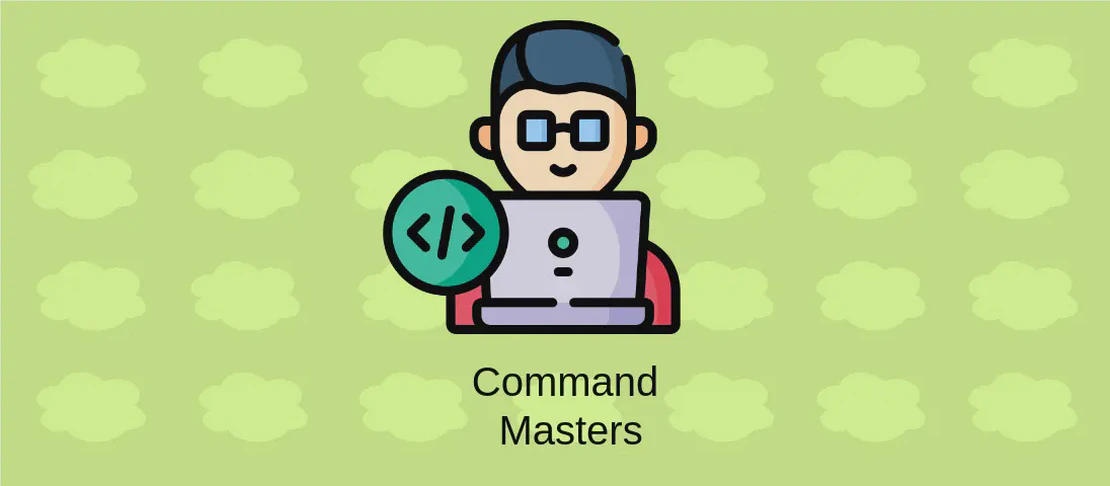
Using the `uuid` Command (with examples)
- Linux
- November 5, 2023
Use Case 1: Generate a UUIDv1
Code:
uuid
Motivation:
Generating a UUIDv1 can be useful when you need a unique identifier that incorporates a timestamp and the system’s hardware address (if available). This can be useful for ordering or tracking events or objects in a distributed system.
Explanation:
The uuid
command without any arguments generates a UUIDv1 based on the time and the system’s hardware address (if available).
Example Output:
7aef7fbc-7cbc-11eb-ae9f-8c859049d07f
Use Case 2: Generate a UUIDv4
Code:
uuid -v 4
Motivation:
Generating a UUIDv4 can be useful when you need a randomly generated unique identifier. This type of UUID is not based on any specific information and is suitable for cases where uniqueness is the main requirement.
Explanation:
The -v 4
option instructs the uuid
command to generate a UUIDv4 based on random data.
Example Output:
ecefb9d6-4698-4315-aa4a-4af46c0c90a2
Use Case 3: Generate multiple UUIDv4 identifiers at once
Code:
uuid -v 4 -n 3
Motivation:
Generating multiple UUIDv4 identifiers at once can be useful when you need a batch of unique identifiers for various purposes, such as generating unique filenames or database keys.
Explanation:
The -n
option specifies the number of UUIDs to generate, and the value after -n
indicates the desired quantity.
Example Output:
127d1b35-7cc8-4291-a7d5-0fdb616b9509
ef97371c-f94b-4f36-97ee-14dadaf97e45
163f397f-6a8f-4a9b-8e93-380cc20c68b7
Use Case 4: Generate a UUIDv4 and specify the output format
Code:
uuid -v 4 -F STR
Motivation:
Specifying the output format can be useful when you need the UUID generated by the uuid
command to be in a specific format to match the requirements of another system or library.
Explanation:
The -F
option followed by BIN
, STR
, or SIV
specifies the output format for the generated UUID. In this case, -F STR
is used to output the UUID as a string.
Example Output:
3e937aec-f1db-401a-9507-00e87e5be41c
Use Case 5: Generate a UUIDv4 and write the output to a file
Code:
uuid -v 4 -o path/to/file
Motivation:
Writing the output of the uuid
command to a file can be useful when you need to store the generated UUID for later use. This can be beneficial in scenarios where you want to generate unique identifiers and persist them in a file for future reference.
Explanation:
The -o
option followed by the file path specifies the location to save the generated UUID. In this case, path/to/file
represents the desired file path where the UUID will be written.
Example Output:
The UUID is written to the specified file (path/to/file
) as a string.
Use Case 6: Generate a UUIDv5 with a specified namespace prefix
Code:
uuid -v 5 ns:DNS object_name
Motivation:
Generating a UUIDv5 can be useful when you want to create a unique identifier based on a given object name and a specific namespace prefix. This can be helpful for scenarios where you need a deterministic UUID that persists across systems for the same object name and namespace.
Explanation:
The -v 5
option instructs the uuid
command to generate a UUIDv5. By using the -v 5 ns:DNS
argument, you can specify the namespace prefix for the UUID generation. The ns
is followed by the desired namespace prefix (DNS, URL, OID, or X500), and object_name
represents the object name associated with the UUID.
Example Output:
5cdfbdfa-27c8-589a-aa23-93fd74b8e964
Use Case 7: Decode a given UUID
Code:
uuid -d 7aef7fbc-7cbc-11eb-ae9f-8c859049d07f
Motivation:
Decoding a UUID can be useful when you have a UUID in its string format and want to retrieve the information encoded within it. This can be beneficial for analyzing and understanding the contents of a UUID.
Explanation:
The -d
option followed by the UUID string instructs the uuid
command to decode the provided UUID string and display the information encoded within it.
Example Output:
UUID: 7aef7fbc-7cbc-11eb-ae9f-8c859049d07f
Version: 1 (time-based version)
Variant: Reserved (NCS backward compatibility)
Timestamp: 1614130914
Clock ID: 55260
Node ID: 140508960944895
By exploring these various use cases, you can leverage the uuid
command to generate and decode.UUIDs for different purposes in your projects and systems.