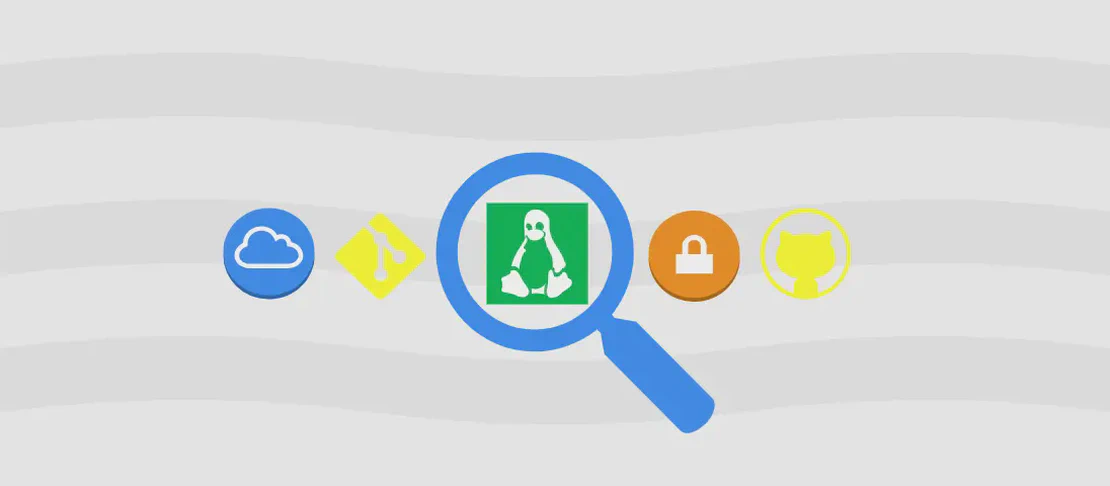
How to Use the Command 'usql' (with examples)
The usql
command-line utility is a powerful tool that provides a universal interface for interacting with SQL databases. Its versatility allows users to connect to various SQL database management systems such as PostgreSQL, MySQL, SQLite, SQL Server, and more with a unified command set. This makes usql
an excellent option for developers or database administrators who require cross-database management capabilities without needing to juggle different database-specific tools.
Use case 1: Connect to a specific database
Code:
usql sqlserver|mysql|postgres|sqlite3|...://username:password@host:port/database_name
Motivation:
Connecting to a specific database is often the first step a developer or database administrator takes before performing any operations. Whether you are pulling data for a report, updating records, or running maintenance tasks, establishing a connection allows you to authenticate and gain access to the data stored within the database. usql
simplifies this process by providing a uniform connection string format for multiple types of databases.
Explanation:
sqlserver|mysql|postgres|sqlite3|...
: This part of the command specifies the type of database you are connecting to. By providing a unified syntax,usql
allows you to choose the appropriate database driver.username:password
: Your credentials for logging into the database. Securely providing these ensures authenticated access.host
: The address where your database is hosted. This could be a local IP address or a network server.port
: The network port used by the database service. Different databases have default ports, which you may need to specify if they differ from the defaults.database_name
: The name of the specific database you wish to access within the server.
Example Output:
Upon executing the command, you will receive a prompt indicating a successful connection to the specified database, ready for further operations.
Use case 2: Execute commands from a file
Code:
usql --file=path/to/query.sql
Motivation:
Executing SQL commands from a file is extremely helpful when you have a batch of commands or a script that needs to be run consistently in different environments. This use case is efficient for executing setups, migrations, or routine tasks without manually inputting each command. It helps ensure consistency in SQL operations across environments.
Explanation:
--file=path/to/query.sql
: This argument pointsusql
to the specific file containing SQL commands you wish to execute. By reading from the file,usql
processes each command in sequence, providing an efficient way to perform batch operations.
Example Output:
The usql
utility will process each command in the specified file, providing output for each like an interactive session, indicating successful execution or any errors encountered.
Use case 3: Execute a specific SQL command
Code:
usql --command="sql_command"
Motivation:
In scenarios where you need to run a single, specific SQL command directly from the command line interface, using this feature can save time. For example, a quick query or a simple update without the need for a file can be performed rapidly using this option.
Explanation:
--command="sql_command"
: This flag allows you to pass a single SQL command directly as a string argument.usql
will execute the command on the active database connection.
Example Output:
Running this command will immediately execute the provided SQL statement, returning results if applicable, or simply confirming execution for operations such as updates or inserts.
Use case 4: Run an SQL command in the usql
prompt
Code:
prompt=> command
Motivation:
For database exploration or dynamic querying, running SQL commands directly from the usql
prompt provides real-time interaction with the data. This is especially helpful when exploring data, debugging, or crafting new queries iteratively.
Explanation:
prompt=>
: This symbol indicates that you are in theusql
interactive session or prompt.command
: The SQL command you want to execute, allowing for real-time database interaction.
Example Output:
The command will execute and provide immediate feedback or results in the usql
interactive session, which allows for further queries or operations without needing to reconnect.
Use case 5: Display the database schema
Code:
prompt=> \d
Motivation:
Understanding the structure of your database is crucial for effective database management and development tasks. Displaying the schema allows you to quickly gain insights into the tables, columns, and data types in your database, facilitating tasks like query writing, data modeling, and debugging.
Explanation:
\d
: This meta-command inusql
outputs the schema of the current database, listing tables and their structures, giving insights into the organization of data.
Example Output:
Executing \d
will list all tables within your current database, along with their columns and data types, providing a comprehensive view of your database schema.
Use case 6: Export query results to a specific file
Code:
prompt=> \g path/to/file_with_results
Motivation:
Exporting query results is essential when you need to analyze data outside the database environment, share query outputs with others, or generate reports. By redirecting the output to a file, you ensure that data can be archived, processed, or reviewed later without needing to run the same query repeatedly.
Explanation:
\g path/to/file_with_results
: The\g
meta-command allows you to direct the output of the most recent query to a specified file path, preserving the results outside of the current session.
Example Output:
After executing a query, using this command will save the results into the designated file, ensuring that you have a permanent record of the data extracted.
Use case 7: Import data from a CSV file into a specific table
Code:
prompt=> \copy path/to/data.csv table_name
Motivation:
Importing data from a CSV file into a table is a common and practical task for data integration and migration. This use case allows you to populate tables with new data without needing a specialized data loading tool, thereby making the transition of data between external sources and your database seamless.
Explanation:
\copy path/to/data.csv table_name
: The\copy
command facilitates the loading of data from a CSV file directly into a specified table. The path points to the CSV file, andtable_name
is the target destination for the data within your database.
Example Output:
The specified table will be populated with the data from the CSV file. During the process, you might see progress or completion messages signifying that the import was successful, and any issues such as data format mismatches will be reported.
Conclusion:
The usql
command-line tool is a versatile and efficient utility designed for those who work with multiple SQL database systems. Its universal interface, combined with the ability to perform essential database operations seamlessly across different SQL platforms, makes it an invaluable tool for developers and database administrators alike. Whether connecting to a database, executing queries, importing data, or exporting results, usql
helps streamline database management tasks across various systems.