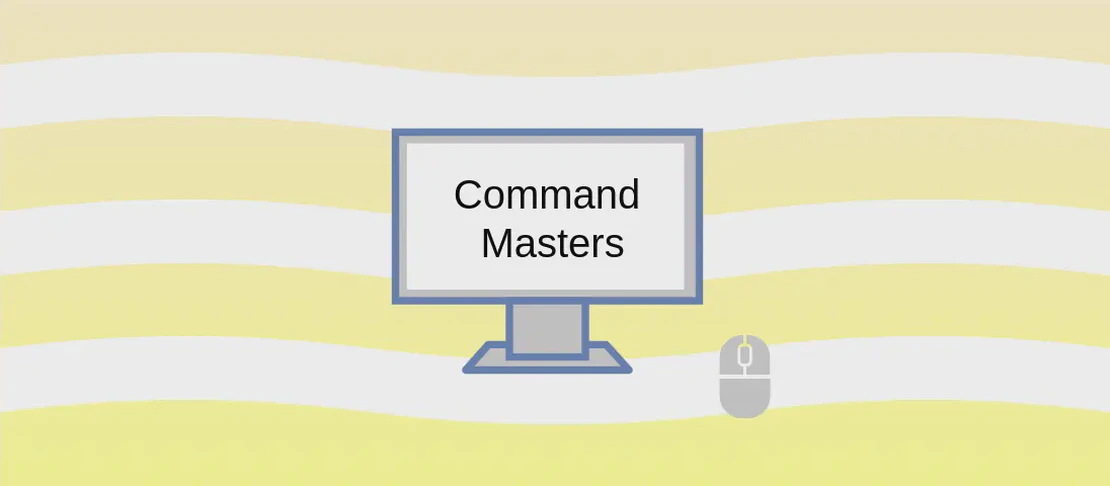
How to Use the Command 'typeset' (with Examples)
The typeset
command in the Bash shell is primarily used for declaring variables and setting their attributes. It’s a powerful tool that allows you to define how variables behave in your script, such as establishing a variable as an integer, an array, or even making it readonly. This command provides greater control over your variables, helping to ensure that your scripts are both robust and efficient. Understanding how to use typeset
can significantly improve the way you handle data within your Bash scripts.
Use Case 1: Declaring a String Variable
Code:
typeset variable="value"
Motivation:
Using typeset
to declare a string variable is a straightforward way to initialize a variable that will hold text data. This is one of the most common tasks in scripting, allowing you to store necessary information that can be used throughout your script. By using typeset
, you can also easily modify or reference this string variable later.
Explanation:
typeset
: The command used to declare a variable.variable
: The name of the variable being declared. This is essentially a placeholder where the data (in this case, a string) will be stored.="value"
: The specification that assigns a text value to the variable. Quotes are used to ensure that the value is read as a string, encompassing any spaces or special characters within.
Example Output:
After executing the code, the variable variable
holds the string value “value”. This can be referenced or altered as needed throughout the script.
Use Case 2: Declaring an Integer Variable
Code:
typeset -i variable="value"
Motivation:
When working with numbers that require arithmetic operations, declaring a variable as an integer ensures that the data is not inadvertently treated as a string. This prevents errors in calculations and maintains numerical accuracy.
Explanation:
typeset -i
: The-i
flag specifies that the variable should be treated as an integer. This ensures that any values assigned to the variable are interpreted as integers.variable
: The name of the integer variable.="value"
: The numeric value assigned to the variable. It will be stored and treated as an integer.
Example Output:
If you execute the code with typeset -i variable="42"
, the variable variable
will store the integer 42, ready for arithmetic operations.
Use Case 3: Declaring an Array Variable
Code:
typeset variable=(item_a item_b item_c)
Motivation:
Arrays are useful when dealing with collections of related data. By declaring an array, you can efficiently manage and process multiple values within a single variable, simplifying data manipulation and iteration.
Explanation:
typeset
: The command is used to declare variables, in this case, an array.variable
: The name representing the array.=(item_a item_b item_c)
: The list of items that the array holds. Each item is a distinct element in the array.
Example Output:
If you declare an array with typeset variable=(apple banana cherry)
, you produce an array where variable[0]
is “apple”, variable[1]
is “banana”, and variable[2]
is “cherry”.
Use Case 4: Declaring an Associative Array Variable
Code:
typeset -A variable=([key_a]=item_a [key_b]=item_b [key_c]=item_c)
Motivation:
Associative arrays are structured like a dictionary that maps keys to values, which is ideal when working with datasets that need explicit key-value pair associations. This can enhance readability and improve the management of complex data structures.
Explanation:
typeset -A
: The-A
flag indicates that the variable is an associative array.variable
: The name of the associative array.=([key_a]=item_a [key_b]=item_b [key_c]=item_c)
: Defines the key-value pairs within the associative array.
Example Output:
Executing the code will produce an associative array where variable[key_a]
is “item_a”, variable[key_b]
is “item_b”, and variable[key_c]
is “item_c”.
Use Case 5: Declaring a Readonly Variable
Code:
typeset -r variable="value"
Motivation:
Sometimes, it’s necessary to protect a variable from being altered after its initial assignment, especially when this variable stores a critical constant. Making a variable readonly ensures that its value remains unchanged throughout the script.
Explanation:
typeset -r
: The-r
flag designates the variable as readonly.variable
: The name of the unchangeable variable.="value"
: The initial and final value of the variable, which cannot be altered later.
Example Output:
The command typeset -r variable="constant"
will ensure that variable
holds “constant” and that any attempts to change it will result in an error.
Use Case 6: Declaring a Global Variable within a Function
Code:
typeset -g variable="value"
Motivation:
In complex scripts where functions are defined, it’s important to have global variables that can be accessed and modified outside a function’s scope. Declaring a global variable ensures its presence across the entire script.
Explanation:
typeset -g
: The-g
flag indicates that the variable should be treated as global.variable
: The name of the variable that will maintain its value across the script and across function calls.="value"
: The global value assigned to the variable.
Example Output:
If you declare a global variable with typeset -g variable="persistent"
, then no matter where you are in your script or which function you are inside, variable
will always reference the string “persistent”.
Conclusion:
The typeset
command in Bash is a versatile tool designed to control how variables are treated within your scripting environment. Whether you are dealing with simple strings, requiring numeric operations, handling arrays, or ensuring variable immutability, understanding the different options provided by typeset
helps you write cleaner, more efficient, and more reliable scripts. Knowing how to effectively declare and manage variable types is an essential skill for any scriptwriter aiming for code robustness and clarity.