will analyze only those files, allowing for focused linting efforts on recent changes or newly added files.
Example Output:
Executing the above command might produce output like:
Linting: path/to/file1.js
WARNING: 1:1 semicolon Missing semicolon
ERROR: 10:23 no-console Calls to 'console.log' are not allowed.
Linting: path/to/file2.js
No lint errors found.
This output clearly indicates any detected violations, allowing developers to quickly address and resolve them.
Use case 3: Fix Lint Issues
Code:
tslint --fix
Motivation:
The ability to automatically fix certain linting issues is a time-saving feature offered by TSLint. This use case becomes relevant when you need to swiftly rectify code style issues without manually making changes to the codebase, enhancing developer efficiency and conforming code to agreed standards.
Explanation:
--fix
: This flag directs TSLint to automatically attempt to correct any fixable linting errors found. It optimizes the process of maintaining clean and compliant code by resolving straightforward discrepancies that do not require manual inspection.
Example Output:
After running this command, TSLint may output something like:
Fixed 10 errors in 5 files.
No remaining errors found.
This confirms that TSLint has addressed and corrected the detectable and fixable warnings or errors, thus refining your project’s code quality with minimal effort.
Use case 4: Lint with the Configuration File in the Project Root
Code:
tslint --project path/to/project_root
Motivation:
Linting an entire project using a configuration file located in the project’s root facilitates a comprehensive analysis of code quality across all files within the project. This approach is particularly essential for large teams or legacy systems, where ensuring consistent code style and quality is crucial across a multitude of files.
Explanation:
--project
: This flag instructs TSLint to apply the lint checks using the configuration file found at the specified path to your project root. Linting the entire project ensures that every aspect of the codebase adheres to predefined coding standards.path/to/project_root
: Denotes the file path leading to the root directory of your TypeScript project. The linting process will recursively evaluate all files within this directory as per the rules defined intslint.json
.
Example Output:
Upon running this command, possible output would look something like:
Linting project at path/to/project_root
All files passed linting.
This indicates successful execution, showing that all files within the project adhered to the defined linting rules without any major issues.
Conclusion:
TSLint offers a wide array of functionalities that cater to different stages of the software development lifecycle. Understanding and effectively using TSLint commands can significantly impact how you manage code quality and standards across diverse TypeScript projects. Through these use cases, developers are better equipped to initiate, target, fix, and comprehensively lint their TypeScript codebases, thereby fostering enhanced code consistency and quality maintenance.