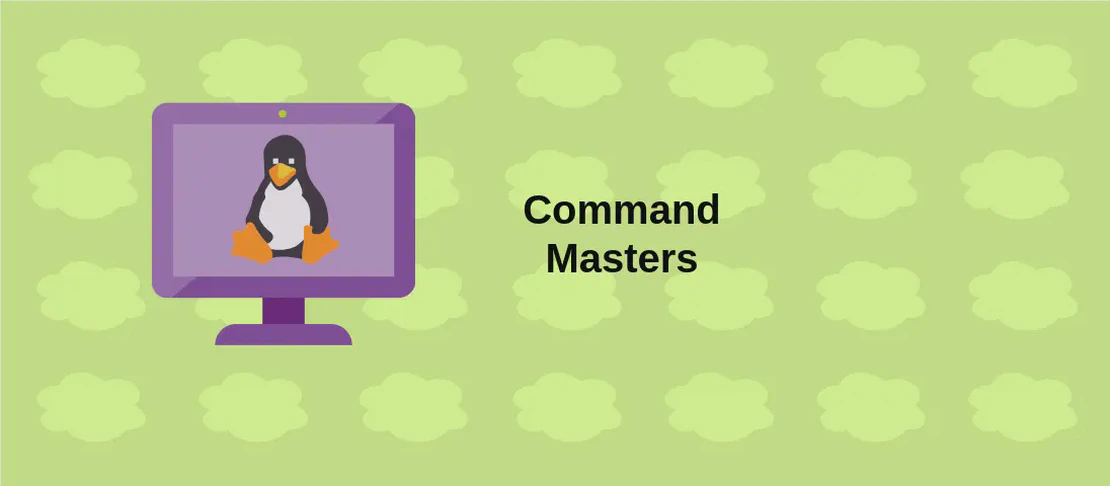
How to use the command 'twine' (with examples)
Twine is a command-line utility for publishing Python packages to the Python Package Index (PyPI), which is the official repository for Python software. Twine simplifies the process of uploading distributions, ensuring that your package metadata meets PyPI’s requirements, and supports uploading packages to alternate repositories such as TestPyPI. This utility emerges as an essential tool for Python developers aiming to distribute their packages widely or pre-test uploads to avoid surprises before a live roll-out.
Use case 1: Upload to PyPI
Code:
twine upload dist/*
Motivation: This is the fundamental command for any Python developer preparing to release their package to the world. Uploading to PyPI makes your package publicly available for installation by anyone using pip, thus enhancing its accessibility and encouraging community involvement or contributions.
Explanation:
twine upload
: This tells Twine to start the process of uploading files.dist/*
: Thedist
directory typically contains distribution files generated bysetup.py
, such as wheels or source archives. The asterisk (*
) indicates that all files within thedist
directory are to be uploaded.
Example output:
Uploading distributions to https://upload.pypi.org/legacy/
Uploading mypackage-0.1-py3-none-any.whl
100%|███████████████████████████████████████| 13.8k/13.8k [00:01<00:00, 24.4kB/s]
Use case 2: Upload to the Test PyPI repository to verify things look right
Code:
twine upload -r testpypi dist/*
Motivation: Testing packages on Test PyPI is an excellent way to ensure that everything looks good before an official release. This practice helps in identifying and resolving issues like metadata errors or dependency mismatches without affecting the main PyPI repository.
Explanation:
-r testpypi
: The-r
flag allows you to specify a particular repository from the.pypirc
configuration file. “testpypi” is a special alternative PyPI repository meant for testing uploads.dist/*
: Again indicates that all files in thedist
directory will be uploaded.
Example output:
Uploading distributions to https://test.pypi.org/legacy/
Uploading mypackage-0.1-py3-none-any.whl
100%|███████████████████████████████████████| 13.8k/13.8k [00:01<00:00, 24.2kB/s]
Use case 3: Upload to PyPI with a specified username and password
Code:
twine upload -u username -p password dist/*
Motivation: Security concerns often require package uploaders to utilize specific credentials rather than global access tokens or sessions when interacting directly with PyPI. This setup ensures that only authorized users publish to an account, restricting unauthorized modifications.
Explanation:
-u username
: Specifies the username for authentication with the PyPI server.-p password
: Specifies the password corresponding to the given username. It’s advised to consider using environment variables for increased security if possible.dist/*
: Denotes all files in the distribution folder for upload.
Example output:
Uploading distributions to https://upload.pypi.org/legacy/
Enter your password:
Uploading mypackage-0.1-py3-none-any.whl
100%|███████████████████████████████████████| 13.8k/13.8k [00:01<00:00, 24.4kB/s]
Use case 4: Upload to an alternative repository URL
Code:
twine upload --repository-url repository_url dist/*
Motivation: Organizations, especially those managing private or internal projects, often set up custom package repositories outside of the standard PyPI infrastructure. Uploading to an alternative URL allows these organizations to securely upload and access Python packages within a controlled environment.
Explanation:
--repository-url repository_url
: Sets a custom URL for the targeted repository, differing from the default PyPI or Test PyPI URLs.dist/*
: Indicates all distribution files targeted for upload.
Example output:
Uploading distributions to https://custom.pypi.repository.com/
Uploading mypackage-0.1-py3-none-any.whl
100%|███████████████████████████████████████| 13.6k/13.6k [00:00<00:00, 24.9kB/s]
Use case 5: Check that your distribution’s long description should render correctly on PyPI
Code:
twine check dist/*
Motivation: A well-rendered long description can be a deciding factor for users considering the installation of a package. Before uploading, it’s wise to ensure that your README and other descriptive elements render correctly in PyPI’s display by using this command.
Explanation:
check
: Initiates Twine’s check function, which verifies whether the long description will be appropriately rendered.dist/*
: Specifies the distribution files for the check process.
Example output:
Checking distribution dist/mypackage-0.1-py3-none-any.whl: Passed
Use case 6: Upload using a specific pypirc configuration file
Code:
twine upload --config-file configuration_file dist/*
Motivation: Developers managing multiple projects or environments may have different configurations specified in separate .pypirc
files. This flexibility allows selecting the precise configuration for the current task at hand, streamlining the deployment pipeline.
Explanation:
--config-file configuration_file
: Directs Twine to use a specified.pypirc
configuration file instead of the default.dist/*
: Marks all files in the distribution folder for upload.
Example output:
Using configuration file: path/to/configuration_file
Uploading distributions to https://upload.pypi.org/legacy/
Uploading mypackage-0.1-py3-none-any.whl
100%|███████████████████████████████████████| 13.8k/13.8k [00:00<00:00, 26.7kB/s]
Use case 7: Continue uploading files if one already exists (only valid when uploading to PyPI)
Code:
twine upload --skip-existing dist/*
Motivation: During multiple uploads, a situation may arise where certain files already exist on PyPI, halting the upload process. The --skip-existing
option allows developers to continue uploading new files without disruptions caused by existing ones.
Explanation:
--skip-existing
: This flag tells Twine to ignore files already present on the server, proceeding with the upload of any new or updated files.dist/*
: Indicates the distribution files to be uploaded.
Example output:
Skipping existing file: mypackage-0.1-py3-none-any.whl
Uploading mypackage-0.2-py3-none-any.whl
100%|███████████████████████████████████████| 14.1k/14.1k [00:01<00:00, 20.0kB/s]
Use case 8: Upload to PyPI showing detailed information
Code:
twine upload --verbose dist/*
Motivation: For developers seeking a closer look at the upload process, the verbose option provides detailed feedback about the stages and operations involved. This insight can be invaluable in diagnosing issues or simply ensuring transparent operation.
Explanation:
--verbose
: Enables detailed logging output, showing each step Twine takes during the upload process, including API call details and responses.dist/*
: Designates all files within the distribution folder for upload.
Example output:
Uploading distributions to https://upload.pypi.org/legacy/
Uploading mypackage-0.1-py3-none-any.whl
Uploading baked-in-fingerprints... done
Starting new HTTPS connection (1): upload.pypi.org:443
https://upload.pypi.org:443 "POST /legacy/ HTTP/1.1" 200 136
100%|███████████████████████████████████████| 13.8k/13.8k [00:01<00:00, 23.5kB/s]
Upload successful
Conclusion:
Twine acts as an efficient, streamlined backend service for package distribution in Python. By examining these usage cases, Python developers can leverage Twine to simplify the deployment process, minimize errors, and effectively manage their package uploads. Whether publishing to PyPI or pre-testing on TestPyPI, Twine provides flexible and versatile command-line tools to ensure successful project releases.