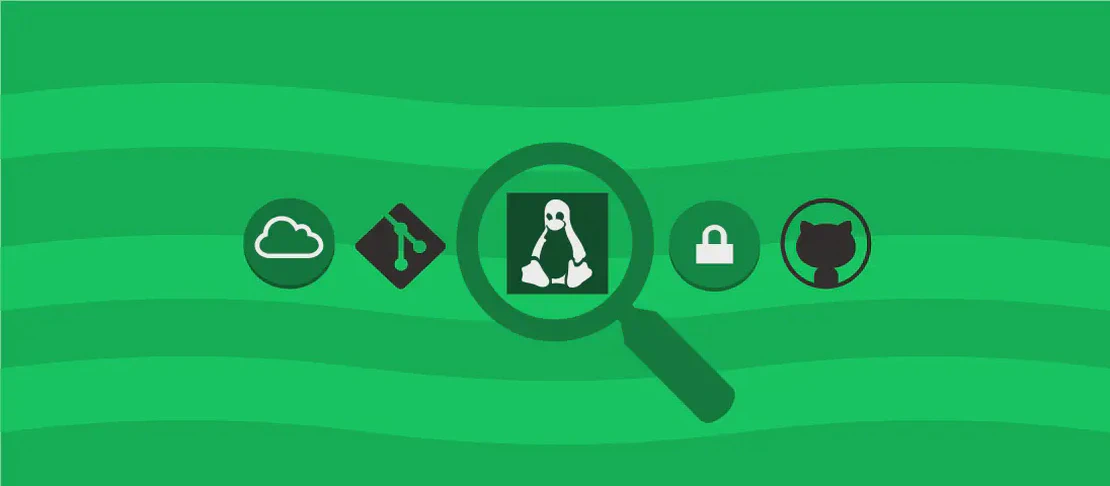
Harnessing TypeORM Features (with examples)
TypeORM is a powerful Object-Relational Mapping (ORM) tool for JavaScript, designed to work seamlessly across multiple platforms such as Node.js, browser, Cordova, Ionic, React Native, NativeScript, and Electron. It simplifies the interactions between applications and databases by mapping database schemas directly to JavaScript objects, thereby allowing developers to work with databases using familiar JavaScript concepts. Through TypeORM, tasks such as setting up a project, creating and managing migrations, and defining entities become more efficient and streamlined.
Generate a new initial TypeORM project structure
Code:
typeorm init
Motivation:
Starting a project with a solid foundation is crucial. The typeorm init
command provides an instant setup for a TypeORM project, ensuring that key components such as configuration files, directory structures, and essential dependencies are in place. This removes the typically tedious process of manual configuration, thus allowing developers to focus on application logic rather than boilerplate setup.
Explanation:
The typeorm init
command is designed to quickly scaffold out a new project directory structure consistent with TypeORM standards. It does not require any arguments, making it an accessible option for developers new to the TypeORM ecosystem.
Example output:
Project created.
Create an empty migration file
Code:
typeorm migration:create --name migration_name
Motivation:
Migrations are vital for tracking changes made to the database schema over time. By creating an empty migration file, developers ensure that they have a structured documentation of schema modifications. This practice is vital for collaboration, rollbacks, and deployments to ensure database consistency across various environments.
Explanation:
The typeorm migration:create
command is responsible for creating a new migration file. The --name
argument is used to specify the name of the migration, allowing the developer to describe the changes or fixes that the migration will encompass.
Example output:
Migration /path/to/project/migration_name.ts has been generated successfully.
Create a migration file with the SQL statements to update the schema
Code:
typeorm migration:generate --name migration_name
Motivation:
Automatically generated migration files ensure that your database schema remains in sync with your domain models. This shortcut minimizes manual intervention by using TypeORM’s introspection abilities to draft the SQL needed to bridge the current database state and the ORM definitions, reducing human error in SQL writing.
Explanation:
The typeorm migration:generate
command detects changes between the database schema and the current state of the entities in the codebase. The --name
argument is included to uniquely identify this specific set of changes.
Example output:
Migration /path/to/project/migration_name.ts has been generated successfully.
Run all pending migrations
Code:
typeorm migration:run
Motivation:
Running pending migrations is essential when progressing from one application state to another, particularly in development and production environments. It ensures that all necessary changes captured in migration files are applied to the database, providing the structure needed for the current iteration of the application.
Explanation:
The command typeorm migration:run
executes all migrations that have not yet been applied to the specified database connection. It doesn’t require specific arguments since it operates on all unapplied migrations.
Example output:
Running migrations:
Migration migration_name.ts has been executed successfully.
Create a new entity file in a specific directory
Code:
typeorm entity:create --name entity --dir path/to/directory
Motivation:
Entities are the core of TypeORM, representing database tables in the form of JavaScript classes. Having the ability to easily generate these entity files in a specific directory aids in maintaining a clean directory structure, fostering better organization and quicker access to these pivotal components of the application.
Explanation:
The typeorm entity:create
command is used to create a new entity file. The --name
argument specifies the name of the entity, while the --dir
argument determines the destination path within the project directory where the entity file will reside.
Example output:
Entity /path/to/directory/entity.ts has been created successfully.
Display the SQL statements to be executed by typeorm schema:sync
on the default connection
Code:
typeorm schema:log
Motivation:
Before changes are applied to a database, it is often helpful to preview the SQL statements that will be executed. This command provides transparency, allowing developers to review changes for confirmation and ensure that no unintended modifications are made to the database schema.
Explanation:
The typeorm schema:log
command elucidates the SQL statements that would be utilized to synchronize the database schema with the current state of the application’s entities. It acts as a safeguard by listing potential changes without executing them, thus allowing for a preliminary review.
Example output:
ALTER TABLE "user" ADD "age" integer;
ALTER TABLE "post" DROP COLUMN "title";
Execute a specific SQL statement on the default connection
Code:
typeorm query `SELECT * FROM users`
Motivation:
On-the-fly SQL execution is often handy for debugging and verifying the state of the database. Being able to directly run SQL commands bypasses the ORM’s abstraction layer and allows for precise interventions when diagnosing issues or making quick checks on data conditions.
Explanation:
The typeorm query
command allows the execution of a raw SQL statement. The sql_sentence
argument serves as a template for any specific SQL command the user wishes to run on the default database connection.
Example output:
id | name | age
---+-------+-----
1 | Alice | 28
2 | Bob | 34
Display help for a subcommand
Code:
typeorm migration:create --help
Motivation:
Detailed command insights are crucial for both beginners and advanced users alike. Knowing every flag available within a subcommand can optimize workflow processes, providing clarity and guidance on how to leverage TypeORM’s full potential.
Explanation:
The command typeorm subcommand --help
is a general syntax for gaining access to explicit documentation on any TypeORM subcommand. migration:create
in the example context, outlines the arguments and flags that can be paired with the migration creation utility, thus enhancing user understanding and capability.
Example output:
Usage: typeorm migration:create [options]
Options:
-n, --name <name> Name of the migration class
-d, --dir <dir> Directory where migration should be created
--outputJs Generates a migration file on the JS format
Conclusion:
TypeORM equips developers with a powerful set of commands that streamline the management of database interactions and schema evolution across multiple environments. From initializing projects and managing migrations to executing specific SQL and reviewing changes, it serves as a cornerstone in crafting robust and scalable applications. Deepening familiarity with these command options ensures a productive development experience, minimizing potential issues and maximizing efficiency.